Routes
The Map Display module allows you to handle route planning and driving actions on the map. This guide covers how to draw a Route
, show the progress along it, and track the user’s location during the trip.
If you’re looking for route planning, see the Planning a route guide.
Adding a route
To show a Route
on the map, call the RouteActions.addRoute(_:)
method. You can configure its shape and appearance with the RouteOptions
struct. Drawing a route on the map requires a list with coordinates of the route points.
You can also define its appearance by setting the color, width, and departure and destination marker images. Route width can be defined as a single value (which will be the same for all zoom levels), or different route widths can be set for different zoom levels. The width will then be interpolated for any zoom levels in between. RouteOptions
also supports specific routing features such as reachable distance, or providing a list of instructions along the route. For a detailed description of all the parameters see the API reference.
1var routeOptions = RouteOptions(coordinates: calculatedRoute.geometry)2routeOptions.color = UIColor(red: 0.2, green: 0.6, blue: 1.0, alpha: 1.0)3let route = try? map.addRoute(routeOptions)
If adding the route was successful, the RouteActions.addRoute(_:)
method returns the Route
object. Otherwise, the method throws an exception. The returned Route
instance can be used to update the color, progress along a route, or observe click events.
To remove the previously added Route
, provide its instance to the RouteActions.removeRoute(_:)
method.
map.removeRoute(route)
You can also remove all routes added to the map by invoking the RouteActions.removeRoutes()
method.
map.removeRoutes()
You can get the list of all routes visible on the map with the RouteActions.allRoutes
property. You can also display an overview of all routes. The camera will be centered and zoomed properly to fit all the routes on the screen, together with applied padding. The padding is expressed in pixels.
map.zoomToRoutes(padding: 32)
RouteDelegate
You can listen for click events performed on Route
objects. To do it, set the RouteDelegate
to the Route.delegate
property. If the user clicks on the Route
its method will be called with the Route
object, clicked coordinates, and the index of the waypoint as parameters.
1func mapRoute(_: TomTomSDKMapDisplay.Route, didTapOnCoordinate _: CLLocationCoordinate2D, waypointIndex _: Int) {2 /* YOUR CODE GOES HERE */3}
Updating route progress
The progress made along the Route
can be indicated on the drawn map. The traveled part of the route is colored with the outline color. See the following example.
Progress can be updated by operating on the Route
object returned during drawing. Its value is expressed in meters. In the following example, the map will show that 2km has already been traveled.
route.progressOnRoute = Measurement<UnitLength>(value: 2000, unit: .meters)
To clear the progress along the Route
use the following method. The color of the Route
will return to the initial color.
route.clearProgress()
CameraTrackingMode
The Map Display SDK allows you to set how the camera tracks the user’s locations to suit different interaction modes.
Do this by setting CameraTrackingMode
to the TomTomMap
instance. There are six options for tracking mode:
CameraTrackingMode.none
- The camera does not track the user’s location. This is the default setting and is mainly used to show the user’s location on the map.CameraTrackingMode.followNorthUp(_:)
- The camera follows the user’s location, but its tilt and zoom do not change.CameraTrackingMode.followRouteDirection(_:)
- The camera follows the user’s location and heading to best present the route. Camera properties like tilt and zoom may be adjusted to better display the route and its guidance instructions.CameraTrackingMode.followDirection(_:)
- The camera follows the user’s location and heading to position the camera in the heading direction. Camera is always following from the top (the tilt is set to 0 degrees)CameraTrackingMode.followRouteNorthUp(_:)
- The camera centers the position marker within the safe area, fixing the camera’s heading north. The map remains unrotated, the marker’s orientation shifts, and the tilt is a top-down view at 0.CameraTrackingMode.routeOverview
- The camera attempts to fit the routes in the current view, by changing the zoom level and other camera properties.
The last three modes are mainly used in navigation applications.
To apply a given tracking mode, set the CameraTrackingMode
parameter:
map.cameraTrackingMode = .followRouteDirection()
To check what tracking mode is currently set:
let cameraTrackingMode = map.cameraTrackingMode
Instructions
The Route
added to the map can also show the location and type of maneuvers the driver must perform to follow it. These maneuvers are indicated with arrows displayed on the route (see the following image).
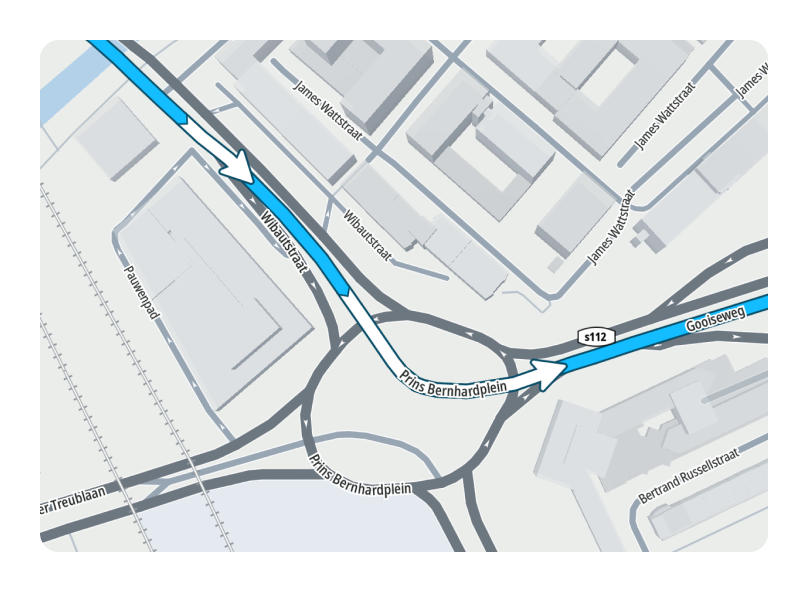
The instructions for the maneuvers have to be provided to the RouteOptions
class using its RouteOptions.instructions
property .
Instructions can be taken from Planning a route, mapped to TomTomSDKMapDisplay.RouteInstructions
and set to the RouteOptions
. You can also create RouteInstruction
on your own. A RouteInstruction
requires the distance from the start of the route to the instruction point in meters. You can also specify whether the arrow can be merged with the next arrow and define its length.
1let allInstructions = calculatedRoute.legs.flatMap { $0.instructions }2routeOptions.instructions = allInstructions.map { TomTomSDKMapDisplay.RouteInstruction(3 distanceAlongRoute: $0.routeOffset,4 length: Constants.length,5 combineWithNext: $0.combineWithNext6) }