Using style in the application
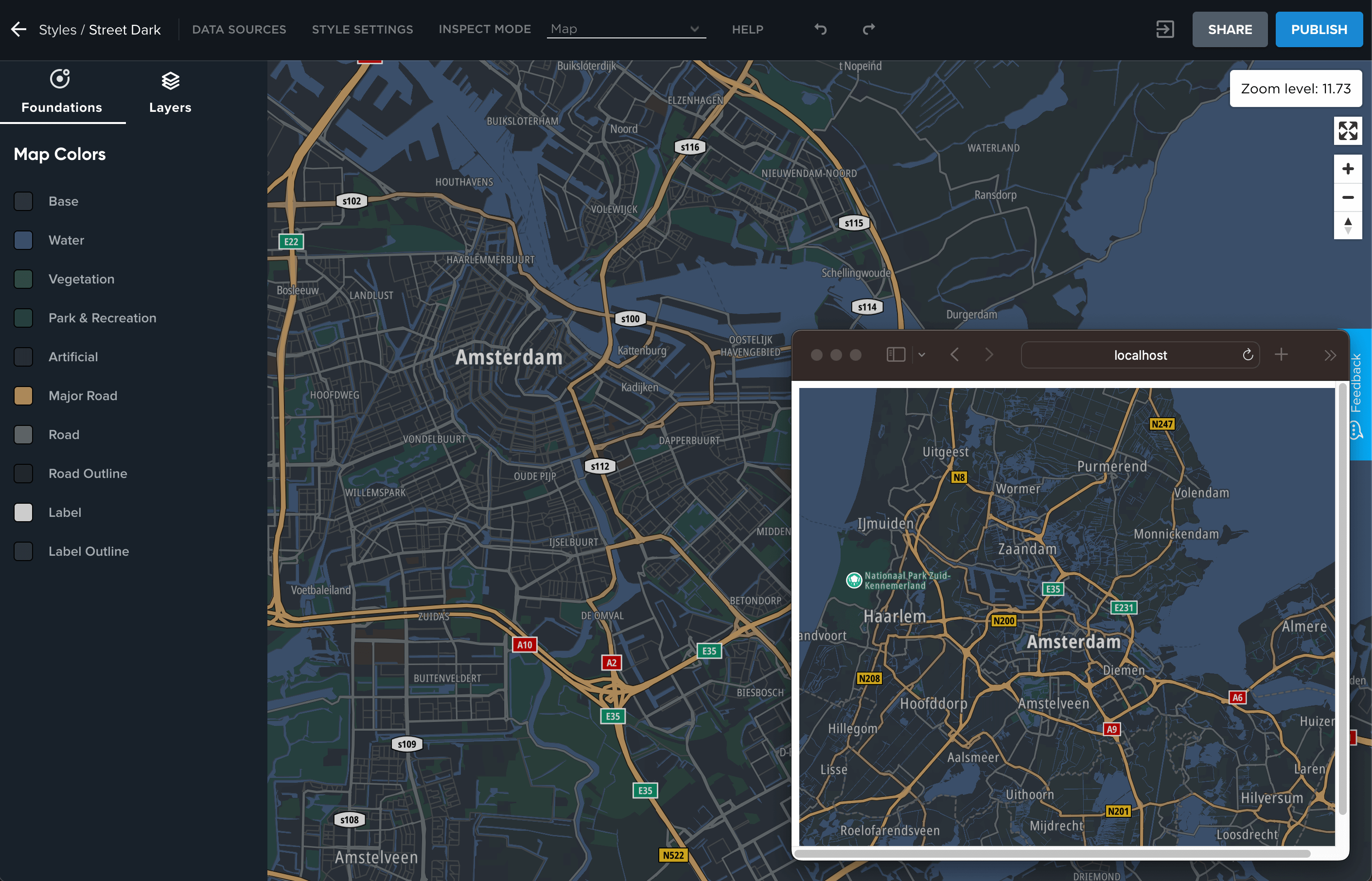
In this guide, you will learn how to use the Map Maker style in your application. The guide will explain how to use:
- style URL
- style file
This guide assumes you:
- Have read the Publish and Share style guide.
- Have an API Key from Dev Portal. Read How do I get a TomTom API Key? for help.
If you want to learn how to use custom style in the Android or iOS Maps SDK, read the Publish and Share style guide first, and then see the guides for Android or iOS.
Example application
An example web application in this guide is using MapLibre GL JS library version 3.0.1 to render the map. To focus on map styling and for simplicity, the application uses code snippets from the MapLibre GL JS API documentation. Copy and paste the following code snippet to your favorite code editor.
1<!DOCTYPE html>2<html lang="en">3 <head>4 <meta charset="UTF-8" />5 <meta http-equiv="X-UA-Compatible" content="IE=edge" />6 <meta name="viewport" content="width=device-width, initial-scale=1.0" />7 <title>My application</title>8 <script src="https://unpkg.com/maplibre-gl@3.0.1/dist/maplibre-gl.js"></script>9 <link10 href="https://unpkg.com/maplibre-gl@3.0.1/dist/maplibre-gl.css"11 rel="stylesheet"12 />13 </head>14 <body>15 <div id="map" style='width: 100vw; height: 100vh;'></div>16 <script>17 const map = new maplibregl.Map({18 container: "map",19 style: "https://demotiles.maplibre.org/style.json", // stylesheet location20 center: [4.89707, 52.377956], // starting position [lng, lat]21 zoom: 5, // starting zoom22 });23 </script>24 </body>25</html>
Note that:
- the
container
parameter of themaplibregl.Map
constructor requires the same value as the id of an HTML element containing the map. - the
style
parameter currently points to the example style provided by MapLibre.
Run an HTTP server
Run an HTTP Server in your code editor; it is needed to avoid CORS errors. You can use http-server
from npm
or popular extensions like Live Server
for VS Code users.
Open your browser and type the URL on which your HTTP Server is running.
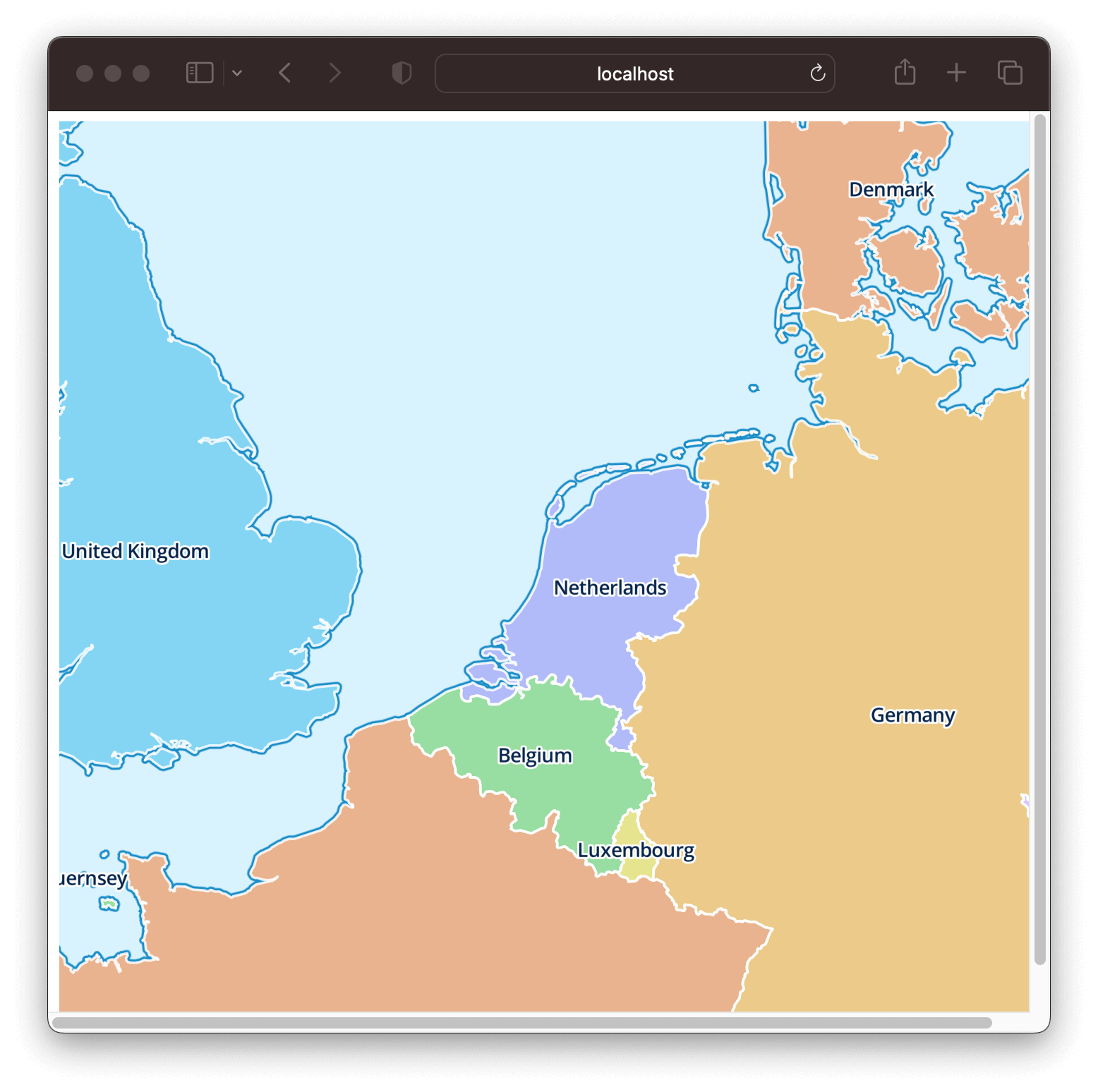
Using a style URL
Map Maker stores your map styles in the TomTom cloud. Your application can access them using style URL. You need to provide the URL only once; all the changes to the map style will be automatically updated under the same style URL.
Follow these steps to use the map style URL in your application:
-
Copy the style URL from the Map Maker, as described in the Publish and Share style guide. This guide assumes you have selected your API Key in Map Maker when sharing the style.
-
In the example application, substitute the stylesheet location with your own style: in the JavaScript section, replace the
style
parameter of themaplibregl.Map
constructor with the style URL copied from the Map Maker. This section should look like this now:1 const map = new maplibregl.Map({2 container: "map",3 style:4 "https://api.tomtom.com/style/2/custom/style/<YOUR-STYLE-ID>=.json?key=<YOUR-API-KEY>", // stylesheet location5 center: [4.89707, 52.377956], // starting position [lng, lat]6 zoom: 9, // starting zoom7 });Notice that in this code snippet, the API Key was replaced with the placeholder.
If you select API Key while sharing the style, the style URL and the style file will contain it. Remember to always protect your API Key. Never share it with others. If you plan to use it on the Web, enable domain whitelisting in the Edit key > Security, in the dashboard on the Developer Portal.
-
Your application is now using the Map Maker style URL.
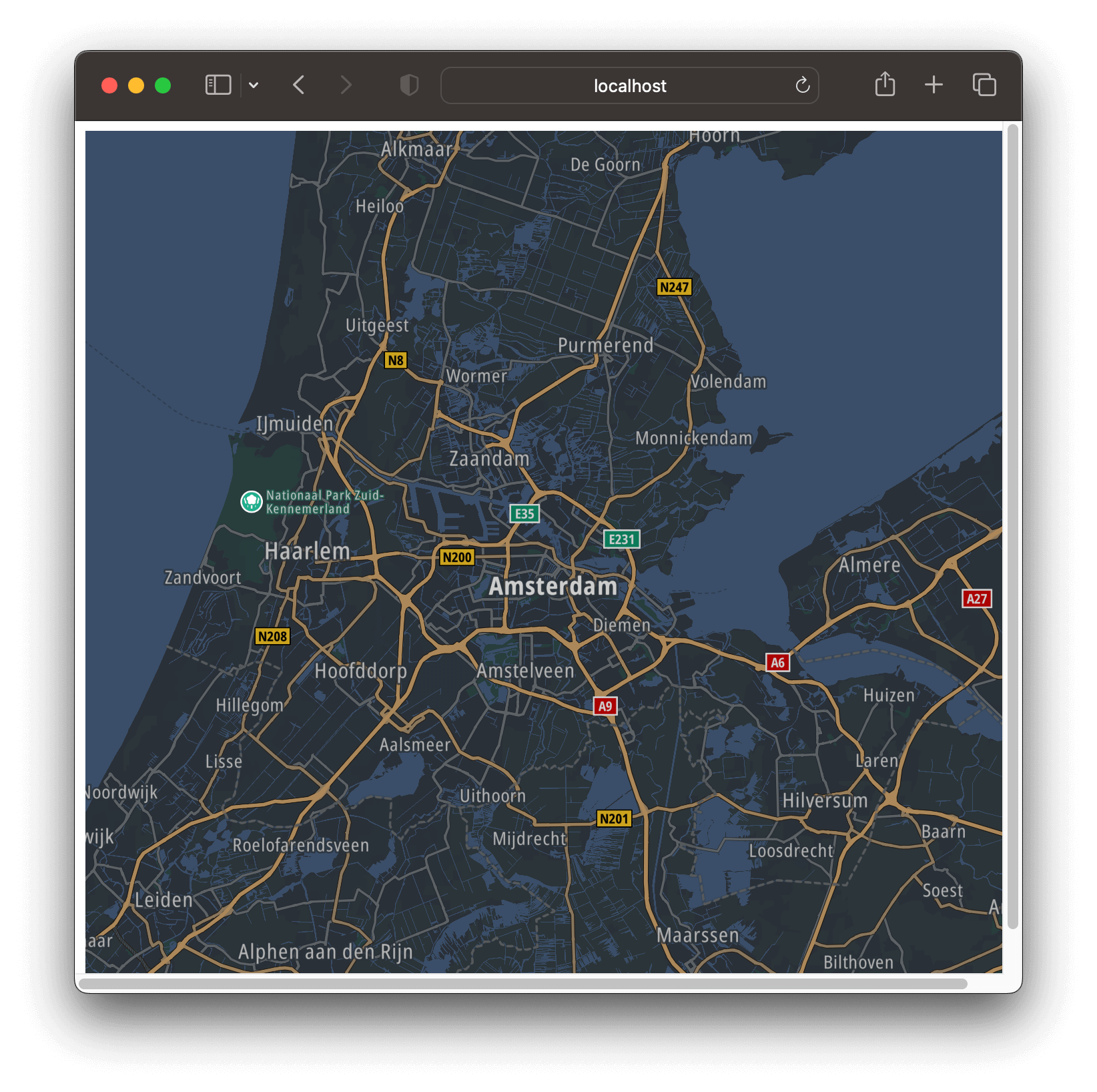
You can now make more changes to the style in the Map Maker. Map style in your application will update:
- after each change, if you are using the Draft style
- after you publish the changes, if you are using the Production style
Using a style file
You can download a style file in .json
format from Map Maker and use it in your application.
Follow these steps to use the map style file in your application:
-
Download the style URL from the Map Maker, as described in Publish and Share style guide. This guide assumes you have selected your API Key in Map Maker when sharing the style.
-
Place the style file in your application directory.
-
In the example application, substitute the stylesheet location with your own style: in the JavaScript section, replace the
style
parameter of themaplibregl.Map
constructor with the relative path to the style file from the Map Maker. If you placed the style file in your application directory, this section should look like this now:1 const map = new maplibregl.Map({2 container: "map",3 style:4 style: "my_custom_style.json", // stylesheet location5 center: [4.89707, 52.377956], // starting position [lng, lat]6 zoom: 9, // starting zoom7 }); -
Your application is now using the Map Maker style file.
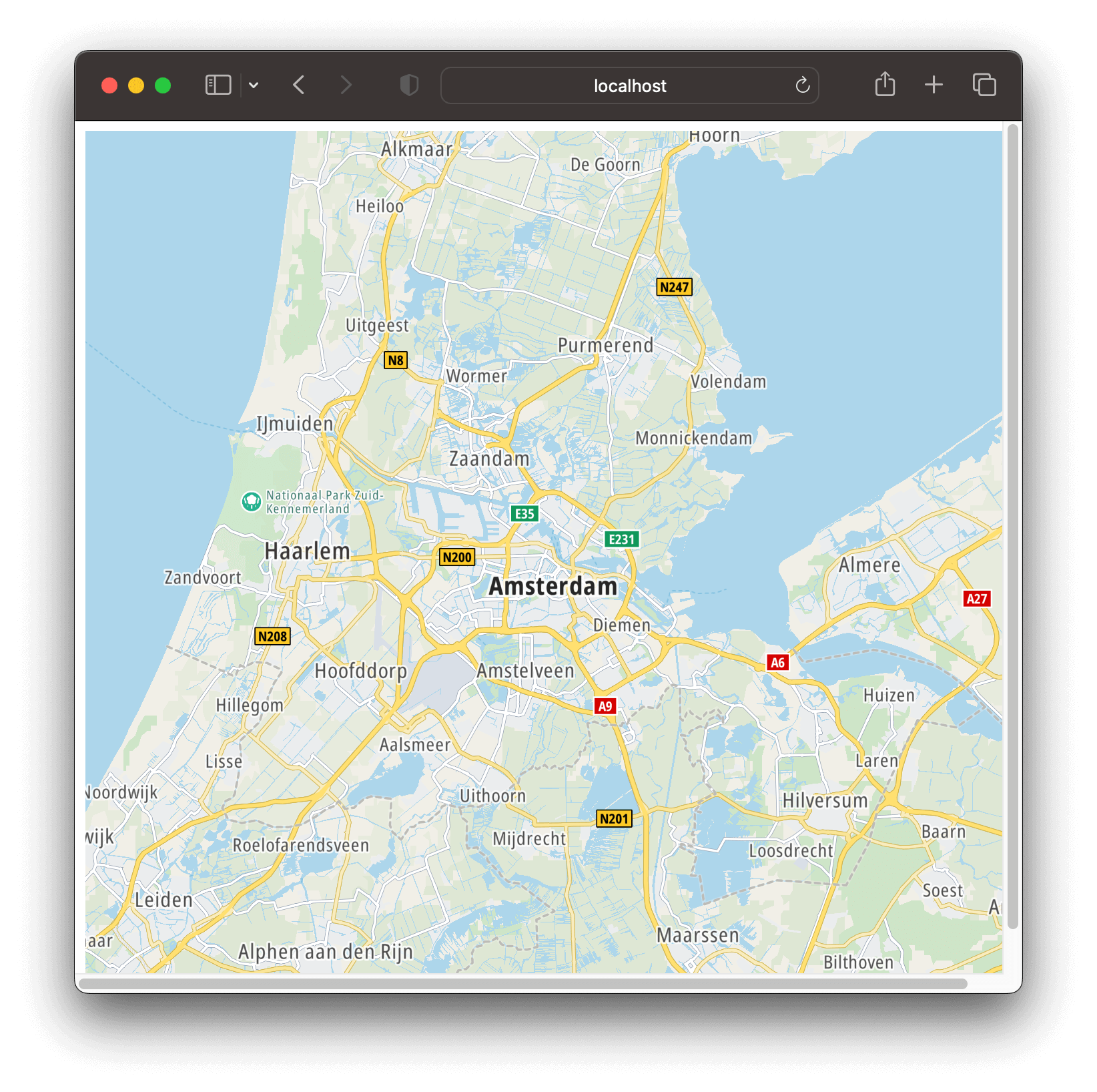
Keep in mind that if you make more changes to your style in the Map Maker, you will have to download the style file and replace it in your application again.
Since you have learned how to use the style in your application, here are recommendations for the next steps: