Tile Content
Important notes:
- This TomTom Orbis API is in public preview.
- This API is powered by TomTom Orbis Maps.
- See the TomTom Orbis Maps documentation for more information.
TomTom Vector Format
The TomTom Orbis Maps Vector Tiles Content document contains map features (points, lines, road shapes, water polygons, building footprints, etc.) that can be used to visualize map data. The data is divided into multiple protobuf layers. Besides the layers, the protobuf tags are also used to further describe the geographic features.
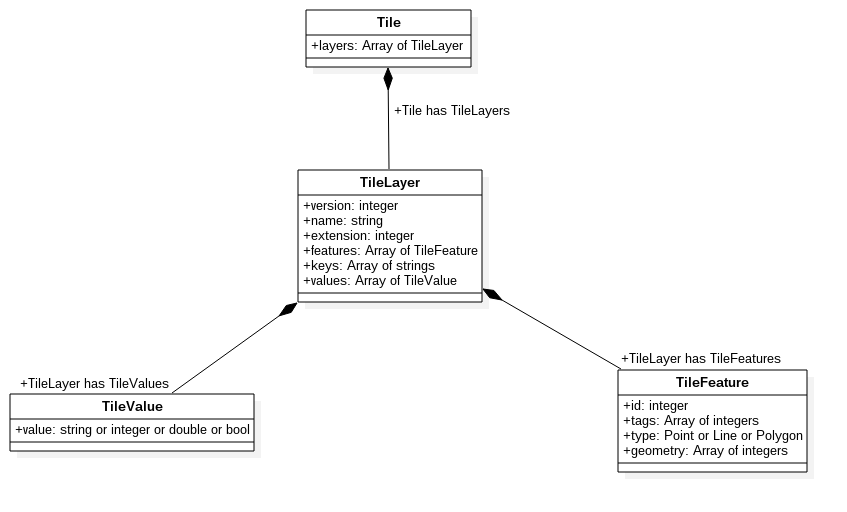
Legend
Tile
Represents a vector tile as a whole entity. It consists of at least one
TileLayer
. If the tile does not contain any relevant data, it contains one layer namedempty
.TileLayer
Part of a
Tile
, representing one of the layers that can be rendered later. One layer consists of e.g., all Parks, Roads, Built-up areas, or Points of interest.Each
TileLayer
contains geometries of the same type. A geometry in the vector tile can be one of three types:POINT
,LINESTRING
, orPOLYGON
. EveryTileLayer
contains the following fields:name
version
extent
It may also contain the following fields:
keys
is an array of uniquestrings
.values
is an array of uniqueTileValues
.features
is an array of uniqueTileFeatures
.
Arrays of
keys
andvalues
contain the mapping for tags ofTileFeature.
TileFeature
Member of an array of
features
stored in aTileLayer
, containing a geometry representation that can be rendered.It always contains the following fields:
type
is a type of geometry. It can bePOINT
,LINESTRING
, orPOLYGON
.geometry
is an array of encoded geometry that can be used for rendering. See the Decoding tile geometry section for details.
It may also contain the following fields:
tags
is an array containing properties of feature.They are a
TileFeature's
detailed description which can be used for styling and later rendering the geometry.- They are encoded into an array of integers.
In order to decode them, the arrays of
keys
andvalues
from a correspondingTileLayer
must be used.See the Decoding feature tile tags section for details.
TileValue
Encoded representation of a string, integer, floating point, or boolean value.
It is stored in a
values
array, in aTileLayer
.It is used for decoding
tags
of aTileFeature
(together with the members of akeys
array).See the Decoding feature tile tags section for details.
TomTom Vector Schema
Schema data
Formally, the structure of a vector tile is described by the protocol buffer schema. It allows the generation of C++, Java, Python, Go, Ruby, Objective-C, and C# code based on the proto file.
1package vector_tile23option optimize_for = LITE_RUNTIME;45message Tile {67 enum GeomType {8 UNKNOWN = 0;9 POINT = 1;10 LINESTRING = 2;11 POLYGON = 3;12 }1314 message Value {15 optional string string_value = 1;16 optional float float_value = 2;17 optional double double_value = 3;18 optional int64 int_value = 4;19 optional uint64 uint_value = 5;20 optional sint64 sint_value = 6;21 optional bool bool_value = 7;22 extensions 8 to max;23 }2425 message Feature {26 optional uint64 id = 1 [ default = 0 ];27 repeated uint32 tags = 2 [ packed = true ];28 optional GeomType type = 3 [ default = UNKNOWN ];29 repeated uint32 geometry = 4 [ packed = true ];30 optional bytes raster = 5;31 }3233 message Layer {34 required uint32 version = 15 [ default = 1 ];35 required string name = 1;36 repeated Feature features = 2;37 repeated string keys = 3;38 repeated Value values = 4;39 optional uint32 extent = 5 [ default = 4096 ];4041 extensions 16 to max;42 }4344 repeated Layer layers = 3;4546 extensions 16 to 8191;47}
Decoding tile geometry
Vector Tile geometry uses the following coordinate system:
- Coordinates are always integers.
- The
(0,0)
point is located in the upper-left corner of the tile. - The X axis has values increasing towards the right of the tile.
- The Y axis has values increasing towards the bottom of the tile.
- The tile may have margin, which is a buffer around the tile in the shape of a square frame. The size of the margin is by default equal to 10% of the width/length of the tile.
- The extent is equal to
4096
, so the value range for X and Y coordinates is from0
to4095
for visible points. - If the tile has a margin, the coordinates values range is extended by its size in both directions. This may cause coordinate values for points in the left or upper margin to be negative.
Vector Tile geometry is encoded as an array of 32 bit unsigned integers in the geometry
array field of the TileFeature
.
Encoded format has the following structure:
[command_and_count][x0][y0]..[xn][yn][command_and_count][x0][y0]..
command_and_count
contains encoded values ofcommand
andcount
.[x0][y0]..[xn][yn]
are(x,y)
coordinate pairs.
Command
The value of command
is decoded as follows:
command = command_and_count & 0x7
Commands are to be executed relative to previous path coordinates or the point (0,0)
if it is the first command.
Command type | Value | Coordinates | Description |
---|---|---|---|
MoveTo |
|
| It defines the
|
LineTo |
|
| It defines a new segment, starting at the current position of the cursor
and ending at the
|
ClosePath |
| none | It closes the current linear ring of a |
Count
The value of count
is decoded as follows:
count = command_and_count >> 0x3
- It defines the
n
number of[xn][yn]
encoded coordinate pairs following thecommand_and_count
value. - These coordinate pairs must be interpreted according to the preceding
command
type.
Coordinates
Coordinates [x0][y0]..[xn][yn]
are encoded in zigzag encoding and are relative to the previous coordinate pair. This means that only the first coordinate pair [x0][y0]
in the first command in every TileFeature
stores absolute values.
The coordinates are decoded as follows:
1decode(x0) = ((x0 >> 0x1) ^ (-(x0 & 0x1)))2decode(y0) = ((y0 >> 0x1) ^ (-(y0 & 0x1)))34decode(x1) = decode(x0) + ((x1 >> 0x1) ^ (-(x1 & 0x1)))5decode(y1) = decode(y0) + ((y1 >> 0x1) ^ (-(y1 & 0x1)))67...89decode(xn) = decode(xn-1) + ((xn >> 0x1) ^ (-(xn & 0x1)))10decode(yn) = decode(yn-1) + ((yn >> 0x1) ^ (-(yn & 0x1)))
Examples
For a POINT
type feature:
Input:
1layer: 02 feature: 03 type: POINT4 geometry: [9, 1136, 6564]
Decoding:
1geometry:2 command_and_count = 93 command = 9 & 0x7 = 14 count = 9 >> 0x3 = 156 x= 1136, y0 = 65647 decode(x0) = ((1136 >> 0x1) ^ (-(1136 & 0x1))) = 5688 decode(y0) = ((6564 >> 0x1) ^ (-(6564 & 0x1))) = 3282
Output:
1layer: 02 feature: 03 geometry: POINT(561, 3282)
For a LINESTRING
type feature:
Input:
1layer: 02 feature: 03 type: LINESTRING4 geometry: [9, 846, 2312, 10, 652, 1938]
Decoding:
1geometry:2 1:3 command_and_count = 94 command = 9 & 0x7 = 15 count = 9 >> 0x3 = 167 x0' = 846, y0' = 23128 decode(x0') = ((846 >> 0x1) ^ (-(846 & 0x1))) = 4239 decode(y0') = ((2312 >> 0x1) ^ (-(2312 & 0x1))) = 115610 2:11 command_and_count = 1012 command = 10 & 0x7 = 213 count = 10 >> 0x3 = 11415 x0 = 652, y0 = 193816 decode(x0) = decode(x0') + ((x0 >> 0x1) ^ (-(x0 & 0x1)))17 decode(y0) = decode(y0') + ((y0 >> 0x1) ^ (-(y0 & 0x1)))18 decode(x0) = 423 + ((652 >> 0x1) ^ (-(652 & 0x1))) = 74919 decode(y0) = 1156 + ((1938 >> 0x1) ^ (-(1938 & 0x1))) = 2125
Output:
1layer: 02 feature: 03 geometry: LINESTRING[(423, 1156), (749, 2125)]
For a POLYGON
type feature:
Input:
1layer: 02 feature: 03 type: POLYGON4 geometry: [9, 1320, 5622, 26, 416, 707, 68, 612, 483, 96, 7]
Decoding:
1geometry:2 1:3 command_and_count = 94 command = 9 & 0x7 = 15 count = 9 >> 0x3 = 167 x0' = 1320, y0' = 56228 decode(x0') = ((1320 >> 0x1) ^ (-(1320 & 0x1))) = 6609 decode(y0') = ((5622 >> 0x1) ^ (-(5622 & 0x1))) = 281110 2:11 command_and_count = 2612 command = 26 & 0x7 = 213 count = 26 >> 0x3 = 31415 x0 = 1736, y0 = 491416 decode(x0) = decode(x0') + ((416 >> 0x1) ^ (-(416 & 0x1))) = 660 + 208 = 86817 decode(y0) = decode(y0') + ((707 >> 0x1) ^ (-(707 & 0x1))) = 2811 + (-354) = 24571819 x1 = 68, y1 = 61220 decode(x1) = decode(x0) + ((68 >> 0x1) ^ (-(68 & 0x1))) = 90221 decode(y1) = decode(y0) + ((612 >> 0x1) ^ (-(612 & 0x1))) = 27632223 x2 = 483, y2 = 9624 decode(x2) = decode(x1) + ((483 >> 0x1) ^ (-(483 & 0x1))) = 66025 decode(y2) = decode(y1) + ((96 >> 0x1) ^ (-(96 & 0x1))) = 281126 3:27 command_and_count = 728 command = 7 & 0x7 = 729 count = 7 >> 0x3 = 0
Output:
1layer: 02 feature: 03 geometry: POLYGON[(660, 2811), (868, 2457), (902, 2763), (660, 2811)]
Decoding feature tags
In order to reduce tile size, the properties of each feature are encoded in a tags
array.
- The
tags
array contains integer values which are indexes ofkeys
andvalues
arrays belonging to the corresponding layer. - The size of a
tags
array is always even. - The content of a
tags
array can be grouped into a list of pairs. Each odd element of an array is the first element of a pair, and each even element of an array is the second element of a pair. - The first element of each pair should be mapped to a
keys
array. - The second element of each pair should be mapped to a
values
array. - As a result, we get a decoded list of
tags
where the first element is the tag name and the second element is the tag value in the form ofTileValue
.
Example
Below is an example of what an encoded tile may look like.
1layer: 02 keys: ["country_code", "icon_text"],3 values: ["SWE", "E4"]4 feature: 05 tags : [0,0,1,1]
After decoding it has to be read like this:
1layer: 02 feature: 03 properties:4 country_code : "SWE"5 icon_text : "E4"
Tile Layers
The data in TomTom Vector Tiles is organized in layers. The following section provides detailed information about:
- Available layers.
- Tags that typically appear in features from a given layer.
earth_cover
Earth cover refers to the generalized surface cover of the earth, whether vegetation, sand, ice, or other.
Geometry type: POLYGON
earth_cover tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
land_cover
Land cover refers to the surface cover on the ground, whether vegetation, urban infrastructure, or other; it does not describe the use of land, and the use of land may be different for lands with the same cover type. For instance, a land cover type of forest may be used for timber production, wildlife management, or recreation; it might be private land, a protected watershed, or a popular state park.
Geometry type: POLYGON
land_cover tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between land covers in the same category.
Values for category
Values for category
Values for category
|
| Fine-grain attribution of each land cover feature corresponding to the
most relevant value found among different feature tags (natural, landuse,
boundary, etc...) for more precise filtering or custom styling.
|
| A numerical value attribute that enables ordering and filtering based on
the ranking of the area. The values are in the range 1, 16 inclusive. |
| An attribute depicting whether a feature belongs to a wetland, to enable
pattern representation as additional overlay on standard fill symbology. |
land_use
The land use refers to the purpose the land serves, for example, recreation, wildlife habitat or agriculture; it does not describe the surface cover on the ground. For example, a recreational land use could occur in a forest, shrubland, grasslands, or on manicured lawns.
Geometry type: POLYGON
land_use tags | Description |
---|---|
| This tag specifies to which group a given feature belongs.
|
| This tag narrows down the group values allowing fine-grained styling
and provides a distinction between land uses in the same group.
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
|
| Fine-grain attribution of each feature corresponding to the most
relevant value found among different primary tags (leisure, landuse,
boundary, etc...) for more precise filtering or custom styling.
|
| A numerical value attribute that enables ordering and filtering based on
the ranking of the area. The values are in the range 1, 16 inclusive. |
| Indicates that area is an underground areas, such as subway platform, parking, etc. |
| Attribution to qualify the main mobility type of a passenger transportation feature.
|
| Indicates main profile of use of an area.
|
structures
Features in this layer represent man-made structures.
Geometry type: POLYGON
structures tags | Description |
---|---|
| Provides macro-classification based on the main type of structure.
|
| Provides fine-grained classification based on the specific function of the structure to enable filtering depending on the use case.
|
| The integer value used to determine the drawing order of overlapping structure segments. The values are in the range -5, 5 inclusive. |
structures_lines
Features in this layer represent man-made structure lines.
Geometry type: LINESTRING
structures_lines tags | Description |
---|---|
| Provides macro-classification based on the main type of structure.
|
| Provides fine-grained classification based on the specific function of the structure to enable filtering depending on the use case.
|
| The integer value used to determine the drawing order of overlapping
structure segments. The values are in the range -5, 5 inclusive. |
overlays
Features in this layer represent areas of the earth's surface based on special status with a prescribed use/restrictions established by different entities.
Geometry type: POLYGON
overlays tags | Description |
---|---|
| This tag specifies to which group a given feature belongs.
|
| This tag narrows down the group values allowing fine-grained styling
and provides a distinction between areas in the same group.
|
| Fine-grain attribution of each feature corresponding to the most
relevant value found among different primary tags (leisure, landuse,
boundary, etc...) for more precise filtering or custom styling.
|
| A numerical value attribute that enables ordering and filtering based on
the ranking of the area. The values are in the range 1, 16 inclusive. |
| A numerical value attribute that enables ordering and filtering based on
the protection status of the area. The values are in the range 1, 6 inclusive. |
built_up_areas
The built_up_areas layer contains features that represent an urbanized area with a large/dense concentration of buildings.
Geometry type: POLYGON
built_up_areas tags | Description |
---|---|
| This tag specifies to which category a given feature belongs. |
transit
The transit layer contains line geometries that represent public and private transit network for person and goods. It conflates entities coming from different means of transportation.
Geometry type: LINESTRING
transit tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between features in the same category.
Values for category
Values for category
|
| The integer value used to determine the drawing order of overlapping
transit segments. The values are in the range -5, 5 inclusive. |
| This tag indicates that the feature is part of a bridge. The value is
always true. If the feature is not a part of a bridge, then this tag is
absent. |
| This tag indicates that the feature is part of a tunnel. The value is
always true. If the feature is not a part of a tunnel, then this tag is
absent. |
| This tag indicates minor tracks present in proximity of railway stations
and yards. The value is always true if applicable, otherwise absent. |
| This tag indicates that the feature is under construction. The value is
always true. If the feature is not under construction, then this tag is
absent. |
| This tag indicates that it is necessary to pay a toll to use a transit.
The value is always true. If the transit is not paid, the this tag is
absent. |
| Attribution to qualify the main mobility type of a passenger transportation feature.
|
| A transit name in an NGT (Neutral Ground Truth) language; the native
language of each country, respectively. |
| A transit name in a language specified in a [language] suffix. List of supported languages. |
| This tag contains reference numbers or code for a route or transit segment. |
transit_areas
The transit_areas layer contains public and private transit areas/facilities for person and goods. It conflates entities coming from different means of transportation.
Geometry type: POLYGON
transit_area tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between features in the same category.
|
| The integer value used to determine the drawing order of overlapping
transit areas. The values are in the range -5, 5 inclusive. |
water
The water layer contains area geometries that represent oceans, seas, lakes, and other water bodies.
Geometry type: POLYGON
water tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| Indicates whether the water body does not permanently contain water,
including seasonal variation. Always true, absent if not applicable. |
| A numerical value attribute that enables ordering and filtering based on
the ranking of the area. The values are in the range 1, 15 inclusive. |
buildings
This layer represents building footprints.
Geometry type:POLYGON
buildings tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| Fine-grain attribution of each building feature corresponding to the
most relevant value found among different feature tags for more precise
filtering or custom styling.
|
| Indicates that building is underground, such as subway station, parking, etc. |
| Building height represents the height of the Building Footprint measured
from the ground level to the top of the structure. When a Building
Footprint has a ground height, building height represents the height of
the actual structure. The height is represented in meters. |
| Ground height represents the height of the Building Footprint measured
from the ground level to the bottom of the structure. The height is
represented in meters. |
| Indicates that building is part of bigger structure. Allows you to
differentiate a building into parts that differ from each other in
terms of some building attributes or the building function. |
water_lines
The water_lines layer contains line geometries that represent river and stream center lines.
Geometry type: LINESTRING
water_lines tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| Indicates whether the water body is man-made or natural. Always true, absent if not applicable. |
| Indicates whether the water body does not permanently contain water,
including seasonal variation. Always true, absent if not applicable. |
| This tag indicates that the water body is part of a tunnel. The value is
always true. If the water body is not a part of a tunnel, then this tag is
absent. |
| This tag indicates that the water body is part of a bridge. The value is
always true. If the water body is not a part of a bridge, then this tag is
absent. |
| A feature name in a NGT (Neutral Ground Truth) language. |
| A feature name in a language specified in a [language] suffix. |
places
This layer contains points for labeling places including countries, states, cities, and towns.
Geometry type: POINT
places tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between places in the same category. Values for category
Values for category
|
| A feature name in an NGT (Neutral Ground Truth) language; the native
language of each country, respectively. |
| A feature name in a language specified in a [language] suffix. |
| Indicates if a settlement is the capital of an administrative area of a given level (2=country, 4=state, etc.). The values are in the range 2, 11 inclusive. |
| Represents a classification of places based on their importance with respect to map display. The values are in the range 1, 12 inclusive. |
| The identifier of the icon asset in the TomTom styles that should be
used for the visualization purpose of the Point of Interest.
|
| A unique place identifier that can be used across other TomTom services. |
| ISO-3166 code of the country for a given label point. |
| ISO-3166 code of state or first sub-national subdivision for label points. |
| Minimum zoom level at which a feature must be visualized. |
| Maximum zoom level at which feature must be visualized. |
poi
The POI layer represents points of interest.
Geometry type: POINT
poi tags | Description |
---|---|
| This tag provides a broad grouping of the Points of Interest entities based on general semantic affiliations.
|
| This tag groups Points of Interest into broad categories that can be
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
Values for group
|
| Fine-grain attribution of each POI feature corresponding to the
|
| A numerical value attribute that enables ordering and filtering based on |
| The tag value represents an absolute priority of the POI among all the POI features. |
| The tag value represents a use profile for which the POI entity is relevant.
|
| Attribution to qualify the main mobility type of a passenger transportation feature.
|
| A POI name in an NGT (Neutral Ground Truth) language; the native |
| A POI name in a language specified in a [language] suffix. |
| Attribute that captures additional information about POIs, depending on their group affiliation. |
| Attribute that captures POI brand information. |
| A unique POI identifier that can be used across other services. |
| Semantic id associated to each feature |
carto_labels
The carto_labels layer includes points that represent cartographic labels.
Geometry type: POINT
carto_labels tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling and provides a distinction between places in the same category.
Values for category
Values for category
Values: for category
Values: for category
Values: for category
|
| Indicates whether the water body does not permanently contain water,
including seasonal variation. Always true, absent if not applicable. |
| A numerical value attribute that enables ordering and filtering based on the size of the corresponding feature or other ranking method. The values are in the range 0, 13 inclusive. |
| A feature name in an NGT (Neutral Ground Truth) language; the native
language of each country, respectively. |
| A feature name in a language specified in a [language] suffix. List of supported languages. |
| A numeric value attribute representing the elevation of the point in meters above the sea level. |
| Minimum zoom level at which a feature must be visualized. |
| Maximum zoom level at which feature must be visualized. |
roads
The roads layers include lines that can be used for drawing roads.
Geometry type: LINESTRING
roads tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between places in the same category.
Values: for category
Values: for category
|
| This tag indicates that traffic on a road is one-way. Value 1 indicates
that the traffic flows in the same direction as geometry of the road,
and the value -1 indicates that the traffic flows in the opposite
direction to the geometry of the road.
|
| This tag indicates that the road is part of a bridge. The value is
always true. If the road is not a part of a bridge, then this tag is
absent. |
| This tag indicates that the road is part of a tunnel. The value is
always true. If the road is not a part of a tunnel, then this tag is
absent. |
| Indicates that the road is covered but open at least on one side. The
value is always true, If road is not covered or fully covered this tag is absent. |
| The integer value used to determine the drawing order of overlapping
road segments. The values are in the range -5, 5 inclusive. |
| This tag indicates that the road is under construction. The value is
always true. If the road is not under construction, then this tag is
absent. |
| A numerical value attribute that enables ordering and filtering based on
the ranking of the road. The values are in the range 5, 15 inclusive. |
| This tag indicates that the road surface is not paved. The value is
always true. If the road is paved then this tag is absent. |
| This tag indicates that it is necessary to pay a toll to use a road. The
value is always true. If the road is not paid, then this tag is absent. |
| Depending on the road type, indicates the degree of difficulty to be
expected by the user (depending on either the steepness, level of maintenance,
etc). The values are in the range -6, 6 inclusive. |
access | Indicates if the road is access-restricted to the general public, to
some specific use or category or based on certain conditions (e.g., time, day).
|
| A road name in an NGT (Neutral Ground Truth) language; the native
language of each country, respectively. |
| A road name in a language specified in a [language] suffix. List of supported languages. |
| Text to be placed on the road shield icon. |
| Primary route shield text for a given road to be used as the label of the road shield symbol. |
| Alternate route shield(s) text for a given road to be used as the label of the road shield symbol. |
| Primary route shield id for a given road to reference a unique route type id. |
| Alternate route shield(s) id for a given road to reference a unique route type id. |
| Primary route shield icon name for a given road to be used to source the asset of the road shield symbol. |
| Alternate route shield(s) icon name for a given road to be used to source the asset of the road shield symbol. |
| Primary route shield text color for a given road to style the text in the road shield symbol. |
| Alternate route shield(s) text color for a given road to style the text in the road shield symbol. |
roads_areas
The roads_areas layers include polygons that can be used for drawing road areas.
Geometry type: POLYGON
roads_area tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag indicates that the road area is part of a bridge. The value is
always true. If the road area is not a part of a bridge, then this tag is
absent. |
| This tag indicates that the road area is part of a tunnel. The value is
always true. If the road area is not a part of a tunnel, then this tag is
absent. |
| Indicates that the road area is covered but open at least on one side. The
value is always true, If road area is not covered or fully covered this tag is absent. |
| The integer value used to determine the drawing order of overlapping
road areas. The values are in the range -5, 5 inclusive. |
| A numerical value attribute that enables ordering and filtering based on
the ranking of the road. The values are in the range 5, 15 inclusive. |
| This tag indicates that the road surface is not paved. The value is
always true. If the road is paved then this tag is absent. |
| Indicates if the road is access-restricted to the general public, to
some specific use or category or based on certain conditions (e.g. time, day).
|
roads_points
Features in this layer represent man-made structures.
Features in this layer represent points related to the road network, including road furniture (traffic lights and signs), junction/exit points, special traffic figures (mini roundabouts, turning loops, etc.) and other relevant features.
Geometry type: POLYGON
roads_points tags | Description |
---|---|
| Provides macro-classification based on the main type of road-related point.
|
| Provides fine-grained classification based on the original definition of the feature to enable filtering depending on the use case.
|
| The highway exit reference number to be visualized on the point. |
| The highway exit name to be visualized on the point on higher zoom levels if needed. |
| The main destination name of the highway exit to be visualized on the point on higher zoom levels if needed. |
| ISO-3166 country code of the highway exit. |
boundaries
The boundaries layer includes boundary lines for national and subnational administrative units.
Geometry type: LINESTRING
boundaries tags | Description |
---|---|
| This tag specifies to which category a given feature belongs.
|
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between boundaries in the same category.
|
| This tag indicates that the border is the result of an agreement under
international law entered by sovereign states and international
organizations but is still experienced as a very sensitive geopolitical
matter. |
| This tag indicates that the border is the subject of a dispute between
two or more countries. It does not give information about the nature of
the dispute. |
| A feature name in an NGT (Neutral Ground Truth) language; the native
language of each country, respectively. |
| A feature name in a language specified in a [language] suffix. See the List of supported languages. |
| Minimum zoom level at which a feature must be visualized. |
| Maximum zoom level at which a feature must be visualized. |
| Minimum zoom level at which a feature label must be visualized. |
| Maximum zoom level at which a feature label must be visualized. |
Handling label translations
Vector tiles contain all available translations for suitable layers and features, i.e., POINT features in the places layer. These translations can be used in style files to change the label language. In order to use translations, you need to modify the desired layer in the style file by replacing the value of the text-field
key with the following expression:
["coalesce",["get", "name_[language]"],["get", "name"]]
Important note:
The [language]
part in name_[language]
needs to be replaced by one of the supported languages.
Example:
1{2 ...3 "id": "Places - Capital",4 "layout": {5 "text-anchor": "center",6 "text-field": ["coalesce",["get", "name_en-GB"],["get", "name_en"],["get", "name"]],7 "text-font": ["Noto-Bold"],8 "text-justify": "auto",9 "text-letter-spacing": 0.05,10 ...11 }12 ...13}
To combine the translated label with other tags the following expression can be used:
["concat",["coalesce",["get", "name_[language]"],["get", "name"]], ["get", "[tag_name]"]]
Important notes:
- The
[language]
part inname_[language]
needs to be replaced by one of the supported languages. - The
[tag_name]
should be replaced by one of the tags available in the version 2 tile.
Example:
1{2 ...3 "id": "POI",4 "layout": {5 "text-anchor": "center",6 "text-field": ["concat",["coalesce",["get', "name_en-GB"],["coalesce",["get', "name_en"],["get", "name"]], "\n", ["get", "sub_text"]],7 "text-font": ["Noto-Bold"],8 "text-justify": "auto",9 "text-letter-spacing": 0.05,10 ...11 }12 ...13}