Reverse geocoding
The Reverse Geocoding module translates a coordinate (37.786505, -122.3862
) into human-understandable information like a street address or street element ("30 The Embarcadero, San Francisco, CA 94111"). Tracking applications use this tool to receive a GPS feed from a device or asset and obtain the corresponding address.
The type of response depends on the available data:
address
: Returns the most detailed information.street element
: Less detailed; returned when there is no address data.geography
: Returns the smallest available geographic data.- This is returned when there is no address or street element data.
- You can specify the geographic level to obtain a geometric element (see the
areaTypes
parameter).
Initializing
To use the Reverse Geocoding SDK, you need to configure its credentials and add the SDK as a dependency:
- Get your TomTom API Key and configure the project as described in the Project setup guide.
- Add the
Reverse Geocoding
module dependency in thebuild.gradle.kts
of your application module and synchronize the project.implementation("com.tomtom.sdk.search:reverse-geocoder:1.23.2")implementation("com.tomtom.sdk.search:reverse-geocoder-online:1.23.2")
After synchronizing the project, initialize the ReverseGeocoder
interface. This interface is the entry point to using the TomTom Reverse Geocoding SDK. There can be multiple implementations of this interface.
val reverseGeocoder: ReverseGeocoder = OnlineReverseGeocoder.create(context, "YOUR_TOMTOM_API_KEY")
Making a reverse geocoding call
Specify the reverse geocoding request parameters with the ReverseGeocoderOptions
class. The only mandatory parameter for the call is the position of the location for which you want to obtain an address. You can also set optional parameters to request more data, like geometry or road use and speed limits. A detailed description of all the parameters can be found in the Reverse Geocoding documentation.
1val coordinate = GeoPoint(52.37650747883495, 4.908392018265384)2val reverseGeocoderOptions =3 ReverseGeocoderOptions(4 position = coordinate,5 )
Once you have a ReverseGeocoderOptions
object, provide it to the Reverse Geocoding request. You can achieve this asynchronously with a response callback using ReverseGeocoderCallback
or synchronously by obtaining a ReverseGeocoderResponse
, as detailed in the section called Making search API calls.
If the call succeeds, the returned result is in the ReverseGeocoderResponse
. If an error occurred, it is in the SearchError
.
1reverseGeocoder.reverseGeocode(2 reverseGeocoderOptions,3 object : ReverseGeocoderCallback {4 override fun onSuccess(result: ReverseGeocoderResponse) {5 // YOUR CODE GOES HERE6 }78 override fun onFailure(failure: SearchFailure) {9 // YOUR CODE GOES HERE10 }11 },12)
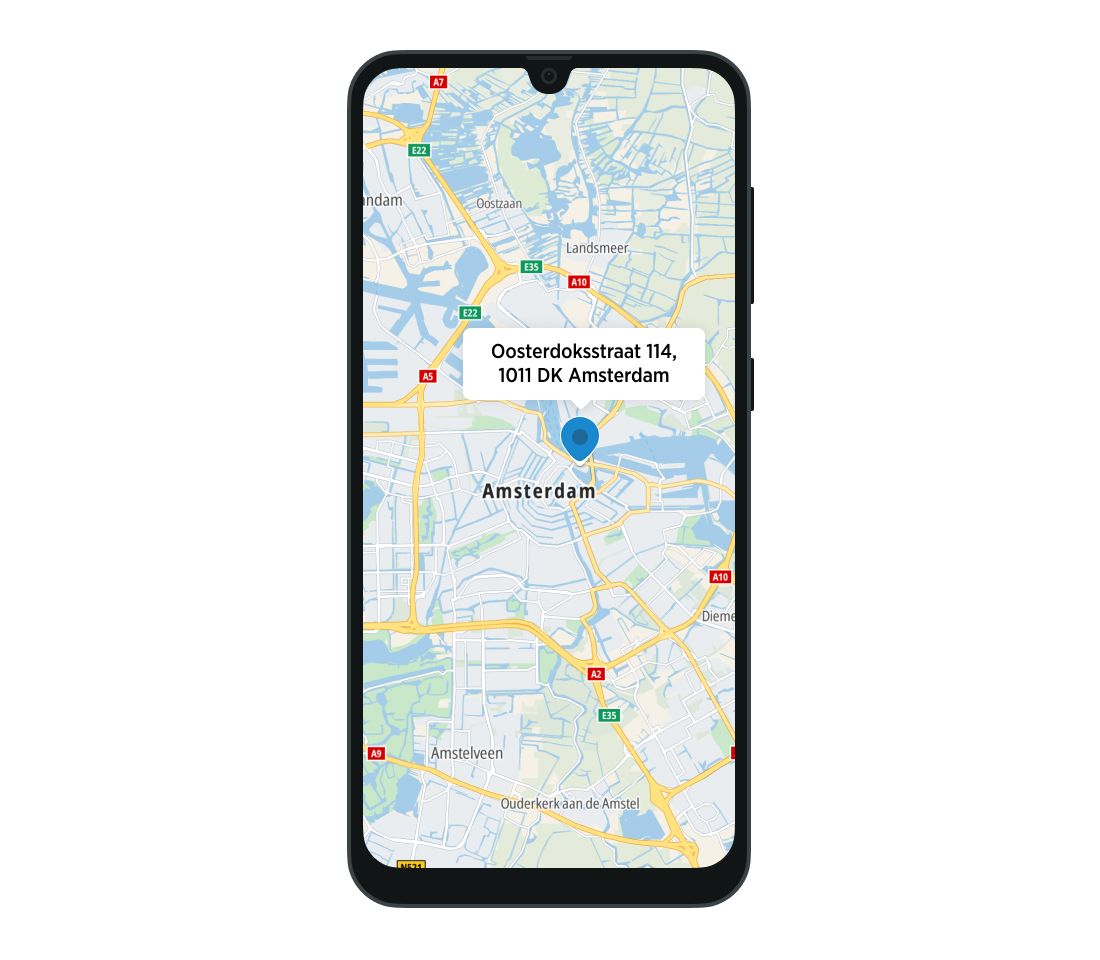
Next steps
Since you have learned how to reverse geocode, here are recommendations for the next steps: