Planning a route
Requesting routes
To calculate a route from A to B, you need to provide route planning criteria. Routes are built using the RoutePlanningOptions
class. You can take advantage of the named parameters in Kotlin to choose the properties that you need. The only required parameter is an itinerary
. There are multiple optional parameters that you can use to shape the route planning criteria to fit your use cases. For a detailed description of available parameters, see the Routing API documentation.
1val amsterdam = GeoPoint(52.377956, 4.897070)2val rotterdam = GeoPoint(51.926517, 4.462456)3val routePlanningOptions = RoutePlanningOptions(4 itinerary = Itinerary(origin = amsterdam, destination = rotterdam),5 costModel = CostModel(routeType = RouteType.Efficient),6 vehicle = Vehicle.Truck(),7 alternativeRoutesOptions = AlternativeRoutesOptions(maxAlternatives = 2),8)
Once you have a RoutePlanningOptions
object, provide it to the planRoute
method. This can be done asynchronously using the RoutePlanningCallback
, or synchronously as described in the Synchronous routing call. If the route planning is successful, the onSuccess(result: RoutePlanningResponse)
method is called. If an error occurred, it appears in the RoutingFailure
.
1routePlanner.planRoute(2 routePlanningOptions,3 object : RoutePlanningCallback {4 override fun onSuccess(result: RoutePlanningResponse) {5 // YOUR CODE GOES HERE6 }78 override fun onFailure(failure: RoutingFailure) {9 // YOUR CODE GOES HERE10 }1112 override fun onRoutePlanned(route: Route) {13 // YOUR CODE GOES HERE14 }15 },16)
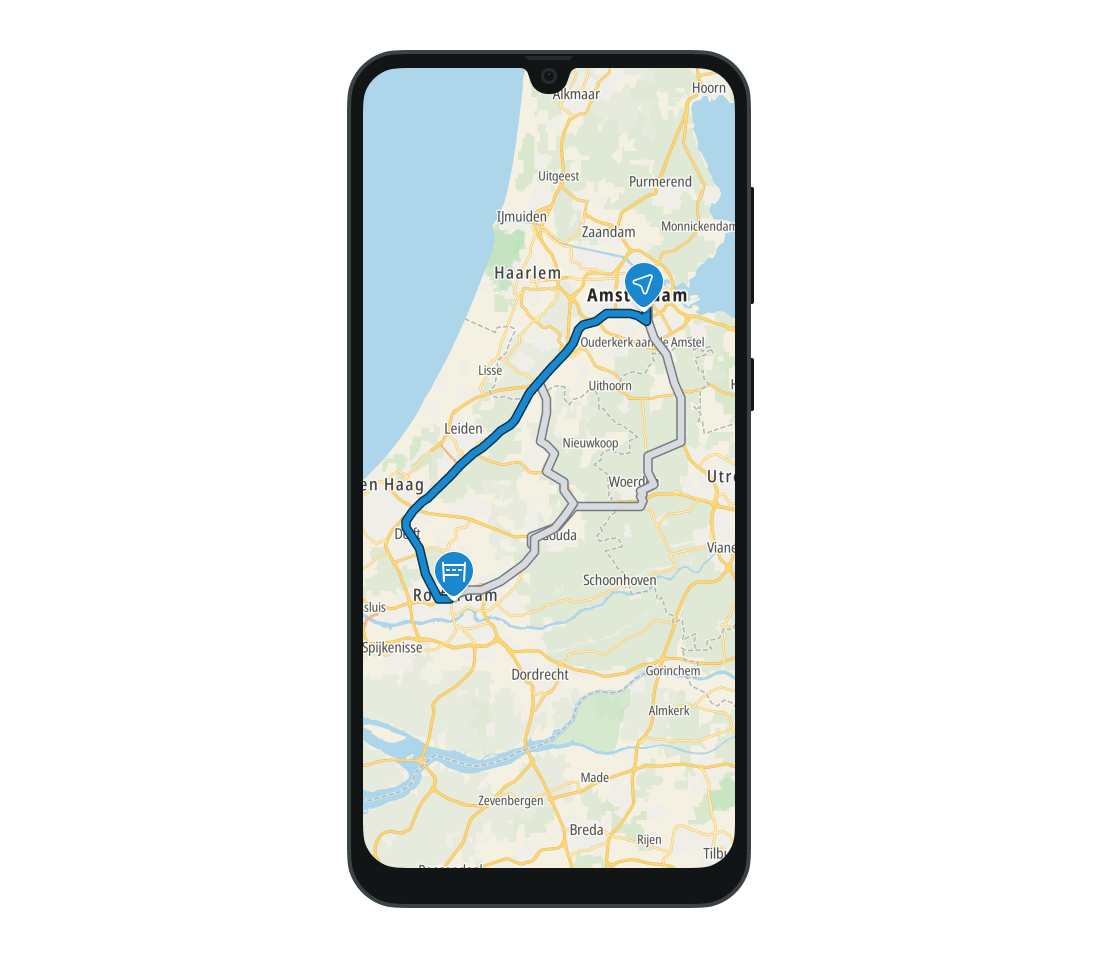
Adjusting route planning criteria
Route types
The route type parameter specifies the type of optimization used when calculating routes:
Fast
: Route calculation is optimized by travel time, while keeping the routes sensible. For example, the calculation may avoid shortcuts along inconvenient side roads or long detours that only save very little time.Short
: Route calculation is optimized by travel distance, while keeping the routes sensible. For example, straight routes are preferred over those incurring turns.Efficient
: Route calculation is optimized to achieve a good compromise between shorter travel time and lower fuel or energy consumption.Thrilling
: Route calculation is optimized so that routes include interesting or challenging roads and use as few motorways as possible.- You can choose the level of turns included and also the degree of hilliness. See the hilliness and windingness parameters to set this.
- There is a limit of 900km on routes planned with thrilling route type.
Default value is set to Fast
.
Avoids
The RoutePlanningOptions.costModel.avoidOptions.avoidTypes
parameter specifies something that the route calculation should try to avoid when determining the route. The avoid can be specified multiple times. Avoids are honored whenever there is a reasonable alternative available that fulfills all avoid criteria. If the detour becomes too large, some avoid criteria can be violated on parts of the route. The violations can be detected by checking the corresponding route sections.
Possible avoids and their corresponding sections are:
AvoidType.TollRoads
: Avoids toll roads (section:SectionType.TollRoad
).AvoidType.Motorways
: Avoids motorways (section:SectionType.Motorway
).AvoidType.Ferries
: Avoids ferries (section:SectionType.Ferry
).AvoidType.UnpavedRoads
: Avoids unpaved roads (section:SectionType.Unpaved
).AvoidType.CarPools
: Avoids routes that require the use of carpool (HOV/High-Occupancy Vehicle) lanes (section:SectionType.Carpool
).AvoidType.AlreadyUsedRoads
:- Avoids using the same road multiple times. This is important for round trips, when you do not want to use the same roads back.
- This is most useful in conjunction with
RouteType.Thrilling
. - There is no corresponding section.
AvoidType.BorderCrossings
: Avoids crossing country borders (section:SectionType.Country
).AvoidType.Tunnels
: Avoids tunnels (section:SectionType.Tunnel
).AvoidType.CarTrains
: Avoids car trains (section:SectionType.CarTrain
).AvoidType.LowEmissionZones
: Avoids low-emission zones (section:SectionType.LowEmissionZone
).
Vehicle
The Vehicle contains parameters relevant for selecting suitable routes. It also provides information about the current state, e.g., the level of fuel. Some roads in the map have vehicle and time dependent restrictions. For example, roads may restrict traffic to pedestrians, or can only be used by electric vehicles. Roads may prohibit vehicles carrying hazardous materials. Tunnels may only be passable by vehicles up to a maximum height, and for trucks with the proper tunnel code.
Vehicles are distinguished by type. Each vehicle type has a different constructor which takes exactly those parameters that are useful for that type. For example, dimensions are only meaningful for motorized vehicles, and not for bikes and pedestrians.
Vehicles have the following properties:
- A type, e.g. car, motorcycle, truck.
- A maximum speed.
- Motorized vehicles can have an engine, either combustion or electric or both for a hybrid vehicle.
- Pedestrians and bicycles do not support engines.
- Each engine can contain consumption parameters used to predict the vehicle range.
- Motorized vehicles may have dimensions.
- They can be commercial transports.
- Trucks may have a load for which restrictions apply:
- A hazmat classification of the load can restrict usable roads.
- An ADR tunnel code describes which tunnels can be used.
Some vehicle properties are valid only at the current point in time and get updated over time, e.g. the fuel amount/charge level. Vehicle data is also useful during free driving mode without a route, e.g. for range features like 360 range around your current position.
Planning failures
A RoutingFailure
is returned if any failure occurred during the Routing API call. There are a few `RoutingFailure`s that are returned in different situations.
ApiKeyFailure
- Routing call did not finish due to a problem with the API key.BadInputFailure
- Some input parameter combination was not valid.ComputationTimeoutFailure
- Indicates that the request reached an internal computation time threshold and timed out.DeserializationFailure
- Deserialization failed.HttpFailure
- Routing call ended with unexpected HTTP code.InstructionGenerationFailure
- Instruction generation failed.InternalFailure
- The internal routing failure.MapMatchingFailure
- One of the input points (Origin, Destination, Waypoints) could not be matched to the map because there is no known drivable section near this point.NetworkFailure
- Routing network call failed with [IOException].NoRouteFoundFailure
- No valid route could be found.RouteReconstructionFailure
- The route reconstruction using supportingPoints failed.RoutePlanningCancelled
- The calculation was cancelled.UnknownFailure
- Routing call ended with unknown failure.
Incremental guidance computation
Incremental guidance computation is an optimization technique that reduces route planning time. It works by initially providing the route with limited number of instructions and lane guidance rather than compute all instructions and lane guidance for the entire route.
The mode for guidance computation is controlled by RouteInformationMode
. It contains two cases: Complete
and FirstIncrement
.
- The
Complete
case is set by default. In this mode theplanRoute
provides all instructions for the route immediately. - In the
FirstIncrement
case, the route is generated with the first guidance increment. This takes less time than usingComplete
mode.
1val amsterdam = GeoPoint(52.377956, 4.897070)2val rotterdam = GeoPoint(51.926517, 4.462456)3val routePlanningOptions = RoutePlanningOptions(4 itinerary = Itinerary(amsterdam, rotterdam),5 mode = RouteInformationMode.FirstIncrement,6)7val result = routePlanner.planRoute(routePlanningOptions)
To get next increment, the increment method advanceGuidanceProgress
is used.
1val route = result.routes.first()2val routeIncrementOptions = RouteIncrementOptions(3 route,4 planningOptions = routePlanningOptions,5)6val result = routePlanner.advanceGuidanceProgress(routeIncrementOptions)
The advanceGuidanceProgress
method is different from planRoute
in the following ways:
planRoute
creates a new route from the options passed as arguments. The computation results in either a route or an error.advanceGuidanceProgress
does not create a route. Instead, it works with an existing route, applying the planning options as it extends that route. This method returns either an updated version of existing route with new instructions and an updatedguidanceProgressOffset
value, or an error if the increment operation failed.
Next steps
Since you have learned how to plan a route, here are recommendations for the next steps: