Building a Traffic Incident App with the TomTom Maps SDK for Android
&w=256&q=90)

With TomTom Maps, you can build an Android app with a real-time traffic incident dashboard – you can even display traffic flow and allow users to search for incidents. We'll go over how using RESTful APIs and the TomTom SDK for Android.
Every day, hundreds of people travel by car to their offices, drop off their kids at school, and do grocery shopping at their nearest shopping center. When running these errands, people want to take the shortest route so they can get to their destination quickly.
But traffic isn’t static. Road and traffic conditions consistently change. Unfortunately, this impacts transit time and the accessibility of businesses and points of interest (POIs). For example, an often traveled route might face some incidents caused by construction or an accident on the road.
Mapping tools and applications are key to avoiding these run-ins. TomTom APIs use real-time data from the roads to provide a live view of the traffic to help users see if the route they want to take is blocked. This data is constantly refreshed, ensuring users have an up-to-date notice of any road closures or blockages. TomTom APIs include a very powerful Search API that you can use in your Android apps to help your users find the fastest routes to their destinations.
With TomTom Maps, you can build an Android app with a real-time traffic incident dashboard. You can even display traffic flow and allow users to search for incidents. Ready to build your own traffic app? Let’s get started.
We assume you’re familiar with Android development and Kotlin — although if you’ve done Android development in Java, you should be able to follow along easily.
To view the full project code, check out this repository.
Creating and Configuring an Android App
You can install the TomTom SDK for Android using Gradle in Android Studio. Our documentation gives you more info on how to configure the Android app to use TomTom APIs. At the time of writing this article, the current version of the API is 2.4807. Check out our Getting started guide for basic configuration.
The TomTom SDK for Android is a wrapper around RESTful APIs. Most of the components and services are standalone Android types, which you can add to your Android apps. For example, a TomTom map fragment enables your customers to see real-time traffic flow and incidents or diversions on a route.
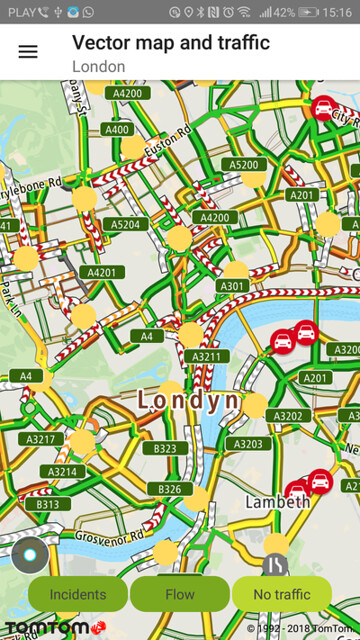
As seen in this example, a TomTom map can display real-time traffic incidents as well as traffic flow. The TomTom SDK for Android has even more features that you can use to build similar experiences for your customers.
Building a Real-Time Incidents Dashboard
TomTom APIs enable you to build an experience in your Android applications that allow users to know of incidents before they reach their destination. This includes construction, road closures, and any accidents that are on the route to their destination or other POIs.
In your Android app, start by creating an instance of a TrafficApi object. This object exposes all the methods needed to request incidents in a specific region (with a specific zoom level) and listen to the responses from the API. To construct an object, you must pass the context and the API key. If you don’t have an API key, create one for free on the TomTom Developers website.
val trafficApi: TrafficApi = OnlineTrafficApi.create(this, apiKey)
You can create this as a field inside your activity and initialize it inside the onCreate method.
Next, create an incident query object to fetch the incidents. Create the object using builder:
val builder: IncidentDetailsQuery = IncidentDetailsQueryBuilder.create(
IncidentStyle.S1,
LONDON_BOUNDING_BOX,
DEFAULT_ZOOM_LEVEL_FOR_EXAMPLE,
TRAFFIC_MODEL_ID
)
.withExpandCluster(true)
.build()
The fields above are:
private val topLeftLatLng: LatLng = LatLng(51.6918741, -0.5103751)
private val bottomRightLatLng: LatLng = LatLng(51.2867602, 0.3340155)
private val LONDON_BOUNDING_BOX: BoundingBox = BoundingBox(
topLeftLatLng,
bottomRightLatLng
)
private val DEFAULT_ZOOM_LEVEL_FOR_EXAMPLE = 12
private val TRAFFIC_MODEL_ID: String = "-1"
These details are for London. You can get details for your region using your latitude and longitude.
Another detail to note is the zoom level. We’re using 12, the highest level, to narrow in on our area as closely as possible. If we decrease the zoom level, we can zoom out and see a broader area. When zoomed out, multiple incidents that take place in the same area will be clustered together. TomTom makes it easy to display incidents as groups, avoiding overlapping incident markers.
Next, create an instance of our response listener:
val resultListener = object : IncidentDetailsResultListener {
override fun onTrafficIncidentDetailsResult(result: IncidentDetailsResponse)
{
this?.let { ctx ->
result.incidents.forEach { incident ->
incidentsContainer.add(incident)
}
// Create the adapter
val incidentsAdapter = IncidentsAdapter(incidentsContainer)
if (incidentsView != null) {
incidentsView?.adapter = incidentsAdapter
} else {
System.out.println("[INFO] incidentsView is null")
}
}
}
override fun onTrafficIncidentDetailsError(error: Throwable) {
Toast.makeText(applicationContext, error.message,
Toast.LENGTH_SHORT).show()
}
}
Now, just request the incidents list and wait for the results to come in:
trafficApi.findIncidentDetails(builder, resultListener)
The listener loads the results on the Android UI using the RecyclerView method. To learn how this API works, please see the Android documentation.
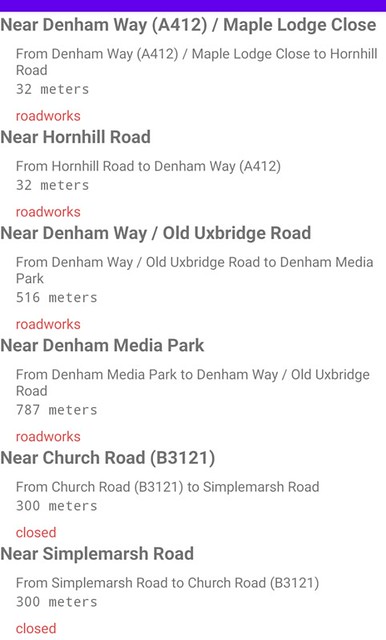
Now, we have a clean, simple UI. Our app loads traffic incident data from TomTom so users can see information such as where the incident originated and how long the roads will be affected.
Displaying Traffic Flow
TomTom APIs allow you to show the current traffic flow on a user’s route. Knowledge of traffic flows is helpful when determining the optimal route.
To add traffic flow to a TomTom map in your app, you can simply call the turnOnTrafficFlowTiles method in the TrafficSettings object. See the TrafficSettings object for more information.
Tip: You can also use the user’s current location to regularly update the traffic flow for the user’s active route.
Learn more about the Traffic Flow API and how you can embed traffic flows in your Android applications.
Searching for Incidents
In our example scenario, we use a pinpoint location to search for incidents in that area. But what if you want to let your users explore incidents in other areas? You can use TomTom’s Search API to query the location of places along your route, like a grocery store.
With the Traffic Incidents API and the Traffic Flow API, you can guide the user to a faster and safer route. When they change their route, you can query again to find the grocery store for them on the new route. By combining multiple API endpoints, you can provide the users with an enjoyable travel experience.
The Search API is the umbrella API for everything related to places. Although not directly an Incident API, it can help you add helpful labels to incidents by using Reverse Geocoding to find the name of the nearest major intersection or highway interchange to the incident’s coordinates.
Conclusion
TomTom SDKs can help you enable your customers to find the best routes and avoid roadblocks. TomTom SDKs are available for several platforms, including Android. Also, they support the Kotlin programming language out of the box. TomTom APIs provide live data from the roads that you can use in your Android apps. You can use this to help your customers make smarter decisions when they travel.
We encourage you to try out the TomTom SDKs for your applications. You can register for free and get an API token. With TomTom SDKs, you get 2,500 free transactions every day to try the API before deciding.
Also, browse through the official tutorials and documentation to learn more about what you can solve and create using TomTom APIs.
* Required field. By submitting your contact details to TomTom, you agree that we can contact you about marketing offers, newsletters, or to invite you to webinars and events. We could further personalize the content that you receive via cookies. You can unsubscribe at any time by the link included in our emails. Review our privacy policy.