Custom Splash Screen
Using this recipe you will replace the stock system UI splash screen.
The splash screen stays on the screen until all Frontend
s become ready; see
Frontend.isReady
for more details. It can be customized to show, for
example, a car brand logo or other visuals.
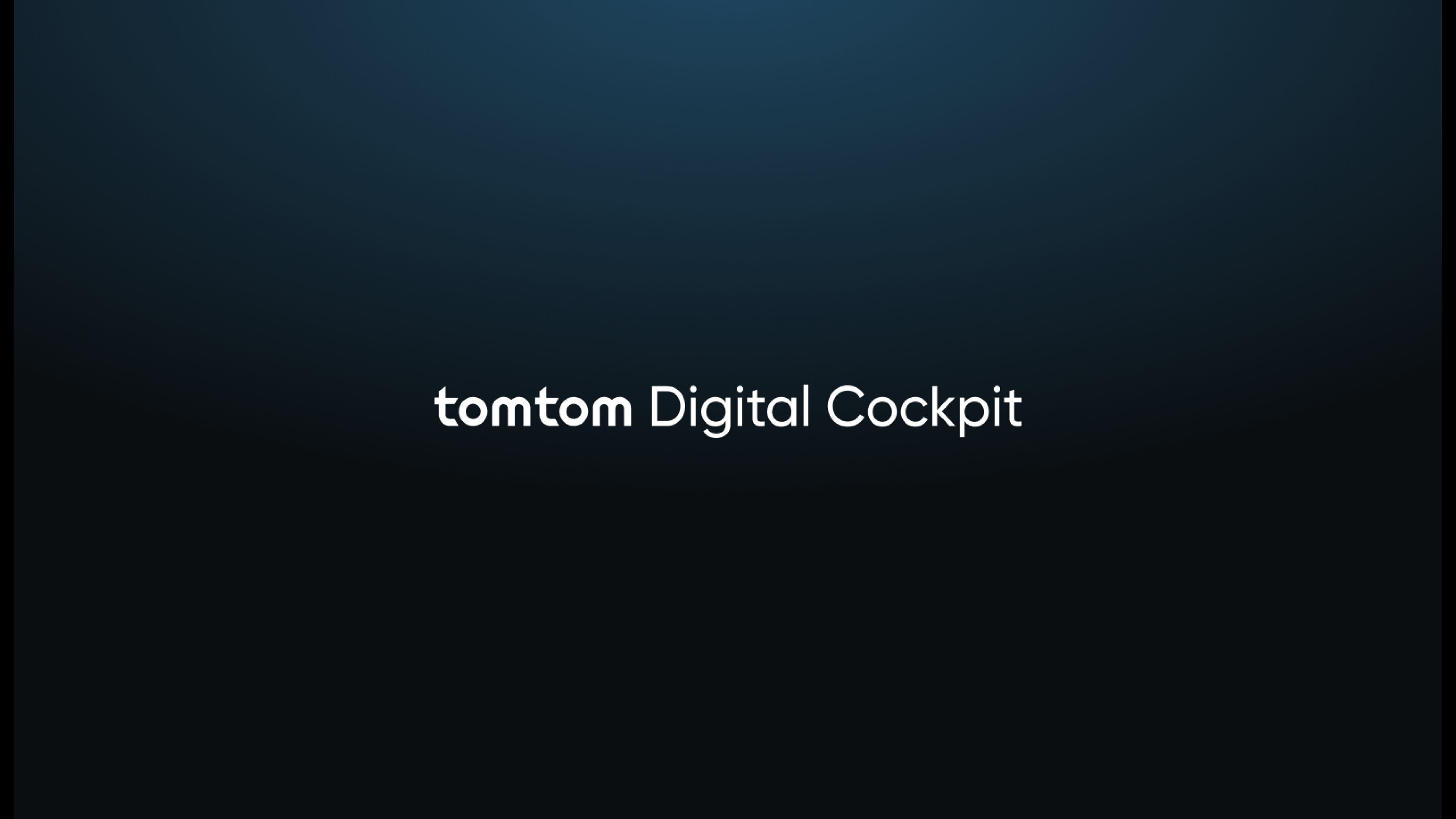
Out of the box
What the splash screen looks like by default.
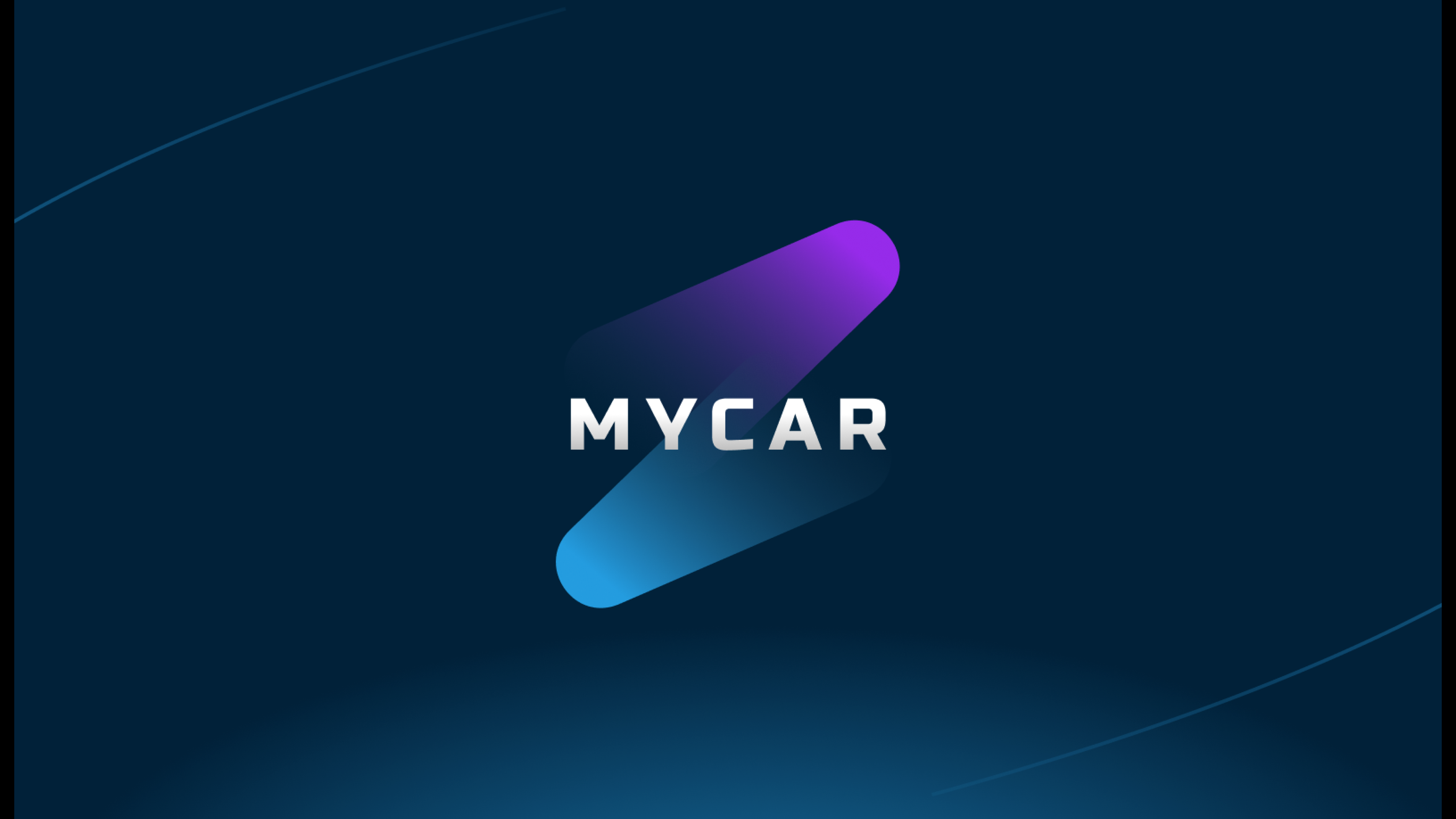
Replaced
The splash screen can be replaced with any custom Android view.
Setup
You will need an image to replace the stock splash screen image with. For the purpose of this guide you can use the one we provided here.
Creating a layout file for the custom splash screen
Create a
custom_splash_screen.xml
layout file.
1<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"2 android:layout_width="match_parent"3 android:layout_height="match_parent">45 <View6 android:layout_width="match_parent"7 android:layout_height="match_parent"8 android:background="@color/splash_screen_background_color" />910 <View11 android:layout_width="match_parent"12 android:layout_height="match_parent"13 android:background="@drawable/splash_screen_glow" />1415 <ImageView16 android:layout_width="@dimen/splash_screen_width"17 android:layout_height="@dimen/splash_screen_height"18 android:layout_gravity="center"19 android:src="@drawable/splash_screen_logo" />20</FrameLayout>
Creating a custom activity
Create a
SplashScreenActivity
.
Override the function getSplashScreenProvider()
to return a custom
SplashScreenProvider
that inflates the layout of the custom splash screen.
1class SplashScreenActivity : DefaultActivity() {23 override fun getSplashScreenProvider(): SplashScreenProvider {4 return object : SplashScreenProvider {5 override fun createSplashScreenView(splashScreenContainer: ViewGroup): View {6 return LayoutInflater7 .from(splashScreenContainer.context)8 .inflate(9 R.layout.custom_splash_screen,10 splashScreenContainer,11 false12 )13 }14 }15 }16}
Using the custom activity as a main activity in the manifest file
Add the new
SplashScreenActivity
to the
AndroidManifest.xml
file.
1<application>2 <activity3 android:name="com.tomtom.ivi.platform.framework.api.product.debugpermissions.SetupActivity">4 <meta-data5 android:name="com.tomtom.ivi.platform.debugpermissions.META_DATA_KEY_MAIN_ACTIVITY_CLASS_NAME"6 android:value="com.example.ivi.splashscreen.SplashScreenActivity"7 tools:replace="android:value" />8 </activity>910 <activity11 android:name="com.example.ivi.splashscreen.SplashScreenActivity"12 android:exported="false">13 </activity>14</application>
Build and deploy your app to your target device.
Next steps
Since you have learned how to create a custom splash screen, here are some recommendations for next steps:
Theming and Customization, for an overview of all theming and customization options.
Custom Fragments, to find out how to replace the Fragments inside the system UI panels.
Using the Configuration Framework, for more fine grained customization settings.