Content
Public Preview Notice
This API is in Public Preview. Go to the Public Preview - what is it? page to see what this means.
TomTom Vector Format
TomTom Vector tiles contain map features (points, lines, road shapes, water polygons, building footprints, etc.) that can be used to visualize map data. The data is divided into multiple protobuf layers. Besides the layers, the protobuf tags are also used to further describe the geographic features.
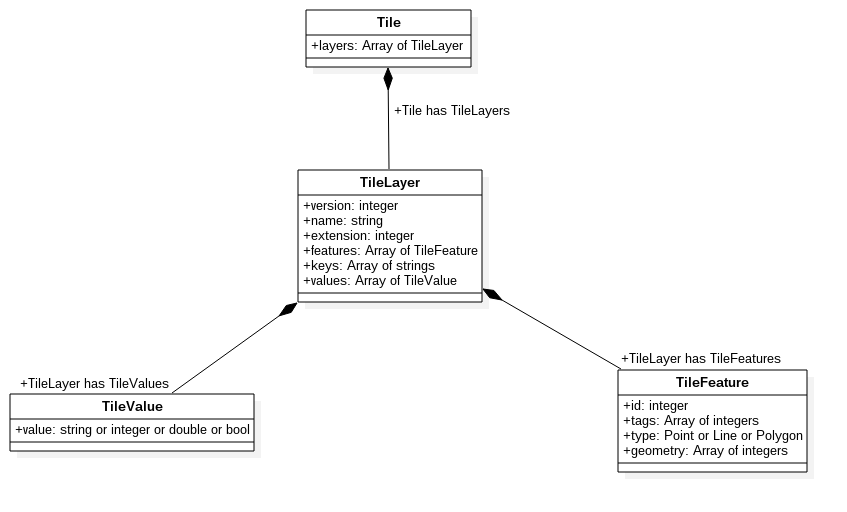
Legend
Tile
Represents a vector tile as a whole entity. It consists of at least one
TileLayer
.
If the tile does not contain any relevant data, it contains one layer namedempty
.TileLayer
Part of a
Tile
, representing one of the layers that can be rendered later. One layer consists of e.g., all Parks, Roads, Built-up areas, or Points of interest.Each
TileLayer
contains geometries of the same type. A geometry in the vector tile can be one of three types:POINT
,LINESTRING
, orPOLYGON
. EveryTileLayer
contains the following fields:name
version
extent
It may also contain the following fields:
keys
is an array of uniquestrings
.values
is an array of uniqueTileValues
.features
is an array of uniqueTileFeatures
.
Arrays of
keys
andvalues
contain the mapping for tags ofTileFeature.
TileFeature
Member of an array of
features
stored in aTileLayer
, containing a geometry representation that can be rendered.It always contains the following fields:
type
is a type of geometry. It can bePOINT
,LINESTRING
, orPOLYGON
.geometry
is an array of encoded geometry that can be used for rendering. See the Decoding tile geometry section for details.
It may also contain the following fields:
tags
is an array containing properties of feature.They are a
TileFeature's
detailed description which can be used for styling and later rendering the geometry.- They are encoded into an array of integers.
In order to decode them, the arrays of
keys
andvalues
from a correspondingTileLayer
must be used.See the Decoding feature tile tags section for details.
TileValue
Encoded representation of a string, integer, floating point, or boolean value.
It is stored in a
values
array, in aTileLayer
.It is used for decoding
tags
of aTileFeature
(together with the members of akeys
array).See the Decoding feature tile tags section for details.
TomTom Vector Schema
Schema data
Formally, the structure of a vector tile is described by the protocol buffer schema. It allows the generation of C++, Java, Python, Go, Ruby, Objective-C, and C# code based on the proto file.
1package vector_tile23option optimize_for = LITE_RUNTIME;45message Tile {67 enum GeomType {8 UNKNOWN = 0;9 POINT = 1;10 LINESTRING = 2;11 POLYGON = 3;12 }1314 message Value {15 optional string string_value = 1;16 optional float float_value = 2;17 optional double double_value = 3;18 optional int64 int_value = 4;19 optional uint64 uint_value = 5;20 optional sint64 sint_value = 6;21 optional bool bool_value = 7;22 extensions 8 to max;23 }2425 message Feature {26 optional uint64 id = 1 [ default = 0 ];27 repeated uint32 tags = 2 [ packed = true ];28 optional GeomType type = 3 [ default = UNKNOWN ];29 repeated uint32 geometry = 4 [ packed = true ];30 optional bytes raster = 5;31 }3233 message Layer {34 required uint32 version = 15 [ default = 1 ];35 required string name = 1;36 repeated Feature features = 2;37 repeated string keys = 3;38 repeated Value values = 4;39 optional uint32 extent = 5 [ default = 4096 ];4041 extensions 16 to max;42 }4344 repeated Layer layers = 3;4546 extensions 16 to 8191;47}
Decoding tile geometry
Vector Tile geometry uses the following coordinate system:
- Coordinates are always integers.
- The
(0,0)
point is located in the upper-left corner of the tile. - The X axis has values increasing towards the right of the tile.
- The Y axis has values increasing towards the bottom of the tile.
- The tile may have margin, which is a buffer around the tile in the shape of a square frame. The size of the margin is by default equal to 10% of the width/length of the tile.
- The extent is equal to
4096
, so the value range for X and Y coordinates is from0
to4095
for visible points. - If the tile has a margin, the coordinates values range is extended by its size in both directions. This may cause coordinate values for points in the left or upper margin to be negative.
Vector Tile geometry is encoded as an array of 32 bit unsigned integers in the geometry
array field of the TileFeature
.
Encoded format has the following structure:
[command_and_count][x0][y0]..[xn][yn][command_and_count][x0][y0]..
command_and_count
contains encoded values ofcommand
andcount
.[x0][y0]..[xn][yn]
are(x,y)
coordinate pairs.
Command
The value of command
is decoded as follows:
command = command_and_count & 0x7
Commands are to be executed relative to previous path coordinates or the point (0,0)
if it is the first command.
Command type | Value | Coordinates | Description |
---|---|---|---|
|
|
| It defines the
|
|
|
| It defines a new segment, starting at the current position of the cursor
and ending at the
|
|
| none | It closes the current linear ring of a |
Count
The value of count
is decoded as follows:
count = command_and_count >> 0x3
- It defines the
n
number of[xn][yn]
encoded coordinate pairs following thecommand_and_count
value. - These coordinate pairs must be interpreted according to the preceding
command
type.
Coordinates
Coordinates [x0][y0]..[xn][yn]
are encoded in zigzag encoding and are relative to the previous coordinate pair. This means that only the first coordinate pair [x0][y0]
in the first command in every TileFeature
stores absolute values.
The coordinates are decoded as follows:
1decode(x0) = ((x0 >> 0x1) ^ (-(x0 & 0x1)))2decode(y0) = ((y0 >> 0x1) ^ (-(y0 & 0x1)))34decode(x1) = decode(x0) + ((x1 >> 0x1) ^ (-(x1 & 0x1)))5decode(y1) = decode(y0) + ((y1 >> 0x1) ^ (-(y1 & 0x1)))67...89decode(xn) = decode(xn-1) + ((xn >> 0x1) ^ (-(xn & 0x1)))10decode(yn) = decode(yn-1) + ((yn >> 0x1) ^ (-(yn & 0x1)))
Examples
For a POINT
type feature:
Input:
1layer: 02 feature: 03 type: POINT4 geometry: [9, 1136, 6564]
Decoding:
1geometry:2 command_and_count = 93 command = 9 & 0x7 = 14 count = 9 >> 0x3 = 156 x= 1136, y0 = 65647 decode(x0) = ((1136 >> 0x1) ^ (-(1136 & 0x1))) = 5688 decode(y0) = ((6564 >> 0x1) ^ (-(6564 & 0x1))) = 3282
Output:
1layer: 02 feature: 03 geometry: POINT(561, 3282)
For a LINESTRING
type feature:
Input:
1layer: 02 feature: 03 type: LINESTRING4 geometry: [9, 846, 2312, 10, 652, 1938]
Decoding:
1geometry:2 1:3 command_and_count = 94 command = 9 & 0x7 = 15 count = 9 >> 0x3 = 167 x0' = 846, y0' = 23128 decode(x0') = ((846 >> 0x1) ^ (-(846 & 0x1))) = 4239 decode(y0') = ((2312 >> 0x1) ^ (-(2312 & 0x1))) = 115610 2:11 command_and_count = 1012 command = 10 & 0x7 = 213 count = 10 >> 0x3 = 11415 x0 = 652, y0 = 193816 decode(x0) = decode(x0') + ((x0 >> 0x1) ^ (-(x0 & 0x1)))17 decode(y0) = decode(y0') + ((y0 >> 0x1) ^ (-(y0 & 0x1)))18 decode(x0) = 423 + ((652 >> 0x1) ^ (-(652 & 0x1))) = 74919 decode(y0) = 1156 + ((1938 >> 0x1) ^ (-(1938 & 0x1))) = 2125
Output:
1layer: 02 feature: 03 geometry: LINESTRING[(423, 1156), (749, 2125)]
For a POLYGON
type feature:
Input:
1layer: 02 feature: 03 type: POLYGON4 geometry: [9, 1320, 5622, 26, 416, 707, 68, 612, 483, 96, 7]
Decoding:
1geometry:2 1:3 command_and_count = 94 command = 9 & 0x7 = 15 count = 9 >> 0x3 = 167 x0' = 1320, y0' = 56228 decode(x0') = ((1320 >> 0x1) ^ (-(1320 & 0x1))) = 6609 decode(y0') = ((5622 >> 0x1) ^ (-(5622 & 0x1))) = 281110 2:11 command_and_count = 2612 command = 26 & 0x7 = 213 count = 26 >> 0x3 = 31415 x0 = 1736, y0 = 491416 decode(x0) = decode(x0') + ((416 >> 0x1) ^ (-(416 & 0x1))) = 660 + 208 = 86817 decode(y0) = decode(y0') + ((707 >> 0x1) ^ (-(707 & 0x1))) = 2811 + (-354) = 24571819 x1 = 68, y1 = 61220 decode(x1) = decode(x0) + ((68 >> 0x1) ^ (-(68 & 0x1))) = 90221 decode(y1) = decode(y0) + ((612 >> 0x1) ^ (-(612 & 0x1))) = 27632223 x2 = 483, y2 = 9624 decode(x2) = decode(x1) + ((483 >> 0x1) ^ (-(483 & 0x1))) = 66025 decode(y2) = decode(y1) + ((96 >> 0x1) ^ (-(96 & 0x1))) = 281126 3:27 command_and_count = 728 command = 7 & 0x7 = 729 count = 7 >> 0x3 = 0
Output:
1layer: 02 feature: 03 geometry: POLYGON[(660, 2811), (868, 2457), (902, 2763), (660, 2811)]
Decoding feature tags
In order to reduce tile size, the properties of each feature are encoded in a tags
array.
- The
tags
array contains integer values which are indexes ofkeys
andvalues
arrays belonging to the corresponding layer. - The size of a
tags
array is always even. - The content of a
tags
array can be grouped into a list of pairs. Each odd element of an array is the first element of a pair, and each even element of an array is the second element of a pair. - The first element of each pair should be mapped to a
keys
array. - The second element of each pair should be mapped to a
values
array. - As a result, we get a decoded list of
tags
where the first element is the tag name and the second element is the tag value in the form ofTileValue
.
Example
Below is an example of what an encoded tile may look like.
1layer: 02 keys: ["country_code", "icon_text"],3 values: ["SWE", "E4"]4 feature: 05 tags : [0,0,1,1]
After decoding it has to be read like this:
1layer: 02 feature: 03 properties:4 country_code : "SWE"5 icon_text : "E4"
Tile Layers
The data in TomTom Vector Tiles is organized in layers. The following section provides detailed information about:
- Available layers.
- Tags that typically appear in features from a given layer.
earth_cover
Earth cover refers to the generalized surface cover of the earth, whether vegetation, sand, ice, or other.
Geometry type: POLYGON
earth_cover tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
land_cover
Land cover refers to the surface cover on the ground, whether vegetation, urban infrastructure, or other; it does not describe the use of land, and the use of land may be different for lands with the same cover type. For instance, a land cover type of forest may be used for timber production, wildlife management, or recreation; it might be private land, a protected watershed, or a popular state park.
Geometry type: POLYGON
land_cover tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
land_use
The land use refers to the purpose the land serves, for example, recreation, wildlife habitat or agriculture; it does not describe the surface cover on the ground. For example, a recreational land use could occur in a forest, shrubland, grasslands, or on manicured lawns.
Geometry type: POLYGON
land_use tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
subcategory string | For category
For category
For category
For category
For category
For category
For category
| This tag narrows down the category values allowing fine-grained styling and provides a distinction between land uses in the same category. |
surface string | For
| This tag represents the dominant cover of a land use feature. |
protected boolean | For
| This tag indicates that the selected area is protected. |
urban boolean | For
| This tag indicates that the selected area is part of an urban area. |
leisure boolean | For
| This tag indicates that the selected area is a leisure area. |
transportation boolean | For
| This tag indicates that the selected area is related to transportation. |
vegetation boolean | For
| This tag indicates that the selected area belongs to the vegetation group. |
has_ban boolean | For
| This tag indicates that there is a zone with a ban regulation. 'Ban' indicates that access to a zone is prohibited when a vehicle matches the characteristics defined in the Emission Regulation. Exceptions may be made for vehicles involved in specific activities, e.g., construction or goods delivery, with appropriate documentation. This restriction cannot be mitigated by paying some charge.
This tag only contains a |
has_fee boolean | For
| This tag indicates that there is a zone with a fee regulation. 'Fee' indicates that access to a zone is allowed upon paying a fee when a vehicle matches the characteristics defined in the Emission Regulation. The fee can be charged on a recurring, short-period basis (e.g., daily or per entry), with the amount often dependent on the vehicle's emission class, e.g., higher polluting vehicles are charged a larger fee.
This tag only contains a |
has_license boolean | For
| This tag indicates that there is a zone with a license plate regulation.
This tag only contains a |
allow_category integer | For
| Tag indicates the status of a zone restriction. The lack of this tag means that a zone entry is allowed. |
requires_vignette boolean | For
| This tag indicates that access to low emission zone is restricted
to vehicles that have an officially-issued vignette.
This tag only contains a |
water
The water layer contains area geometries that represent oceans, seas, lakes, and other water bodies.
Geometry type: POLYGON
water tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
buildings
This layer represents building footprints.
Geometry type: POLYGON
buildings tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
subcategory string | For category
| This tag narrows down the category values allowing fine-grained styling, and provides a distinction between land uses in the same category. |
height float | - | Building height represents the height of the Building Footprint measured from the ground level to the top of the structure. When a Building Footprint has a ground height, building height represents the height of the actual structure. The height is represented in meters. |
ground_height float | - | Ground height represents the height of the Building Footprint measured from the ground level to the bottom of the structure. The height is represented in meters. |
water_lines
The water_lines layer contains line geometries that represent river and stream center lines.
Geometry type: LINESTRING
water_lines tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
subcategory string | For category
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between places in the same category. Please
note that the river subcategory is also present in the
|
name string | - | A feature name in a NGT (Neutral Ground Truth) language. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. |
places
This layer contains points for labeling places including countries, states, cities, and towns.
Geometry type: POINT
places tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
subcategory string | For category
For category
| This tag narrows down the category values allowing fine-grained styling and provides a distinction between places in the same category. |
name string | - | A feature name in an NGT (Neutral Ground Truth) language; the native language of each country, respectively. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. List of supported languages. |
abbr string | - | This tag provides an abbreviation of the state name. It will only be present for features with category state. |
capital string |
| This tag value indicates the administrative importance of point. |
priority integer | - | The tag value represents a priority of the city which is based on the combination of the population of the city and its administrative importance. The lower the value of this tag the more important the city is. |
admin_class integer | - | The tag value represents the administrative importance of a place. |
display_class integer | - | The tag value represents a classification of places based on their importance with respect to map display. |
icon string | - | The identifier of the icon asset in the TomTom styles that should be used for the visualization purpose of the Point of Interest. |
id integer | - | A unique place identifier that can be used across other TomTom services. |
number string | - | Address point (house) number. Available only when the
|
poi_basic
The POI layers represent points of interest. The difference between the poi_basic
and the poi_extended
layer is the amount of available categories.
Geometry type: POINT
poi_basic tags | Values | Description |
---|---|---|
category string |
| This tag groups Points of Interest into broad categories that can be used for styling purposes. |
subcategory string | For category
For category
For category
For category
For category
| This tag narrows down the category values allowing fine grained styling and provides a distinction between places in the same category. |
name string | - | A feature name in an NGT (Neutral Ground Truth) language; the native language of each country, respectively. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. List of supported languages. |
id string | - | A unique Point of Interest identifier that can be used across other TomTom services. |
category_id integer | - | An identifier representing a category. Either a 4 digits integer for Points of Interest without subcategory or a 7 digits integer for Points of Interest with subcategory (where the first 4 digits identify a category). It can be used across other TomTom services. |
priority integer | - | The tag value represents a priority of the Point of Interest. The lower the value of this tag the more important the Point of Interest is. |
icon integer | - | The identifier of the icon asset in the TomTom styles that should be used for the visualization purpose of the Point of Interest. |
sub_text string | - | The additional information about POIs, i.e., if available, for a hill it contains its height in meters, in the countries where imperial units are used the height is in feet. |
The Category ID and Subcategory ID in the following table correspond to the ID in poiCategories in the Search API.
Category | Category ID | Possible subcategories | Subcategory ID |
---|---|---|---|
industrial_building | 9383 | - | - |
college_or_university | 7377 | - | - |
fire_station_or_brigade | 7392 | - | - |
government_office | 7367 | - | - |
library | 9913 | - | - |
military_installation | 9388 | - | - |
native_reservation | 9389 | - | - |
police_station | 7322 | - | - |
post_office | 7324 | - | - |
prison_or_correctional_facility | 9154 | - | - |
school | 7372 | - | - |
hospital | 7321 | - | - |
amusement_park | 9902 | - | - |
museum | 7317 | - | - |
park_and_recreation_area | 9362 | national_park cemetery | 9362008 9362003 |
zoo_arboreta_and_botanical_garden | 9927 | - | - |
shopping_center | 7373 | - | - |
golf_course | 9911 | - | - |
stadium | 7374 | - | - |
tourist_attraction | 7376 | - | - |
place_of_worship | 7339 | church mosque synagogue temple gurudwara ashram pagoda | 7339002 7339003 7339004 7339005 7339006 7339007 7339008 |
airport | 7383 | - | - |
ferry_terminal | 7352 | - | - |
public_transportation_stop | 9942 | bus_stop taxi_stand coach_stop streetcar_stop | 9942002 9942003 9942005 9942004 |
railroad_station | 7380 | international_railroad_station national_railroad_station subway_station | 738002 738003 738005 |
geographic_feature | 8099 | mountain_peak ridge valley reef hill | 8099002 8099004 8099006 8099014 8099025 |
poi_extended
The POI layers represent points of interest. The difference between the poi_basic
and the poi_extended
layer is the amount of available categories.
Geometry type: POINT
poi_extended tags | Values | Description |
---|---|---|
category string |
| This tag groups Points of Interest into broad categories that can be used for styling purposes. |
subcategory string | For category
For category
For category
For category
For category
For category
| This tag narrows down the category values allowing fine-grained styling and provides a distinction between places in the same category. |
name string | - | A feature name in an NGT (Neutral Ground Truth) language; the native language of each country, respectively. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. List of supported languages. |
id string | - | A unique Point of Interest identifier that can be used across other TomTom services. |
category_id integer | - | An identifier representing category. Either 4 digits integer for Points of Interest without subcategory or 7 digits integer for Points of Interest with subcategory (where the first 4 digits identify a category). It can be used across other TomTom services. |
priority integer | - | The tag value represents a priority of the Point of Interest. The lower the value of this tag the more important the Point of Interest is. |
icon integer | - | The identifier of the icon asset in the TomTom styles that should be used for the visualization purpose of the Point of Interest. |
The Category ID and Subcategory ID in the following table correspond to the ID in poiCategories in the Search API.
Category | Category ID | Possible subcategories | Subcategory ID |
---|---|---|---|
automotive_dealer | 9910 | - | - |
car_wash | 9155 | - | - |
electric_vehicle_station | 7309 | - | - |
motoring_organization_office | 7368 | - | - |
open_parking_area | 7369 | - | - |
parking_garage | 7313 | - | - |
gas_station | 7311 | - | - |
rent_a_car_facility | 7312 | - | - |
rent_a_car_parking | 9930 | - | - |
repair_facility | 7310 | - | - |
rest_area | 7395 | - | - |
toll_gate | 7375 | - | - |
truck_stop | 7358 | - | - |
weigh_station | 7359 | - | - |
agriculture_business | 7335 | - | - |
bank | 7328 | - | - |
business_park | 7378 | - | - |
atm | 7397 | - | - |
commercial_building | 9382 | - | - |
company | 9352 | - | - |
courier_drop_box | 7388 | - | - |
emergency_medical_service | 7391 | - | - |
exchange | 9160 | - | - |
exhibition_and_convention_center | 9377 | - | - |
industrial_building | 9383 | - | - |
manufacturing_facility | 9156 | - | - |
media_facility | 9158 | - | - |
research_facility | 9157 | - | - |
cafe_or_pub | 9376 | cafe pub internet_cafe tea_house coffee_shop microbrewery | 9376002 9376003 9376004 9376005 9376006 9376007 |
restaurant | 7315 | - | - |
restaurant_area | 9359 | - | - |
college_or_university | 7377 | - | - |
courthouse | 9363 | - | - |
embassy | 7365 | - | - |
fire_station_or_brigade | 7392 | - | - |
government_office | 7367 | - | - |
library | 9913 | - | - |
military_installation | 9388 | - | - |
native_reservation | 9389 | - | - |
non_governmental_organization | 9152 | - | - |
police_station | 7322 | - | - |
post_office | 7324 | - | - |
primary_resource_or_utility | 9150 | - | - |
prison_or_correctional_facility | 9154 | - | - |
public_amenity | 9932 | - | - |
school | 7372 | - | - |
traffic_service_center | 7301 | - | - |
transport_authority_or_vehicle_registration | 9151 | - | - |
welfare_organization | 9153 | - | - |
dentist | 9374 | - | - |
doctor | 9373 | - | - |
emergency_room | 9956 | - | - |
health_care_service | 9663 | - | - |
hospital | 7321 | - | - |
pharmacy | 7326 | - | - |
veterinarian | 9375 | - | - |
amusement_park | 9902 | - | - |
casino | 7341 | - | - |
movie_theater | 7342 | - | - |
club_and_association | 9937 | - | - |
community_center | 7363 | - | - |
cultural_center | 7319 | - | - |
entertainment | 9900 | - | - |
leisure_center | 9378 | - | - |
marina | 7347 | - | - |
museum | 7317 | - | - |
nightlife | 9379 | - | - |
park_and_recreation_area | 9362 | national_park cemetery | 9362008 9362003 |
theater | 7318 | - | - |
trail_system | 7302 | - | - |
winery | 7349 | - | - |
zoo_arboreta_and_botanical_garden | 9927 | - | - |
camping_ground | 7360 | - | - |
vacation_rental | 7304 | - | - |
hotel_or_motel | 7314 | - | - |
residential_accommodations | 7303 | - | - |
department_store | 7327 | - | - |
market | 7332 | - | - |
shop | 9361 | - | - |
shopping_center | 7373 | - | - |
golf_course | 9911 | - | - |
ice_skating_rink | 9360 | - | - |
sports_center | 7320 | - | - |
stadium | 7374 | - | - |
swimming_pool | 7338 | - | - |
tennis_court | 9369 | - | - |
water_sport | 9371 | - | - |
adventure_sports_venue | 7305 | - | - |
beach | 9357 | - | - |
tourist_attraction | 7376 | - | - |
place_of_worship | 7339 | church mosque synagogue temple gurudwara ashram pagoda | 7339002 7339003 7339004 7339005 7339006 7339007 7339008 |
scenic_or_panoramic_view | 7337 | - | - |
tourist_information_office | 7316 | - | - |
access_gateway | 7389 | - | - |
airport | 7383 | - | - |
checkpoint | 9955 | - | - |
ferry_terminal | 7352 | - | - |
frontier_crossing | 7366 | - | - |
helipad | 7308 | - | - |
mountain_pass | 9935 | - | - |
port_or_warehouse_facility | 9159 | - | - |
public_transportation_stop | 9942 | bus_stop taxi_stand coach_stop streetcar_stop | 9942002 9942003 9942005 9942004 |
railroad_station | 7380 | international_railroad_station national_railroad_station subway_station | 738002 738003 738005 |
geographic_feature | 8099 | mountain_peak cave ridge dune valley plain_or_flat plateau pan well oasis rocks reservoir reef rapids bay cove harbor lagoon cape mineral_or_hot_springs island marsh river_crossing hill quarry locale | 8099002 8099003 8099004 8099005 8099006 8099007 8099008 8099009 8099010 8099011 8099012 8099013 8099014 8099015 8099016 8099017 8099018 8099019 8099020 8099021 8099022 8099023 8099024 8099025 8099026 8099027 |
carto_labels
The carto_labels layer includes points that represent cartographic labels. It also includes lines for some features like river that can be used to place a label along the geometry of the river.
Geometry type: POINT
, LINESTRING
carto_labels tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
subcategory string | For category
| This tag narrows down the category values allowing fine-grained styling
and provides a distinction between places in the same category. Please
note that the river subcategory is also present in the
|
id string | - | A unique Point of Interest identifier that can be used across other TomTom services. |
icon string | - | The identifier of the icon asset in the TomTom styles that should be used for the visualization purpose of the Point of Interest. |
name string | - | A feature name in an NGT (Neutral Ground Truth) language; the native language of each country, respectively. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. List of supported languages. |
roads
The roads layers include lines that can be used for drawing roads.
Geometry type: LINESTRING
roads tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
subcategory string | For category
For category
For category
For category
For category
| This tag narrows down the category values allowing fine-grained styling and provides a distinction between places in the same category. |
tunnel boolean |
| This tag indicates that the road is part of a tunnel. The value is always true. If the road is not a part of a tunnel, then this tag is absent. |
toll boolean |
| This tag indicates that it is necessary to pay a toll to use a road. The value is always true. If the road is not paid, the this tag is absent. |
under_construction boolean |
| This tag indicates that the road is under construction. The value is always true. If the road is not under construction, then this tag is absent. |
bridge boolean |
| This tag indicates that the road is part of a bridge. The value is always true. If the road is not a part of a bridge, then this tag is absent. |
direction integer |
| This tag indicates that traffic on a road is one-way. Value 1 indicates that the traffic flows in the same direction as geometry of the road, and the value -1 indicates that the traffic flows in the opposite direction to the geometry of the road. |
shield_icon_[0-4] string | - | The name of the road shield icon associated with the road. For easier referencing and implementation, the name of a roadshield follows one of two formats:
See the Shield icon shape types section for existing shape types. See the Shield icon fill and stroke colors section for existing shield colors. See the Shield icon countries and states section for details on country names and state codes. numOfChars is a number in the range 1-7. shieldType is a unique shield ID. |
shield_icon_text_[0-4] string | - | Text to be placed on the road shield icon with the corresponding shield_icon idx. |
shield_icon_text_color_[0-4] string | - | The color of the text on the road shield icon with the corresponding shield_icon idx. Should be used in day mode. |
shield_icon_text_color_night_[0-4] string | - | The color of the text on the road shield icon with the corresponding shield_icon idx. Should be used in night mode. |
z_level integer | - | The integer value used to determine the drawing order of overlapping road segments. The values are in the range -3, 3 inclusive. Note: This tag is currently sent from zoom level 13 inclusive up to zoom level 22. |
name string | - | A feature name in an NGT (Neutral Ground Truth) language; the native language of each country, respectively. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. List of supported languages. |
<restriction> string | - | Value of restriction. The unit of this value is provided in the tag |
<restriction>_unit integer | - | Unit of restriction value.
Value is provided in the tag See: |
<restriction>_icon string | - | Road sign restriction icon.
|
<restriction>_priority integer | - | Priority of restriction road sign.
|
<restriction>_period string | - | Validity period in human-readable form.
If the restriction starts or ends in the nearest 24 hours, then the tag contains a string with restriction validity hours.
It contains a period in 12h or 24h format.
|
<restriction>_max_weight float | - | Max weight. |
allow_category integer |
| Tag indicates the status of restriction. |
restriction_direction integer | 1 -1 | The aggregated value of a restriction's direction. This tag indicates that all restrictions on the road are one-way. Value 1 indicates that all restrictions flow in the same direction as the geometry of the road, and the value -1 indicates that all restrictions flow in the opposite direction to the geometry of the road. |
left_hand_traffic boolean |
| This tag indicates that traffic is on the left side of the road.
This tag is present only together with tag |
Shield icon shape types
rectangle | hexagon | hexagon2 | octagon | special01 | special02 | special03 | special04 |
shield01 | shield02 | shield03 | shield04 | shield05 | shield06 | shield07 | shield08 |
shield09 | shield10 | shield11 | shield12 | pentagon | circle | pill | us1 |
us2 | us3 | oval | diamond |
Shield icon fill and stroke colors
white | yellow1 | yellow2 | yellow3 | orange | green1 | green2 | green3 | blue1 |
blue2 | blue3 | brown1 | brown2 | red | grey | anthracite | black |
Shield icon countries and states
Possible values of the countryName part of a name are listed in the following table.
- For Canada shields, the stateCode part of the shield's name is either a two-letter province name abbreviation or empty.
- For USA shields, the stateCode may be a two-letter ISO-3166 state name abbreviation,
highway
,county
, or empty. - For other country shields the stateCode is empty.
colombia | south | mexico | indonesia | taiwan | canada | usa | venezuela | southafrica |
cuba | india | australia | argentina | saudiarabia | uae | peru | korea |
boundaries
The boundaries layer includes boundary lines for national and subnational administrative units.
Geometry type: LINESTRING
boundaries tags | Values | Description |
---|---|---|
category string |
| This tag specifies to which category a given feature belongs. |
treaty boolean |
| This tag indicates that the border is the result of an agreement under international law entered by sovereign states and international organizations but is still experienced as a very sensitive geopolitical matter. |
disputed boolean |
| This tag indicates that the border is the subject of a dispute between two or more countries. It does not give information about the nature of the dispute. |
name string | - | A feature name in an NGT (Neutral Ground Truth) language; the native language of each country, respectively. |
name_[language] string | - | A feature name in a language specified in a [language] suffix. List of supported languages. |
List of supported languages
Language name | Language tag |
---|---|
Neutral Ground Truth (custom) |
Official languages for all regions in local scripts if available. |
Neutral Ground Truth - Latin exonyms (custom) |
Latin script will be used if available. |
Arabic |
|
Bulgarian |
|
Chinese (Taiwan) |
|
Chinese (Simplified) |
|
Czech |
|
Danish |
|
Dutch |
|
English (Australia) |
|
English (Canada) |
|
English (Great Britain) |
|
English (New Zealand) |
|
English (USA) |
|
Finnish |
|
French |
|
German |
|
Greek |
|
Hungarian |
|
Indonesian |
|
Italian |
|
Korean |
|
Korean written in the Latin script. |
|
Lithuanian |
|
Malay |
|
Norwegian |
|
Polish |
|
Portuguese (Brazil) |
|
Portuguese (Portugal) |
|
Russian written in the Cyrillic script. |
|
Russian written in the Latin script. |
|
Russian written in the Cyrillic script. Cyrillic script used where possible. |
|
Slovak |
|
Slovenian |
|
Spanish (Castilian) |
|
Spanish (Mexico) |
|
Swedish |
|
Thai |
|
Turkish |
|
Default language algorithm
The best match will be chosen based on the following algorithm.
Every
name
andname_[language]
tag provided in the tile is being matched from left to right. Partial match is allowed. For example:If
[language]
exactly match, the language is selected.If
[language]
does not have a match for a region, but a script subtag is available for another primary language subtag, then this other language will be used.
If there are multiple matches for a region, then the one with highest priority is used.
- If there is no match then NGT (Neutral Ground Truth) is used.
Handling label translations
Version 2 tiles contain all available translations for suitable layers and features, i.e., POINT features in the places layer. These translations can be used in style files to change the label language. In order to use translations, you need to modify the desired layer in the style file by replacing the value of the text-field
key with the following expression:
["coalesce",["get", "name_[language]"],["get", "name"]]
The [language]
part in name_[language]
needs to be replaced by one of the supported languages.
Example:
1{2 ...3 "id": "Places - Capital",4 "layout": {5 "text-anchor": "center",6 "text-field": ["coalesce",["get", "name_en-GB"],["get", "name"]],7 "text-font": ["Noto-Bold"],8 "text-justify": "auto",9 "text-letter-spacing": 0.05,10 ...11 }12 ...13}
To combine the translated label with other tags the following expression can be used:
["concat",["coalesce",["get", "name_[language]"],["get", "name"]], ["get", "[tag_name]"]]
- The
[language]
part inname_[language]
needs to be replaced by one of the supported languages. - The
[tag_name]
should be replaced by one of the tags available in the version 2 tile.
Example:
1{2 ...3 "id": "POI",4 "layout": {5 "text-anchor": "center",6 "text-field": ["concat",["coalesce",["get', "name_pl-PL"],["get", "name"]], "\n", ["get", "sub_text"]],7 "text-font": ["Noto-Bold"],8 "text-justify": "auto",9 "text-letter-spacing": 0.05,10 ...11 }12 ...13}
List of supported restrictions
Restriction | Description |
---|---|
max_weight | Max gross (total) weight. float |
max_axle_weight | Max weight per axle. float |
max_height | Maximum height allowed. float |
max_axle_count | Maximum number of axles. integer |
no_entry | No entry of a specified vehicle type. |
max_width | Maximum width allowed. float |
max_length | Maximum length allowed. float |
speed_limit | Maximum speed allowed. integer |
hazmat_restriction | Type of general hazmat restriction. See: General load type mapping section integer |
hazmat_class_explosives | Hazmat restriction with explosives dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_gases | Hazmat restriction with the gases dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_flammable_liquids | Hazmat restriction with the flammable liquids dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_flammable_solids | Hazmat restriction with the flammable solids dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_oxidizing_and_organic | Hazmat restriction with the oxidizing and organic dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_toxic_and_infectious | Hazmat restriction with the toxic and infectious dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_radioactive | Hazmat restriction with the radioactive dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_corrosives | Hazmat restriction with the corrosives dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
hazmat_class_miscellaneous | Hazmat restriction with the miscellaneous dangerous goods class. See: Vehicle dangerous goods load type mapping section integer |
adr_restriction_category | Vehicle ADR category. See: Vehicle ADR category type mapping section string |
no_commercial_vehicle | Commercial vehicles are not allowed. |
List of supported units
Value | Description |
---|---|
0 | Kilometer per hour |
1 | Gallon |
2 | Percentage |
3 | Mile per hour |
4 | Metric ton |
5 | Centimeter |
6 | Meter |
7 | Liter |
8 | Short Ton |
9 | Inch |
10 | Foot |
General load type mapping
Value | General load type |
---|---|
0 | General_Hazardous_Materials |
1 | Explosive_Materials |
2 | Goods_Harmful_To_Water |
Dangerous goods load type mapping
Value | Dangerous goods load type |
---|---|
0 | Explosives |
1 | Gases |
2 | Flammable_Liquids |
3 | Flammable_Solids |
4 | Oxidizing_And_Organic_Substance |
5 | Toxic_And_Infectious_Substance |
6 | Radioactive_Material |
7 | Corrosives |
8 | Miscellaneous_Dangerous_Goods |
Adr category type mapping
Value | ADR category |
---|---|
B | ADR category B |
C | ADR category C |
D | ADR category D |
E | ADR category E |