Overlays
The Map Display SDK allows you to draw different shapes on the map. You can add a Marker
, Circle
, Polygon
or Line
. All of them inherit from the Annotation
protocol, which contains a unique ID and a tag. The Marker
annotation is described in detail in the Markers guide.
All of the shapes can be removed from the map using this single method.
map.removeAnnotations()
In addition, each of the annotations can be removed separately by calling the TomTomMap.remove(annotation:)
method with the Annotation
instance as a parameter.
map.remove(annotation: annotation)
You can listen to click events performed on all of the shapes which include a Marker
. To do so, you need to implement a method from the MapDelegate
protocol. It returns the following parameters when the user taps on a shape:
TomTomMap
- instance of the map to which annotations were added.Annotation
- instance of the clicked shape.CLLocationCoordinate2D
- the coordinates of the click.
1func map(_ map: TomTomMap, onInteraction interaction: MapInteraction) {2 switch interaction {3 case let .tappedOnAnnotation(annotation, coordinate):4 /* YOUR CODE GOES HERE */5 break6 default:7 break8 }9}
Circles
You can draw a circle on the map by specifying:
- Coordinates for the center.
- Radius of the circle in meters.
- Circle color.
1let circleOptions = CircleOptions(2 coordinate: CLLocationCoordinate2DMake(52.367956, 4.897070),3 radius: 3000.0,4 fillColor: UIColor(red: 0.93, green: 0.62, blue: 0.0, alpha: 0.3)5)6let circle = try? map.addCircle(options: circleOptions)
The AnnotationsActions.addCircle(options:)
method adds the Circle
to the map. If the operation was successful the Circle
instance is returned. Otherwise, the method throws an exception.
The Circle
object can be used to read the location of its center and its radius. You can also use its property Circle.isVisible
to toggle its visibility.
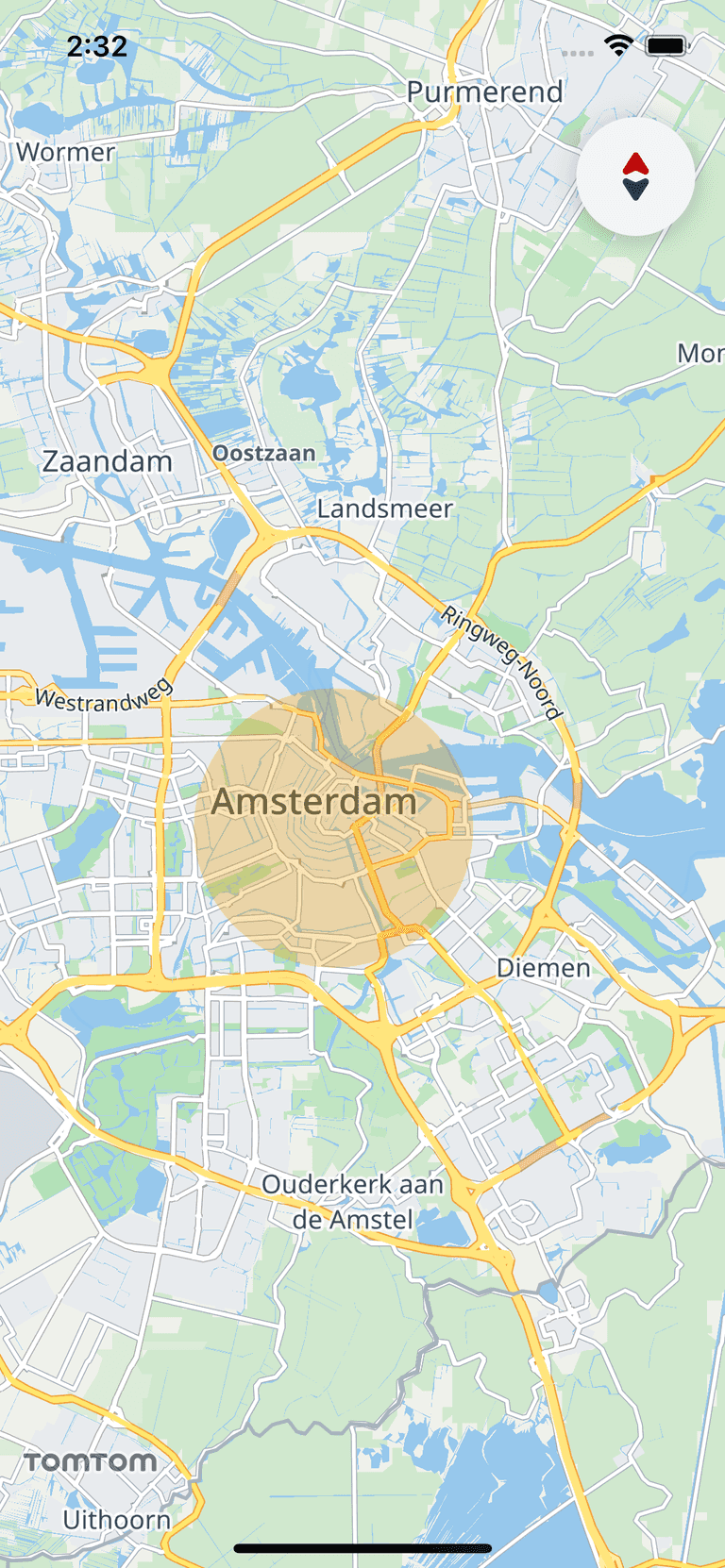
Polygons
The Map Display module also supports drawing polygons on the map. The polygons are configured with the PolygonOptions
structure. Drawing a polygon requires at least 3 vertices to be specified. The coordinates of the polygon are provided as a list of CLLocationCoordinate2D
objects, listed in counter-clockwise order.
In addition to defining the shape of the figure, you can specify its fill color and display an image inside it. You can also set the color and width of the polygon outline stroke.
To set the image inside a Polygon
use the TextureOptions
structure. To preserve the size of the original image, set its TextureOptions.isImageOverlay
property to false. If the image is smaller than the polygon, it will be tiled. To scale the image to cover the whole polygon, set it to true.
If you add an image inside the polygon together with the
PolygonOptions.fillColor
, their pixels will be multiplied. By default, thePolygonOptions.fillColor
property is set to blue. To preserve the original image colors, change it to white.
1var polygonOptions = PolygonOptions(coordinates: [2 CLLocationCoordinate2DMake(52.33744437330409, 4.84036333215833),3 CLLocationCoordinate2DMake(52.3374581784774, 4.88185047814447),4 CLLocationCoordinate2DMake(52.32935816673911, 4.910078096170823),5 CLLocationCoordinate2DMake(52.381705486736315, 4.893630047460435),6 CLLocationCoordinate2DMake(52.385294680380866, 4.846939597146335),7])8polygonOptions.outlineColor = .blue9polygonOptions.outlineWidth = 2.010polygonOptions.fillColor = UIColor(red: 0.0, green: 0.0, blue: 1.0, alpha: 0.5)11let polygon = try? map.addPolygon(options: polygonOptions)
The AnnotationsActions.addPolygon(options:)
method returns a Polygon
object. If the operation was successful the Polygon
instance is returned. Otherwise, the method throws an exception. Use it to modify the shape’s properties, toggle its visibility with the Polygon.isVisible
property, and get its CoordinateBounds
.
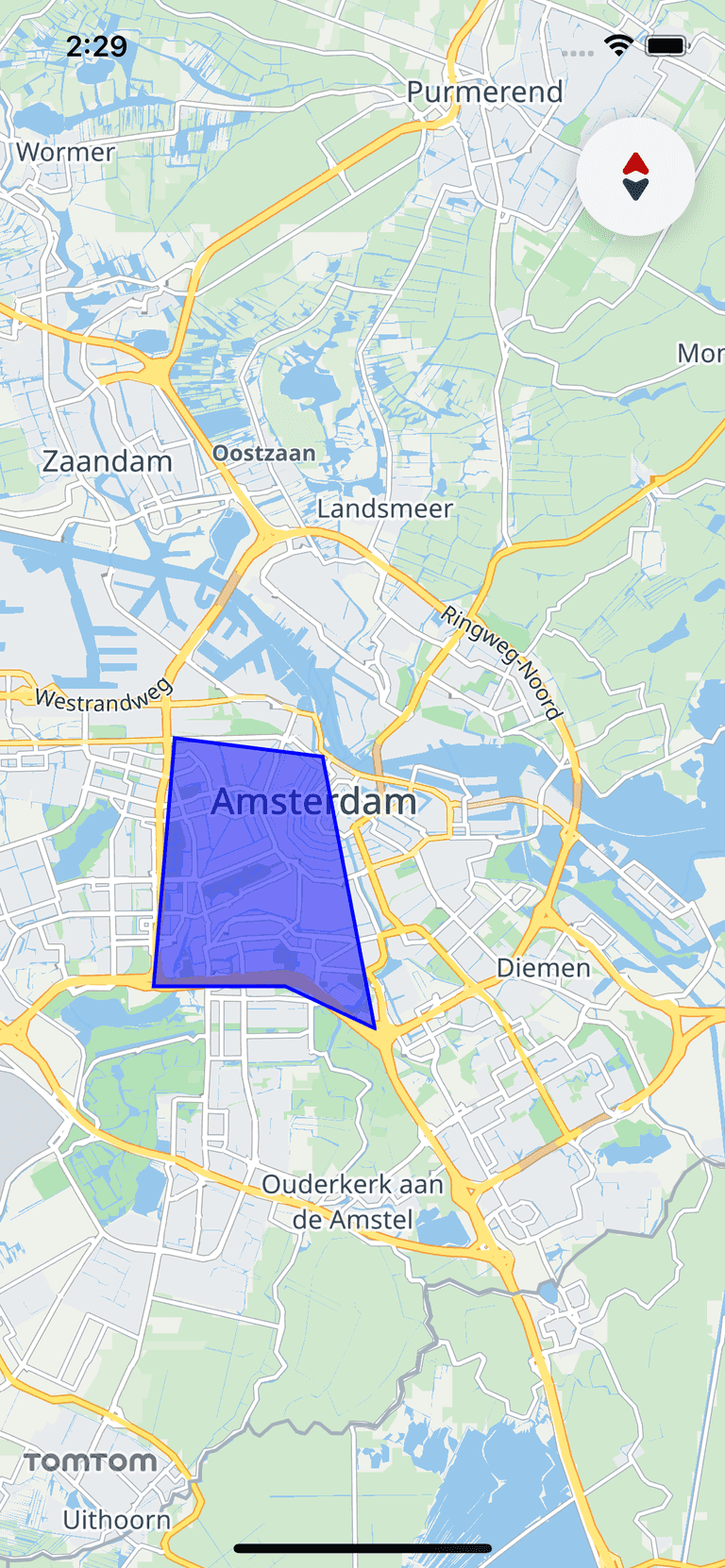
Lines
A Line
is a continuous line composed of one or more segments. Each endpoint of the segments in a Line
is a coordinate on the map. Set the list of Line
coordinates using the LineOptions
structure.
The list should contain at least two points.
The appearance of the Line
can be configured to meet your requirements.
The color, width, and outline of the Line
can be customized. You can also change the shape of its ends. There are several different shapes that you can apply to the start and end of the line. They are defined as LineCapType
s.
The
Line
can be customized with more details. Look for the other properties of theLineOptions
in the API reference.
1let lineColor = UIColor(red: 0.2, green: 0.6, blue: 1.0, alpha: 1.0)2let outlineColor = UIColor(red: 0.0, green: 0.3, blue: 0.5, alpha: 1.0)3var lineOptions = LineOptions(coordinates: [4 CLLocationCoordinate2DMake(52.33744437330409, 4.84036333215833),5 CLLocationCoordinate2DMake(52.3374581784774, 4.88185047814447),6 CLLocationCoordinate2DMake(52.32935816673911, 4.910078096170823),7])8lineOptions.outlineAppearance.lineStartCap = .inverseDiamond9lineOptions.outlineAppearance.lineEndCap = .diamond10lineOptions.lineColor = lineColor11lineOptions.outlineAppearance.outlineColor = outlineColor12lineOptions.lineWidth = 10.013let line = try? map.addLine(options: lineOptions)
To display a polyline on the map, use the TomTomMap.addLine(options:)
method with LineOptions
describing the desired line properties. If the operation was successful the Line
instance is returned. You can use the returned object to read its coordinates and CoordinateBounds
. It can also be used to change its visibility with the Line.isVisible
property.
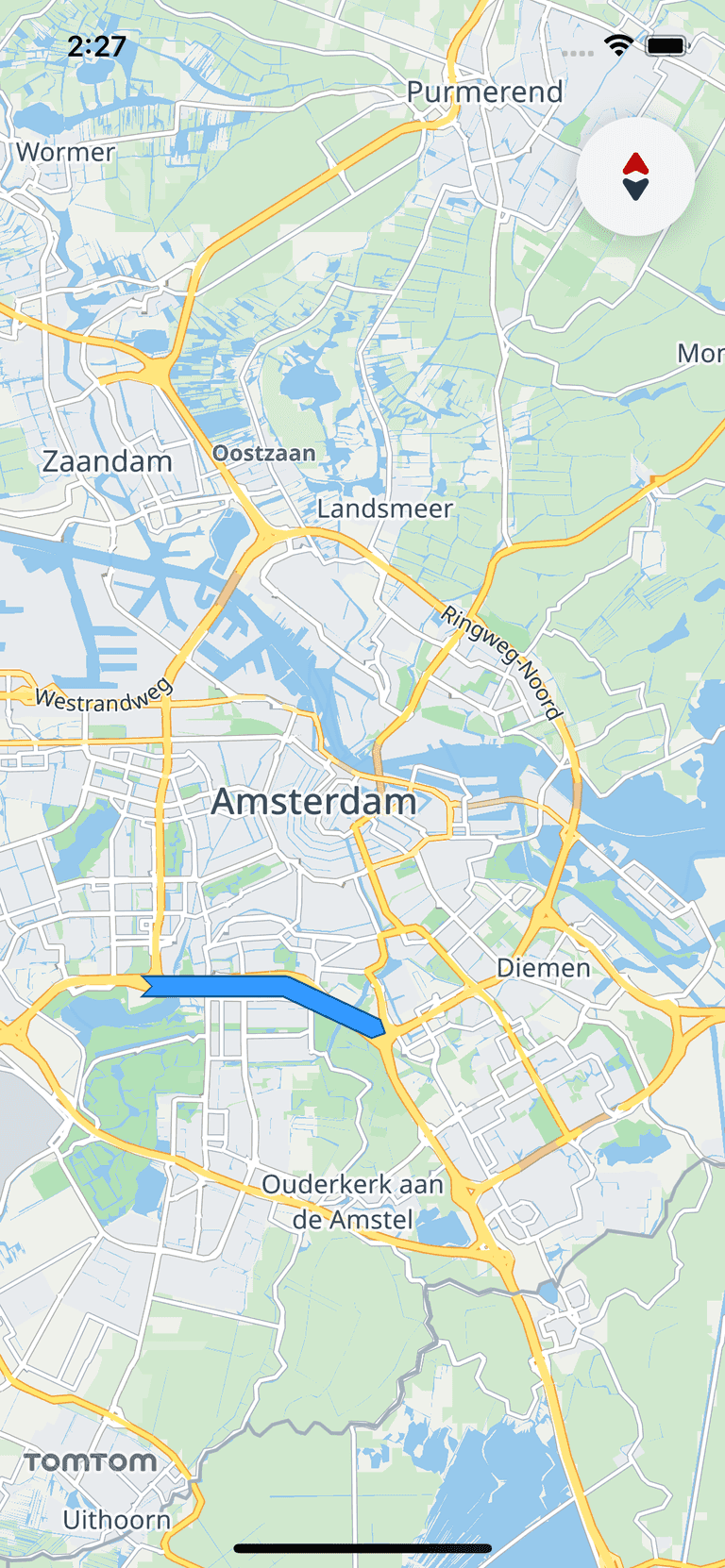