Overlays
The TomTom Map Display module allows you to draw different shapes on the map.
Circles
You can draw a circle on the map by specifying:
- Coordinates for the center.
- Radius of the circle.
- Circle color.
1val fillColor = Color.argb(0.5f, 0.99f, 0.93f, 0.83f)2val outlineColor = Color.argb(0.6f, 0.93f, 0.62f, 0f)3val circleOptions =4 CircleOptions(5 GeoPoint(52.367956, 4.897070),6 radius = Radius(3000.0, RadiusUnit.Meters),7 fillColor = fillColor,8 outlineColor = outlineColor,9 outlineRadius = 100.0,10 )11val circle = tomTomMap.addCircle(circleOptions)
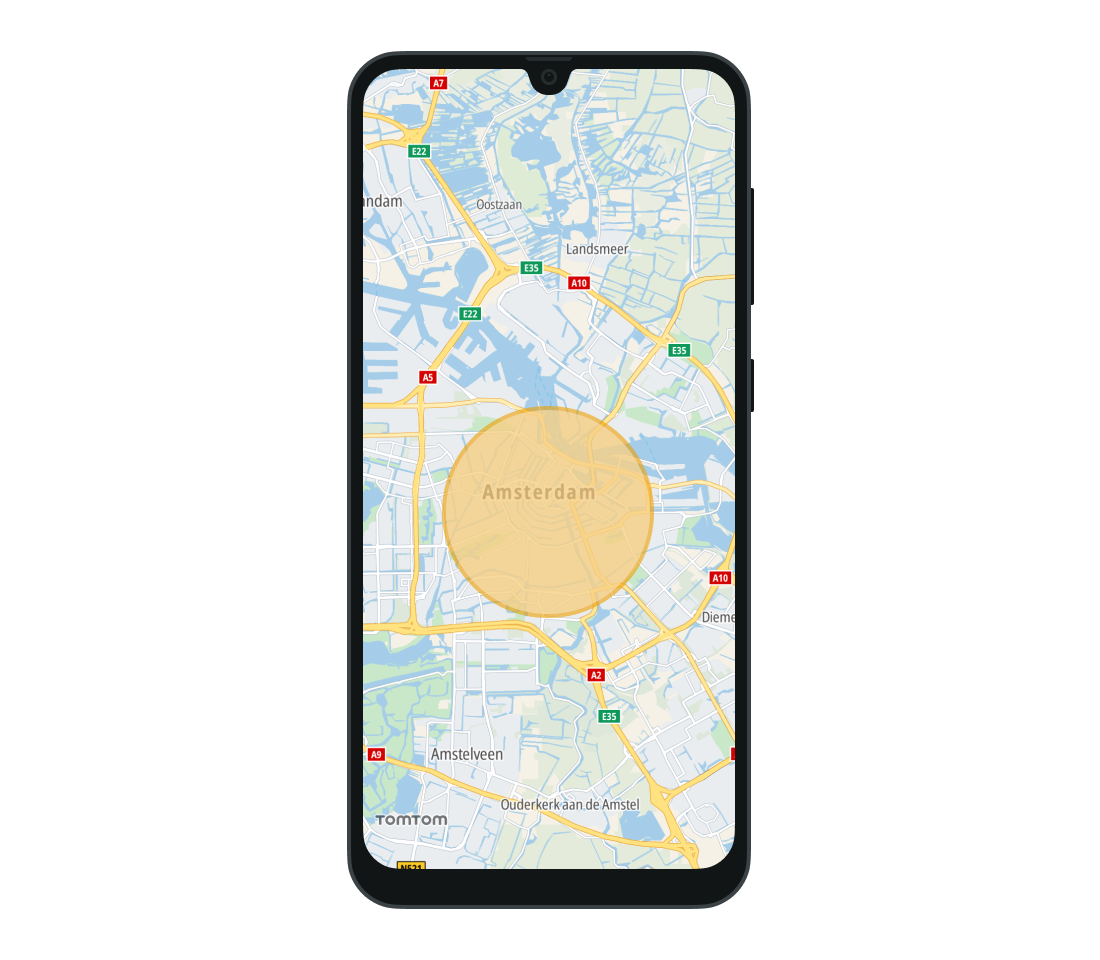
The TomTomMap.addCircle(CircleOptions)
method returns a Circle
object. Use it to update the circle’s properties, or call its Circle.remove()
method to remove it from the map.
To remove circles with a specific tag from the map, call its TomTomMap.removeCircles(String)
method.
tomTomMap.removeCircles()
Use the CircleClickListener
to listen for click events performed on any circle added to the map. Add the listener to the TomTomMap
object. Then whenever a Circle
is clicked, the CircleClickListener.onCircleClicked(Circle)
method is called with that specific Circle
instance as a parameter.
1val circleClickListener =2 CircleClickListener { circle: Circle -> /* YOUR CODE GOES HERE */ }3tomTomMap.addCircleClickListener(circleClickListener)
The CircleClickListener
can be removed when it is no longer needed.
tomTomMap.removeCircleClickListener(circleClickListener)
Polygons
The Map Display module also supports drawing polygons on the map. Drawing a polygon requires at least 3 vertices to be specified. The coordinates of a polygon are provided as a list of GeoPoint
objects, listed in counter-clockwise order.
In addition to defining the shape of the figure, you can specify its fill color and display an image inside it. To preserve the size of the original image, set the isImageOverlay
property to false. If the image is smaller than the polygon, it will be tiled. To scale the image to cover the whole polygon, set it to true.
1val fillColor = Color.argb(0.5F, 0.0F, 0.0F, 1.0F)2val polygonOptions =3 PolygonOptions(4 listOf(5 GeoPoint(latitude = 52.33744437330409, longitude = 4.84036333215833),6 GeoPoint(latitude = 52.3374581784774, longitude = 4.88185047814447),7 GeoPoint(latitude = 52.32935816673911, longitude = 4.910078096170823),8 GeoPoint(latitude = 52.381705486736315, longitude = 4.893630047460435),9 GeoPoint(latitude = 52.385294680380866, longitude = 4.846939597146335),10 ),11 outlineColor = Color.BLUE,12 outlineWidth = 2.0,13 fillColor = fillColor,14 )15val polygon = tomTomMap.addPolygon(polygonOptions)
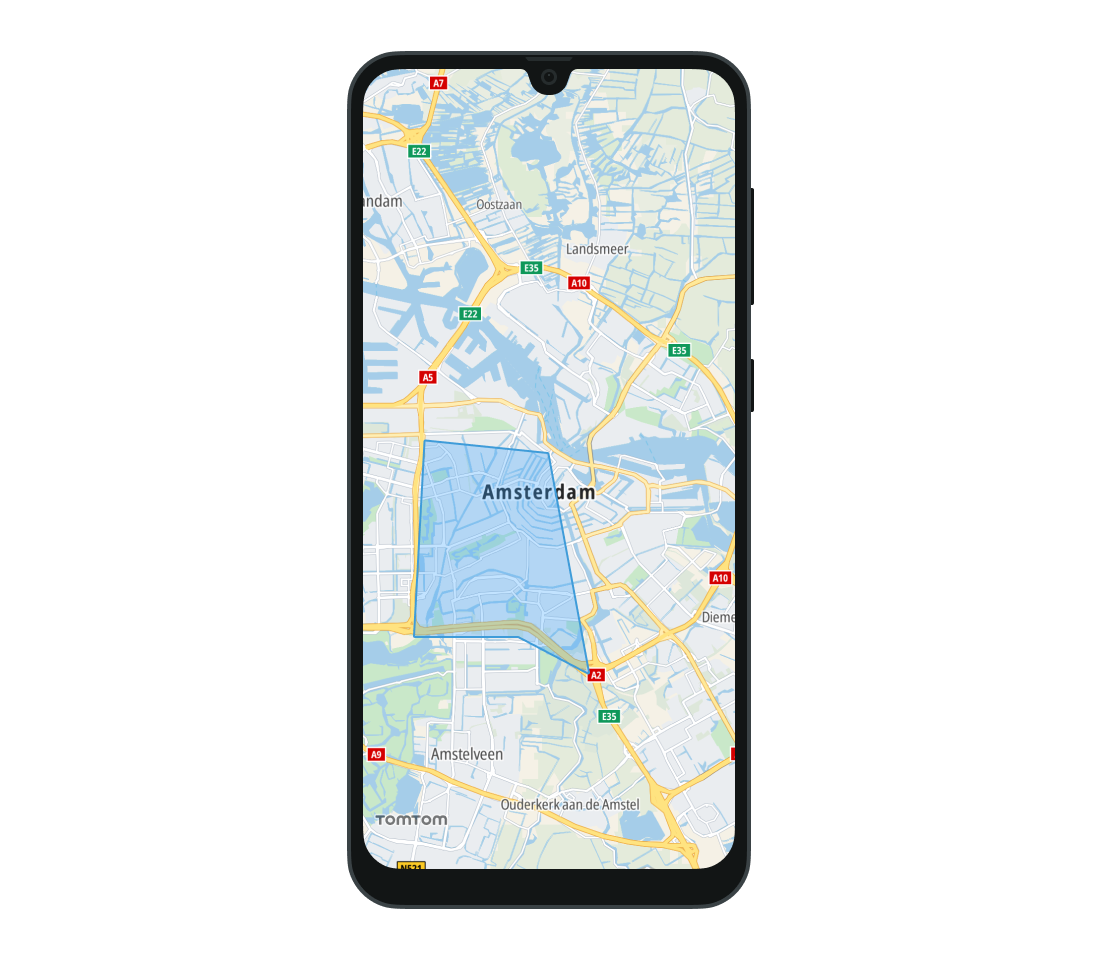
The TomTomMap.addPolygon(PolygonOptions)
method returns a Polygon
object. Use it to modify the shape’s properties, or call its Polygon.remove()
method to remove it from the map. To remove polygons with a specific tag from the map, use the TomTomMap.removePolygons(String)
method.
Use the PolygonClickListener
to listen for click events performed on any polygon added to the map. Add the listener to the TomTomMap
object. Then whenever a Polygon
is clicked, the PolygonClickListener.onPolygonClicked(Polygon)
method is called with that specific Polygon
instance as a parameter.
1val polygonClickListener =2 PolygonClickListener { polygon: Polygon -> /* YOUR CODE GOES HERE */ }3tomTomMap.addPolygonClickListener(polygonClickListener)
Remove the listener when you no longer need to observe click events on polygons.
tomTomMap.removePolygonClickListener(polygonClickListener)
Polylines
A Polyline
is a continuous line composed of one or more line segments. Each endpoint of the line segments in a Polyline
is a coordinate on the map. Set the list of Polyline
coordinates using the PolylineOptions
class.
The list should contain at least two points.
You can also define the appearance of a Polyline
by setting its color and width. Polyline width can be defined as a single value (which will be the same for all zoom levels), or different polyline widths can be set for different zoom levels. The width will be then interpolated for all the zoom levels in between. Line widths for zoom levels outside of the specified range will be clamped to the nearest specified value.
In addition to setting the color and width of the Polyline
, you can change the shape of its ends. There are several different shapes that you can apply to the start and end of the line. They are defined as CapType
s.
1val lineColor = Color.rgb(0.2f, 0.6f, 1.0f)2val outlineColor = Color.rgb(0f, 0.3f, 0.5f)3val polylineOptions =4 PolylineOptions(5 listOf(6 GeoPoint(latitude = 52.33744437330409, longitude = 4.84036333215833),7 GeoPoint(latitude = 52.3374581784774, longitude = 4.88185047814447),8 GeoPoint(latitude = 52.32935816673911, longitude = 4.910078096170823),9 ),10 lineColor = lineColor,11 lineWidths = listOf(WidthByZoom(10.0)),12 outlineColor = outlineColor,13 outlineWidths = listOf(WidthByZoom(1.0)),14 lineStartCapType = CapType.InverseDiamond,15 lineEndCapType = CapType.Diamond,16 )17val polyline = tomTomMap.addPolyline(polylineOptions)
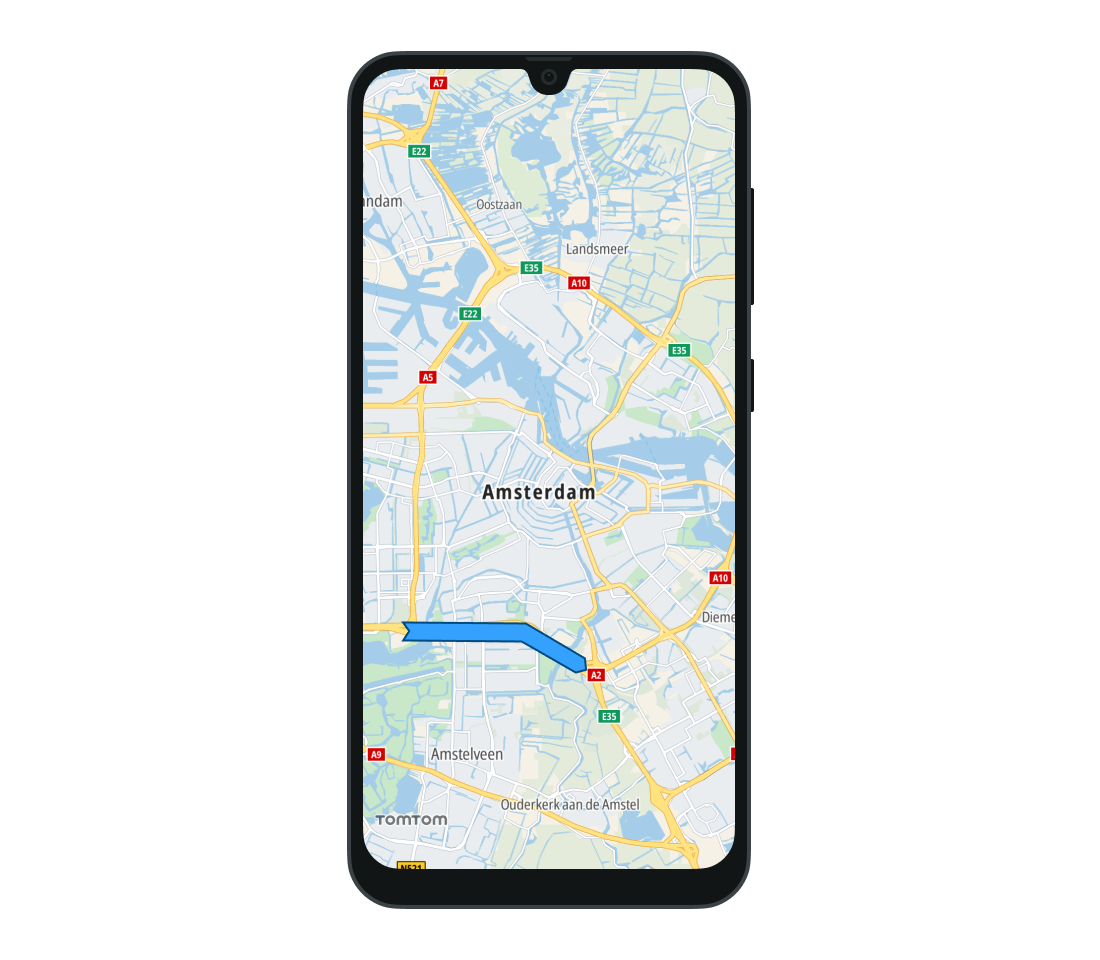
To display a polyline on the map, use TomTomMap.addPolyline(PolylineOptions)
method with PolylineOptions
describing desired polyline properties. Use the returned Polyline
object to modify its properties or remove it from the map. To remove polylines with a specific tag from the map, use the TomTomMap.removePolylines()
method.
tomTomMap.removePolylines()
Use the PolylineClickListener
to listen for click events performed on any Polyline
added to the map. Add the listener to the TomTomMap
object. Then whenever a Polyline
is clicked, the PolylineClickListener.onPolylineClicked(Polyline)
method is called with that specific Polyline
instance as a parameter.
1val polylineClickListener =2 PolylineClickListener { polyline: Polyline -> /* YOUR CODE GOES HERE */ }3tomTomMap.addPolylineClickListener(polylineClickListener)
Remove a previously set listener by calling the TomTom.removePolylineClickListener(PolylineClickListener)
with the listener instance as a parameter.
tomTomMap.removePolylineClickListener(polylineClickListener)
Next steps
Since you have learned how to draw different shapes on the map, here are the recommended next steps: