Using TomTom Data via APIs to Improve Delivery ETA Accuracy
)
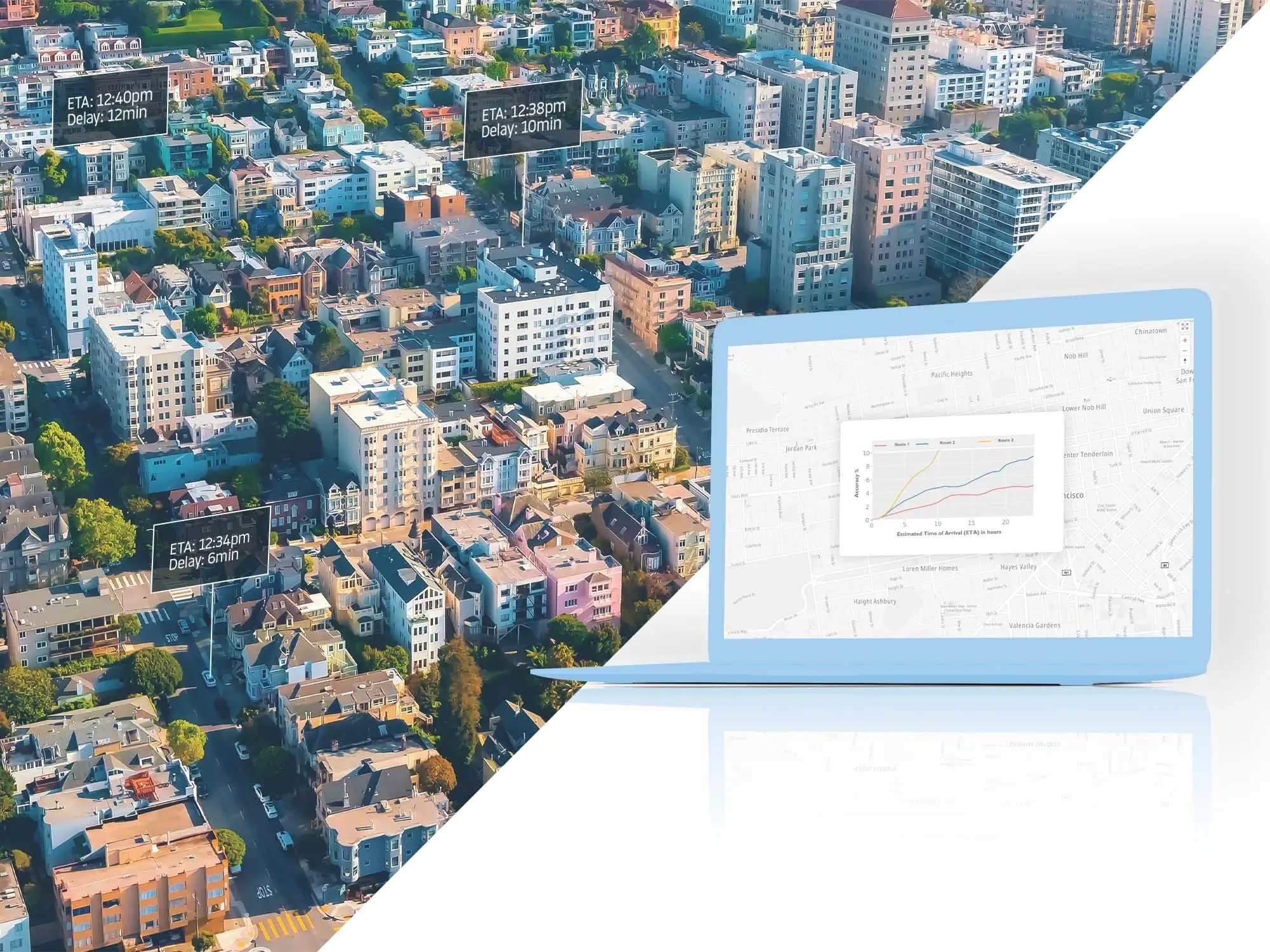
We’ll show you how to use data from the TomTom Routing API and a little bit of Python to make delivery ETAs more accurate.
When using delivery applications, it’s crucial to know roughly when a delivery will arrive, whether you’re expecting food, a device, documents, or a service person. The estimated time of arrival (ETA) helps determine which drivers can pick up a customer or an order quickly, the fastest route to the customer, and when the customer should expect their package to arrive.
One straightforward way to improve delivery ETA is to call the Routing API periodically to get ETA updates. This method is most suitable for real-time or nearly real-time applications when the driver is currently delivering the goods. Periodic calls to the Routing API can use bandwidth and incur additional costs, so it doesn’t make sense to use this method when it isn’t necessary.
In contrast, offline tools are best for planning and optimizing a delivery company’s routes. These tools can use historical traffic data to help the company decide when to send a delivery — without using excessive resources. The TomTom Routing API provides route information for a vast range of dates and times.
For example, we can send multiple GET requests to the Routing API with varying dates and times. We can, for instance, fix the day of the week then change the departure times. We then get the ETA for each route and select the one with a minimal ETA to optimize our course.
However, the more routes we must analyze, the longer it takes. A data science solution helps by automatically gathering large datasets (with variable route parameters) from the Routing API. We can build a machine learning (ML) model based on that information, and the model can then help us find the most optimal departure time to ensure the delivery arrives when expected. For instance, we can discover the route with the shortest travel time to ensure temperature-sensitive deliveries arrive as quickly as possible, with an accurate ETA to help customers know when to expect our driver.
Here, we’ll use the TomTom Routing API, Python, and scikit-learn to create such a model. The problem described above is an optimization (we need to find the route with the shortest travel time). To keep it simple, we’ll get route information for ten different departure times, then build the linear regression model to predict the ETA for a given departure time. We use scikit-learn for the machine learning portion of our solution. It will help us learn (fitting data to a model) and predict (using fitted parameters to predict ETAs).
For this tutorial, you’ll need some understanding of Python, a TomTom account, and a registered API key for the TomTom Maps SDK.
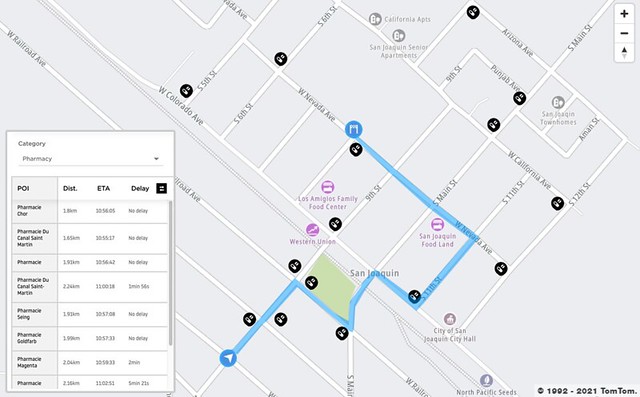
Improving Delivery ETA Accuracy
TomTom’s Maps APIs provide rich data collections that we can use to enhance accuracy for delivery applications. Developers can integrate this information with transportation management systems, truck tracking systems, mapping data, and predictive analytics. Data scientists can also use machine learning and data analytics to improve our applications.
TomTom Maps APIs provide a great developer experience because of their flexibility. Additionally, the variety and richness of data generated by TomTom Maps APIs make them the ideal choice for data scientists who aim to build accurate and handy prediction models based on mapping data. Significant benefits of using TomTom APIs as input for data science predictive models include:
• Increased ETA accuracy • Onboarding quickly • Suitable to use with AI and machine learning • Proactive service and customer satisfaction
Developers and data scientists first pull data from TomTom Maps APIs and put the information to work using our favorite tools and libraries, like Python, Pandas, scikit-learn, TensorFlow, and PyTorch. Then, we merge this data into our data science applications to get the desired results. The figure below presents an outline of using data science techniques to improve ETA based on the data collected from TomTom Maps APIs.

Using the Routing API
We start building our ETA optimization solution using the Routing API. The best way to familiarize yourself with this service is through the TomTom Developer portal. The portal’s user interface (UI) is similar to Swagger (that API developers frequently use) with a set of text boxes and dropdown lists to configure request parameters.
The image below shows an example of using this portal to determine the route between San Francisco (37.77493,-122.419415) and Los Angeles (34.052234,-118.243685). We specify these route endpoints in the locations parameter. For this tutorial, we’ll also use the departAt field and assume the delivery is by truck (travelMode):


Once we finish selecting the appropriate values, we scroll down and click Execute. The portal returns a sample response (here we use JSON format):


The results are:
{
"formatVersion": "0.0.12",
"routes": [
{
"summary": {
"lengthInMeters": 624544,
"travelTimeInSeconds": 24670,
"trafficDelayInSeconds": 0,
"trafficLengthInMeters": 0,
"departureTime": "2021-10-20T10:00:00-07:00",
"arrivalTime": "2021-10-20T16:51:10-07:00"
},
"legs": [
{
"summary": {
"lengthInMeters": 624544,
"travelTimeInSeconds": 24670,
"trafficDelayInSeconds": 0,
"trafficLengthInMeters": 0,
"departureTime": "2021-10-20T10:00:00-07:00",
"arrivalTime": "2021-10-20T16:51:10-07:00"
},
"points": [
{
"latitude": 37.77499,
"longitude": -122.41948
},
// Note that most of the points were omitted
{
"latitude": 34.05238,
"longitude": -118.24359
}
]
}
],
"sections": [
{
"startPointIndex": 0,
"endPointIndex": 4779,
"sectionType": "TRAVEL_MODE",
"travelMode": "truck"
}
]
}
]
}
As the results above show, we can read ETA from the arrivalTime value (2021-10-22T17:00:10-07:00). We can also get the estimated travel time, expressed in seconds, from the travelTimeInSeconds field. Here, the travel time is approximately seven hours.
Fetching Data Using Python
We now have all the required information to write the Python script for fetching ETAs. We just need to prepare the request link based on the previous section then use a dedicated tool for sending the GET request to the TomTom API. Here, we use the Requests pip package, a standard Python tool.
Of course, we also need to encode the request URL correctly, so we use urllib.parse.
This code constructs the request URL to get the route information between San Francisco and Los Angeles:
import requests
import urllib.parse as urlparse
# Route parameters
start = "37.77493,-122.419415" # San Francisco
end = "34.052234,-118.243685" # Los Angeles
routeType = "fastest" # Fastest route
traffic = "true" # To include Traffic information
travelMode = "truck" # Travel by truck
avoid = "unpavedRoads" # Avoid unpaved roads
departAt = "2021-10-20T10:00:00" # Departure date and time
vehicleCommercial = "true" # Commercial vehicle
key = "key=<TYPE_YOUR_API_KEY_HERE>" # API Key
# Building the request URL
baseUrl = "https://api.tomtom.com/routing/1/calculateRoute/";
requestParams = (
urlparse.quote(start) + ":" + urlparse.quote(end)
+ "/json?routeType=" + routeType
+ "&traffic=" + traffic
+ "&travelMode=" + travelMode
+ "&avoid=" + avoid
+ "&vehicleCommercial=" + vehicleCommercial
+ "&departAt=" + urlparse.quote(departAt))
requestUrl = baseUrl + requestParams + "&key=" + key
Once we have the request, we send it like this:
response = requests.get(requestUrl)
The resulting response object contains the HTTP status code as well as the route information. Here is how to parse the data to get the first route’s ETA and travel time:
if(response.status_code == 200):
# Get response's JSON
jsonResult = response.json()
# Read summary of the first route
routeSummary = jsonResult['routes'][0]['summary'];
# Read ETA
eta = routeSummary['arrivalTime']
# Read travel time and convert it to hours
travelTime = routeSummary['travelTimeInSeconds'] / 3600
# Print results
print(f"{departAt}, ETA: {eta}, Travel time: {travelTime:.2f}h")
You can find the complete script on GitHub.
First, edit the script to replace the API key placeholder (line 12) with your API key. Then, run the script (python3 01_GetData.py). You should get results similar to the following screenshot:
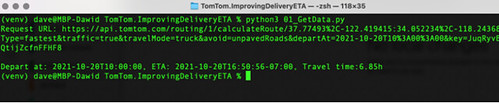
Getting Training Data
Next, we need to send several requests for various departure times to get data for building our model. You can find the complete script on GitHub, built on the previous code. Specifically, there is a getEta function, which accepts one parameter, departureTime. Based on this, the getEta function returns a tuple comprising three items: departure time, ETA, and travel time. To determine ETAs for various departure times, we need to call getEta multiple times, like so:
# Departure times
departureTimeStart = datetime.datetime(2021, 10, 20, 0, 0, 0)
for i in range(0,23):
# Update an hour
departureTime = departureTimeStart.replace(hour=departureTimeStart.hour + i)
# Format datetime string
departureTime = departureTime.strftime('%Y-%m-%dT%H:%M:%S')
# Get and print ETA
getEta(departureTime)
This code prints the arrival times and travel times for each departure time as follows:

We now see that by leaving San Francisco at 10 PM, the route will take about 6.5 hours. Conversely, if we leave San Francisco at 4 PM, the travel time will be about 7.3 hours. Hence, we can save nearly an hour by choosing one departure time versus another.
Training the Model to Predict ETA
We can save effort and analyze more departure times using an ML model to determine the optimal departure time. To prepare the model, we first copy the data printed by the previous script to the CSV file: train_data.csv. Also, we add one more column, departure hour, containing only the hour part of the departure datetime. We do this to simplify linear regression.
Now, we can use Pandas to read the CSV (see 03_ModelTrainingAndPrediction.py):
import pandas as pd
from sklearn.linear_model import LinearRegression
# Read train data
dataFrame = pd.read_csv('train_data.csv',sep=';')
# Use DepartureHour column as X
X = dataFrame.DepartureHour
# Use TravelTimeInHours column as Y
Y = dataFrame.TravelTimeInHours
As shown above, we use DepartureHour and TravelTimeInHours as our X (predictor) and Y (criterion variable).
Then, we use scikit-learn to train the Linear Regression model:
# Train the model
from sklearn.linear_model import LinearRegression
import numpy as np
model = LinearRegression().fit(np.array(X).reshape(-1,1), Y)
Finally, we predict travel times for the departure hours that the model has not already seen, like so:
# Predict travel time for different departure hours
departureHours = [1.5, 3.12, 5.17, 16.05, 10.15, 20.29]
for departureHour in departureHours:
predictedTravelTime = model.predict(np.array(departureHour).reshape(-1,1))
print(f"Departure hour: {departureHour}, Predicted travel time:
{predictedTravelTime[0]:.2f} h")
The above code produces the following result:

With this solution in hand, route planners can now find the best departure time. Our estimator (model) is not perfect as it uses the simple linear model. However, we can always replace it with a more comprehensive ML model. Also, we can use more features from the TomTom Routing API (like routeType, avoid, hilliness, windingness, and vehicleMaxSpeed) during training to further optimize our route planning.
Conclusion
The problem of predicting estimated travel time accuracy isn’t straightforward. It’s a complicated process that must consider all types of traffic modes without affecting application performance. Once we know the speed limits of roads and have an up-to-date road routing plot and traffic information, our application can analyze the expected arrival time of different routes to predict the optimal route needed for customer satisfaction.
The data science module uses TomTom’s historical traffic data to predict the optimal time to leave one city to reduce travel time. Choosing this route helps ensure the customer’s delivery arrives with minimal travel time (ideal for temperature-sensitive items, for instance) with a more accurate ETA based on actual historical data for that time of day. Pulling in additional details for each route can further improve ETA accuracy.
Now that you’ve seen the capabilities of TomTom Maps APIs and how you can improve the accuracy of your ETAs, sign up and start using TomTom Maps!