Content
TomTom Vector Format
TomTom Vector tiles contain map features (points, lines, road shapes, water polygons, building footprints, etc.) that can be used to visualize map data. The data is divided into multiple protobuf layers. Besides the layers, the protobuf tags are also used to further describe the geographic features.
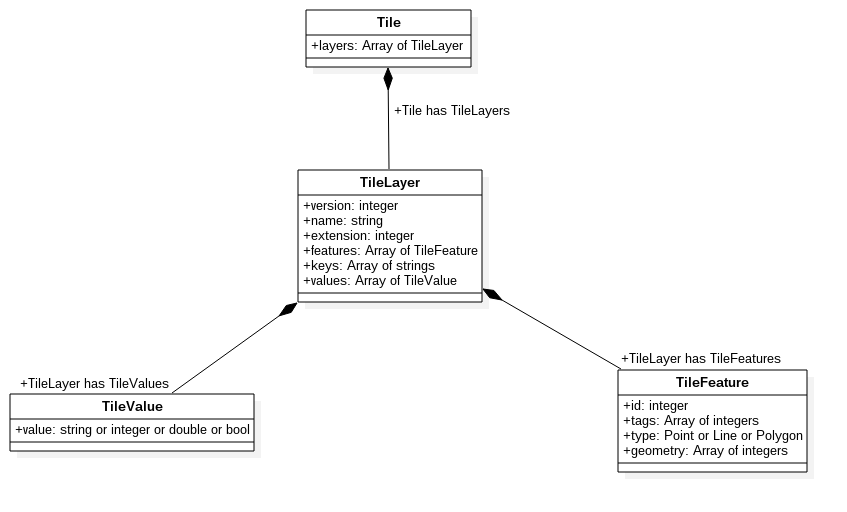
Legend
Tile
Represents a vector tile as a whole entity. It consists of at least one
TileLayer
.
If the tile does not contain any relevant data, it contains one layer namedempty
.TileLayer
Part of a
Tile
, representing one of the layers that can be rendered later. One layer consists of e.g., all Parks, Roads, Build up areas, or Points of interest.Each
TileLayer
contains geometries of the same type. A geometry in the vector tile can be one of three types:POINT
,LINESTRING
, orPOLYGON
. EveryTileLayer
contains the following fields:name
version
extent
It may also contain the following fields:
keys
are an array of uniquestrings
.values
are an array of uniqueTileValues
.features
are an array of uniqueTileFeatures
.
Arrays of
keys
andvalues
contain the mapping for tags ofTileFeature.
TileFeature
Member of an array of
features
stored in aTileLayer
, containing a geometry representation that can be rendered.It always contains the following fields:
type
is a type of geometry. It can bepoint
,linestring
, orpolygon
.geometry
is an array of encoded geometry that can be used for rendering. See the Decoding tile geometry section for details.
It may also contain the following fields:
tags
is an array containing properties of feature.They are a
TileFeature's
detailed description which can be used for styling and later rendering the geometry.- They are encoded into an array of integers.
In order to decode them, the arrays of
keys
andvalues
from a correspondingTileLayer
must be used.See the Decoding feature tags section for details.
TileValue
Encoded representation of a string, integer, floating point, or boolean value.
It is stored in a
values
array, in aTileLayer
.It is used for decoding
tags
of aTileFeature
(together with the members of akeys
array).See the Decoding feature tags section for details.
TomTom Vector Schema
Schema data
Formally, the structure of vector tile is described by the protocol buffer schema. It allows the generation of C++, Java, Python, Go, Ruby, Objective-C, and C# code based on proto file.
1package vector_tile23option optimize_for = LITE_RUNTIME;45message Tile {67 enum GeomType {8 UNKNOWN = 0;9 POINT = 1;10 LINESTRING = 2;11 POLYGON = 3;12 }1314 message Value {15 optional string string_value = 1;16 optional float float_value = 2;17 optional double double_value = 3;18 optional int64 int_value = 4;19 optional uint64 uint_value = 5;20 optional sint64 sint_value = 6;21 optional bool bool_value = 7;22 extensions 8 to max;23 }2425 message Feature {26 optional uint64 id = 1 [ default = 0 ];27 repeated uint32 tags = 2 [ packed = true ];28 optional GeomType type = 3 [ default = UNKNOWN ];29 repeated uint32 geometry = 4 [ packed = true ];30 optional bytes raster = 5;31 }3233 message Layer {34 required uint32 version = 15 [ default = 1 ];35 required string name = 1;36 repeated Feature features = 2;37 repeated string keys = 3;38 repeated Value values = 4;39 optional uint32 extent = 5 [ default = 4096 ];4041 extensions 16 to max;42 }4344 repeated Layer layers = 3;4546 extensions 16 to 8191;47}
Decoding tile geometry
Vector Tile geometry uses the following coordinate system:
- Coordinates are always integers.
- The
(0,0)
point is located in the upper-left corner of the tile. - The X axis has values increasing towards the right of the tile.
- The Y axis has values increasing towards the bottom of the tile.
- The tile may have margin, which is a buffer around the tile in the shape of a square frame. The size of the margin is by default equal to 10% of the width/length of the tile.
- The extent is equal to
4096
, so the value range for X and Y coordinates is from0
to4095
for visible points. - If the tile has a margin, the coordinates values range is extended by its size in both directions. This may cause coordinate values for points in the left or upper margin to be negative.
Vector Tile geometry is encoded as an array of 32 bit unsigned integers in the geometry
field of the TileFeature
.
Encoded format has the following structure:
[command_and_count][x0][y0]..[xn][yn][command_and_count][x0][y0]..
command_and_count
contains encoded values ofcommand
andcount
.[x0][y0]..[xn][yn]
are(x,y)
coordinate pairs.
Command
The value of command
is decoded as follows:
command = command_and_count & 0x7
Commands are to be executed relative to previous path coordinates or the point (0,0)
if it is the first command.
Command type | Value | Coordinates | Description |
---|---|---|---|
|
|
| It defines the
|
|
|
| It defines a new segment, starting at the current position of the cursor
and ending at the
|
|
| none | It closes the current linear ring of a |
Count
The value of count
is decoded as follows: count = command_and_count >> 0x3
- It defines the
n
number of[xn][yn]
encoded coordinate pairs following thecommand_and_count
value. - These coordinate pairs must be interpreted according to the preceding
command
type.
Coordinates
Coordinates [x0][y0]..[xn][yn]
are encoded in zigzag encoding and are relative to the previous coordinate pair. This means that only the first coordinate pair [x0][y0]
in the first command in every TileFeature
stores absolute values.
The coordinates are decoded as follows:
1decode(x0) = ((x0 >> 0x1) ^ (-(x0 & 0x1)))2decode(y0) = ((y0 >> 0x1) ^ (-(y0 & 0x1)))34decode(x1) = decode(x0) + ((x1 >> 0x1) ^ (-(x1 & 0x1)))5decode(y1) = decode(y0) + ((y1 >> 0x1) ^ (-(y1 & 0x1)))67...89decode(xn) = decode(xn-1) + ((xn >> 0x1) ^ (-(xn & 0x1)))10decode(yn) = decode(yn-1) + ((yn >> 0x1) ^ (-(yn & 0x1)))
Examples
For a POINT
type feature
1layer: 02 feature: 03 type: POINT4 geometry: [9, 1136, 6564]
1geometry:2 command_and_count = 93 command = 9 & 0x7 = 14 count = 9 >> 0x3 = 156 x0 = 1136, y0 = 65647 decode(x0) = ((1136 >> 0x1) ^ (-(1136 & 0x1))) = 5688 decode(y0) = ((6564 >> 0x1) ^ (-(6564 & 0x1))) = 3282
1layer: 02 feature: 03 geometry: POINT(561, 3282)
For a LINESTRING
type feature
1layer: 02 feature: 03 type: LINESTRING4 geometry: [9, 846, 2312, 10, 652, 1938]
1geometry:2 1:3 command_and_count = 94 command = 9 & 0x7 = 15 count = 9 >> 0x3 = 167 x0 = 846, y0 = 23128 decode(x0) = ((846 >> 0x1) ^ (-(846 & 0x1))) = 4239 decode(y0) = ((2312 >> 0x1) ^ (-(2312 & 0x1))) = 115610 2:11 command_and_count = 1012 command = 10 & 0x7 = 213 count = 10 >> 0x3 = 11415 x0 = 652, y0 = 193816 decode(x0) = decode(1:x0) + ((652 >> 0x1) ^ (-(652 & 0x1))) = 423 + 326 = 74917 decode(y0) = decode(1:y0) + ((1938 >> 0x1) ^ (-(1938 & 0x1))) = 1156 + 969 = 2125
1layer: 02 feature: 03 geometry: LINESTRING[(423, 1156), (749, 2125)]
For a POLYGON
type feature
1layer: 02 feature: 03 type: POLYGON4 geometry: [9, 1320, 5622, 26, 416, 707, 68, 612, 483, 96, 7]
1geometry:2 1:3 command_and_count = 94 command = 9 & 0x7 = 15 count = 9 >> 0x3 = 167 x0 = 1320, y0 = 56228 decode(x0) = ((1320 >> 0x1) ^ (-(1320 & 0x1))) = 6609 decode(y0) = ((5622 >> 0x1) ^ (-(5622 & 0x1))) = 281110 2:11 command_and_count = 2612 command = 26 & 0x7 = 213 count = 26 >> 0x3 = 31415 x0 = 1736, y0 = 491416 decode(x0) = decode(1:x0) + ((416 >> 0x1) ^ (-(416 & 0x1))) = 660 + 208 = 86817 decode(y0) = decode(1:y0) + ((707 >> 0x1) ^ (-(707 & 0x1))) = 2811 + (-354) = 24571819 x1 = 68, y1 = 61220 decode(x1) = decode(x0) + ((68 >> 0x1) ^ (-(68 & 0x1))) = 90221 decode(y1) = decode(y0) + ((612 >> 0x1) ^ (-(612 & 0x1))) = 27632223 x2 = 483, y2 = 9624 decode(x2) = decode(x1) + ((483 >> 0x1) ^ (-(483 & 0x1))) = 66025 decode(y2) = decode(y1) + ((96 >> 0x1) ^ (-(96 & 0x1))) = 281126 3:27 command_and_count = 728 command = 7 & 0x7 = 729 count = 7 >> 0x3 = 0
1layer: 02 feature: 03 geometry: POLYGON[(660, 2811), (868, 2457), (902, 2763), (660, 2811)]
Decoding feature tags
In order to reduce tile size, the properties of each feature are encoded in a tags
array. The tags
array contains integer values which are indexes of keys
and values
arrays belonging to the corresponding layer. The size of a tags
array is always even.
The content of a tags
array can be grouped into a list of pairs. Each odd element of an array is the first element of a pair, and each even element of an array is the second element of a pair.
- The first element of each pair should be mapped to a
keys
array. - The second element of each pair should be mapped to a
values
array.
As a result, we get a decoded list of tags
where the first element is the tag name and the second element is the tag value in the form of TileValue
.
Example
Below is an example of what an encoded tile may look like.
1layer: 02 keys: ["country_code", "icon_text"],3 values: ["SWE", "E4"]4 feature: 05 tags : [0,0,1,1]
After decoding it has to be read like this:
1layer: 02 feature: 03 properties:4 country_code : "SWE"5 icon_text : "E4"
Tile Layers
The data in TomTom Vector Tiles is organized in layers that can be arranged into groups, consisting of layers that describe similar map features. The following section provides detailed information about:
- All groups.
- Layers that are contained in each group.
- Tags that typically appear in features from a given group.
Landuse
The landuse
layers include polygons that represent land use and land cover.
- Group name:
landuse
- Geometry type:
polygon
Landuse layers
Built-up area | National or state park | City park | Woodland |
---|---|---|---|
Regional park | National park | State or province park | County park |
Cemetery | Moor or heathland | Beach or dune | Zoo |
Industrial harbor area | Industrial area | Stadium | Hospital |
Shopping | Amusement park | University | School |
Golf | Parking area | Sports hall | Institution |
Airport | Runway | Military | Sand |
Forest | Pedestrian Deck | Town shore | Town garden path |
Town swimming pool | Town paved area | Town walkway | Town carriageway divider |
Town factory ground | Town school ground | Town hospital ground | Town railway ground |
Town greens | Town grass | Town water body | Meridian |
Sidewalk | Breakwater | Pool | Park |
This layer group does not use any additional tags.
Earth Cover
The Earth Cover layers include polygons that represent the dominant physical cover of the earth's surface from a top-down perspective.
- Group name:
earth_cover
- Geometry type:
polygon
Earth Cover layer
Earth Cover | - | - | - |
Earth Cover tags
Tag | Description |
---|---|
string | This tag provides a distinction between different types of earth cover. Allowed values:
|
Water
The water layers include polygons that represent water bodies like ocean, seas, rivers, lakes, and other water bodies.
- Group name:
water
- Geometry type:
polygon
Water layers | |||
---|---|---|---|
Other water | Intermittent water | River | Lake |
Sea 1 | Ocean 1 | Ocean or sea | - |
1 Layer for future use. Not supported yet. |
This layer group does not use any additional tags.
Buildings
The building layers represent footprints of buildings.
- Group name:
buildings
- Geometry type:
polygon
Buildings layers | |||
---|---|---|---|
Subway Station | Factory building | Place of worship | Hospital building |
School building | Hotel building | Cultural Facility | Railway Station |
Government Administration Office | Other building | Other town block | - |
Buildings tags | |
---|---|
Tag | Description |
float | The height of the building in meters. |
Places
The places layers includes points that can be used for labeling places like countries, states, cities, towns, and villages.
- Group name:
places
- Geometry type:
point
Places layers | |||
---|---|---|---|
Capital city | Large city | Medium city | Small city |
Town | Village | Country name | State name |
State name short | - | - | - |
Places tags | |
---|---|
Tag | Description |
integer | The tag value represents a priority of the city which is based on the combination of the population of the city and its administrative importance. The lower the value of this tag the more important the city is. Not applicable for layers: Country name , State name , or State name short. |
integer | The tag presence indicates that the point representing a city center is
a state capital. The value of this tag is always |
string | This tag provides a distinction between different types of settlements. Allowed values:
|
POI
The POI group consists of layers that represent points of interest. Depending on the type of tile layer in a request, (see Tiles Layers and Styles different layers will be present in the protobuf tile.
- Group name:
poi
- Geometry type:
point
POI layers (basic, hybrid, and labels vector tiles) | |||
---|---|---|---|
Airport POI | Railway station | National park name | Ferry terminal |
POI | Point of Interest | - | - |
POI layers (basic, hybrid, and labels vector tiles) | |||
---|---|---|---|
Point of Interest | - | - | - |
POI tags | |
---|---|
Tag | Description |
string | A Point of Interest name in a language provided as a query parameter to the request. For more information about languages in the Map Display API, please see the list of supported languages. |
string | The tag value represents a brand name of a facility or accommodation. |
string | A unique Point of Interest identifier that can be used across other TomTom services. |
string | This tag groups Points of Interest into broad categories that can be used for styling purposes. Allowed values depend on the tile layer in a request. For values available in basic, hybrid, and labels vector tiles, see Point of Interest Categories: basic, hybrid, and labels vector tiles. For values available in POI vector tiles, see Point of Interest Categories: poi tiles. |
string | This tag narrows down the category values allowing fine grained styling and providing distinction between Points of Interest in the same category. Allowed values depend on the tile layer in a request. For values available in basic, hybrid, and labels vector tiles, see Point of Interest Categories: basic, hybrid, and labels vector tiles. For values available in POI vector tiles, see Point of Interest Categories: poi tiles. |
integer | An identifier representing category. Either 4 digits integer for Points of Interest without subcategory or 7 digits integer for Points of Interest with subcategory (where the first 4 digits identify a category). It can be used across other TomTom services.
|
integer | The tag value represents a priority of the Point of Interest. The lower the value of this tag the more important the Point of Interest is. |
string | The name of the icon asset in the TomTom styles that should be used for the visualization purpose of the Point of Interest. |
Point of Interest Categories: basic, hybrid, and labels vector tiles
The Category ID and Subcategory ID in the following table correspond to the ID in poiCategories in the Search API.
Category ID | Category | Possible subcategories | Subcategory ID |
---|---|---|---|
9383 | Industrial Building | - | - |
7377 | College/University | - | - |
7392 | Fire Station/Brigade | - | - |
7367 | Government Office | - | - |
9913 | Library | - | - |
9388 | Military Installation | - | - |
9389 | Native Reservation | - | - |
7322 | Police Station | - | - |
7324 | Post Office | - | - |
9154 | Prison/Correctional Facility | - | - |
7372 | School | - | - |
7321 | Hospital | - | - |
9902 | Amusement Park | - | - |
7317 | Museum | - | - |
9362 | Park & Recreation Area | National Park Cemetery | 9362008 9362003 |
9927 | Zoo, Arboreta & Botanical Garden | - | - |
7373 | Shopping Center | - | - |
9911 | Golf Course | - | - |
7374 | Stadium | - | - |
7376 | Tourist Attraction | - | - |
7339 | Place of Worship | Church Mosque Synagogue Temple Gurudwara Ashram Pagoda | 7339002 7339003 7339004 7339005 7339006 7339007 7339008 |
7383 | Airport | - | - |
7352 | Ferry Terminal | - | - |
9942 | Public Transportation Stop | Bus Stop Taxi Stand Coach Stop Streetcar Stop | 9942002 9942003 9942005 9942004 |
7380 | Railroad Station | International Railroad Station National Railroad Station Subway Station | 738002 738003 738005 |
8099 | Geographic Feature | Mountain Peak Ridge Valley Reef Hill | 8099002 8099004 8099006 8099014 8099025 |
Point of Interest Categories: poi vector tiles
The Category ID and Subcategory ID in the following table correspond to the ID in poiCategories in the Search API.
Category ID | Category | Possible subcategories | Subcategory ID |
---|---|---|---|
9910 | Automotive Dealer | - | - |
9155 | Car Wash | - | - |
7309 | Electric Vehicle Station | - | - |
7368 | Motoring Organization Office | - | - |
7369 | Open Parking Area | - | - |
7313 | Parking Garage | - | - |
7311 | Gas Station | - | - |
7312 | Rent-a-Car Facility | - | - |
9930 | Rent-a-Car-Parking | - | - |
7310 | Repair Facility | - | - |
7395 | Rest Area | - | - |
7375 | Toll Gate | - | - |
7358 | Truck Stop | - | - |
7359 | Weigh Station | - | - |
7335 | Agriculture Business | - | - |
7328 | Bank | - | - |
7378 | Business Park | - | - |
7397 | ATM | - | - |
9382 | Commercial Building | - | - |
9352 | Company | - | - |
7388 | Courier Drop Box | - | - |
7391 | Emergency Medical Service | - | - |
9160 | Exchange | - | - |
9377 | Exhibition & Convention Center | - | - |
9383 | Industrial Building | - | - |
9156 | Manufacturing Facility | - | - |
9158 | Media Facility | - | - |
9157 | Research Facility | - | - |
9376 | Café/Pub | Café Pub Internet Café Tea House Coffee Shop Microbrewery | 9376002 9376003 9376004 9376005 9376006 9376007 |
7315 | Restaurant | - | - |
7377 | College/University | - | - |
9363 | Courthouse | - | - |
7365 | Embassy | - | - |
7392 | Fire Station/Brigade | - | - |
7367 | Government Office | - | - |
9913 | Library | - | - |
9388 | Military Installation | - | - |
9389 | Native Reservation | - | - |
9152 | Non Governmental Organization | - | - |
7322 | Police Station | - | - |
7324 | Post Office | - | - |
9150 | Primary Resource/Utility | - | - |
9154 | Prison/Correctional Facility | - | - |
9932 | Public Amenity | - | - |
7372 | School | - | - |
7301 | Traffic Service Center | - | - |
9151 | Transport Authority/Vehicle Registration | - | - |
9153 | Welfare Organization | - | - |
9374 | Dentist | - | - |
9373 | Doctor | - | - |
9956 | Emergency Room | - | - |
9663 | Health Care Service | - | - |
7321 | Hospital | - | - |
7326 | Pharmacy | - | - |
9375 | Veterinarian | - | - |
9902 | Amusement Park | - | - |
7341 | Casino | - | - |
7342 | Movie Theater | - | - |
9937 | Club & Association | - | - |
7363 | Community Center | - | - |
7319 | Cultural Center | - | - |
9900 | Entertainment | - | - |
9378 | Leisure Center | - | - |
7347 | Marina | - | - |
7317 | Museum | - | - |
9379 | Nightlife | - | - |
9362 | Park & Recreation Area | National Park Cemetery | 9362008 9362003 |
7318 | Theater | - | - |
7302 | Trail System | - | - |
7349 | Winery | - | - |
9927 | Zoo, Arboreta & Botanical Garden | - | - |
7360 | Camping Ground | - | - |
7304 | Vacation Rental | - | - |
7314 | Hotel/Motel | - | - |
7303 | Residential Accommodations | - | - |
7327 | Department Store | - | - |
7332 | Market | - | - |
9361 | Shop | - | - |
7373 | Shopping Center | - | - |
9911 | Golf Course | - | - |
9360 | Ice Skating Rink | - | - |
7320 | Sports Center | - | - |
7374 | Stadium | - | - |
7338 | Swimming Pool | - | - |
9369 | Tennis Court | - | - |
9371 | Water Sport | - | - |
7305 | Adventure Sports Venue | - | - |
9357 | Beach | - | - |
7376 | Tourist Attraction | - | - |
7339 | Place of Worship | Church Mosque Synagogue Temple Gurudwara Ashram Pagoda | 7339002 7339003 7339004 7339005 7339006 7339007 7339008 |
7337 | Scenic/Panoramic View | - | - |
7316 | Tourist Information Office | - | - |
7389 | Access Gateway | - | - |
7383 | Airport | - | - |
9955 | Checkpoint | - | - |
7352 | Ferry Terminal | - | - |
7366 | Frontier Crossing | - | - |
7308 | Helipad | - | - |
9935 | Mountain Pass | - | - |
9159 | Port/Warehouse Facility | - | - |
9942 | Public Transportation Stop | Bus Stop Taxi Stand Coach Stop Streetcar Stop | 9942002 9942003 9942005 9942004 |
7380 | Railroad Station | International Railroad Station National Railroad Station Subway Station | 738002 738003 738005 |
8099 | Geographic Feature | Mountain Peak Cave Ridge Dune Valley Plain/Flat Plateau Pan Well Oasis Rocks Reservoir Reef Rapids Bay Cove Harbor Lagoon Cape Mineral/Hot Springs Island Marsh River Crossing Hill Quarry Locale | 8099002 8099003 8099004 8099005 8099006 8099007 8099008 8099009 8099010 8099011 8099012 8099013 8099014 8099015 8099016 8099017 8099018 8099019 8099020 8099021 8099022 8099023 8099024 8099025 8099026 8099027 |
Cartolabels
The cartolabels layers include points that represent cartographic labels. Please note that most of the layers in this group are being replaced by Points of Interest with equivalent categories.
- Group name:
cartolabels
- Geometry type:
point
Cartolabels layers | |||
---|---|---|---|
Airport label | Amusement area label | Cemetery label | Park/Garden label |
Stadium label | Military Territory label | Golf Course label | Hospital label |
Industrial area label | Other water body label | Woodland label | Prison label |
University/School label | Intermittent water label | Island label | Lake label |
Zoo label | Reservation label | Shopping centre label | Landmark label |
River label | Ocean label | Sea label | National park label |
Other label | Museum label | - | - |
Cartolabels tags | |
---|---|
Tag | Description |
string | Label name in a language provided as a query parameter to the request. For more information about languages in the Map Display API, please see the list of supported languages. |
Cartolabels layers | |||
---|---|---|---|
Airport label | Amusement area label | Cemetery label | Park/Garden label |
Stadium label | Military Territory label | Golf Course label | Hospital label |
Industrial area label | Other water body label | Woodland label | Prison label |
University/School label | Intermittent water label | Island label | Lake label |
Zoo label | Reservation label | Shopping centre label | Landmark label |
River label | Ocean label | Sea label | National park label |
Other label | Museum label | - | - |
Cartolabels tags | |
---|---|
Tag | Description |
string | Label name in a language provided as a query parameter to the request. For more information about languages in the Map Display API, please see the list of supported languages. |
Roads
The roads layers include lines that can be used for drawing roads.
- Group name:
cartolabels
- Geometry type:
line
Roads layers | |||
---|---|---|---|
Motorway | International road | Major road | Secondary road |
Connecting road | Major local road | Local road | Minor local road |
Motorway tunnel | International road tunnel | Major road tunnel | Secondary road tunnel |
Connecting road tunnel | Major local road tunnel | Local road tunnel | Minor local road tunnel |
Toll motorway | Toll international road | Toll major road | Toll secondary road |
Toll connecting road | Toll major local road | Toll local road | Toll minor local road |
Toll motorway tunnel | Toll international road tunnel | Toll major road tunnel | Toll secondary road tunnel |
Toll connecting road tunnel | Toll major local road tunnel | Toll local road tunnel | Toll minor local road tunnel |
Ferry road | Pedestrian road | Non public road | Walkway road |
Each road may have one or more road shields. The shields are indexed from 0, and described by sets of tags.
Roads tags | |
---|---|
Tag | Description |
string | Label name in a language provided as a query parameter to the request. For more information about languages in the Map Display API, please see the list of supported languages. |
string | Icon id used in older styles. Currently replaced by the shield_icon_{idx} tag. |
string | The name of the road shield icon associated with the road. Value: For easier referencing and implementation, the name of a roadshield follows one of two formats:
See the Shield icon shape types section for existing shape types. See the Shield icon fill and stroke colors section for existing shield colors. See the Shield icon countries and states section for details on country names and state codes. numOfChars is a number in the range |
string | Text to be placed on the road shield icon with the corresponding shield_icon idx. Value: road number |
string | The color of the text on the road shield icon with the corresponding shield_icon idx. Value: hexadecimal color code |
string | The color of the text on the road shield icon in night mode with the corresponding shield_icon idx. Value: hexadecimal color code |
boolean | The tag presence indicates that the road is under construction. The
value of this tag is always |
integer | The tag presence indicates that the road is a one way road. The value of
this tag is Note: This tag is currently sent from zoom level 15 inclusive up to zoom level 22. |
string | A 3 character ISO code of a country to which a given road belongs. |
string | The value of the tag indicates a portion of a country or other region delineated for the purpose of administration such as State, District, Region, or Province Codes. |
integer | The integer value used to determine the drawing order of overlapping
road segments. The values are in the range Note: This tag is currently sent from zoom level 13 inclusive up to zoom level 22. |
Shield icon shape types
rectangle | hexagon | hexagon2 | octagon | special01 | special02 | special03 | special04 |
shield01 | shield02 | shield03 | shield04 | shield05 | shield06 | shield07 | shield08 |
shield09 | shield10 | shield11 | shield12 | pentagon | circle | pill | us1 |
us2 | us3 | oval | diamond |
Shield icon fill and stroke colors
white | yellow1 | yellow2 | yellow3 | orange | green1 | green2 | green3 | blue1 |
blue2 | blue3 | brown1 | brown2 | red | grey | anthracite | black |
Shield icon countries and states
Possible values of the countryName part of a name are listed in the following table.
- For Canada shields, the stateCode part of the shield's name is either a two-letter province name abbreviation or empty.
- For USA shields, the stateCode may be a two-letter ISO-3166 state name abbreviation,
highway
,county
, or empty. - For other country shields the stateCode is empty.
colombia | south | mexico | indonesia | taiwan | canada | usa | venezuela | southafrica |
cuba | india | australia | argentina | saudiarabia | uae | peru | korea |
Transit
The transit layers include lines that can be used to draw transit lines.
- Group name:
transit
- Geometry type:
line
Transit layers | |||
---|---|---|---|
Railway | - | - | - |
This layer group does not use any additional tags.
Boundaries
The boundaries layers include lines that can be used to draw different types of boundaries.
- Group name:
boundaries
- Geometry type:
line
Boundaries layers | |||
---|---|---|---|
Country border | Disputed country border | Treaty country border | State border |
This layer group does not use any additional tags.