Summoning Taxis with TomTom Maps APIs
)
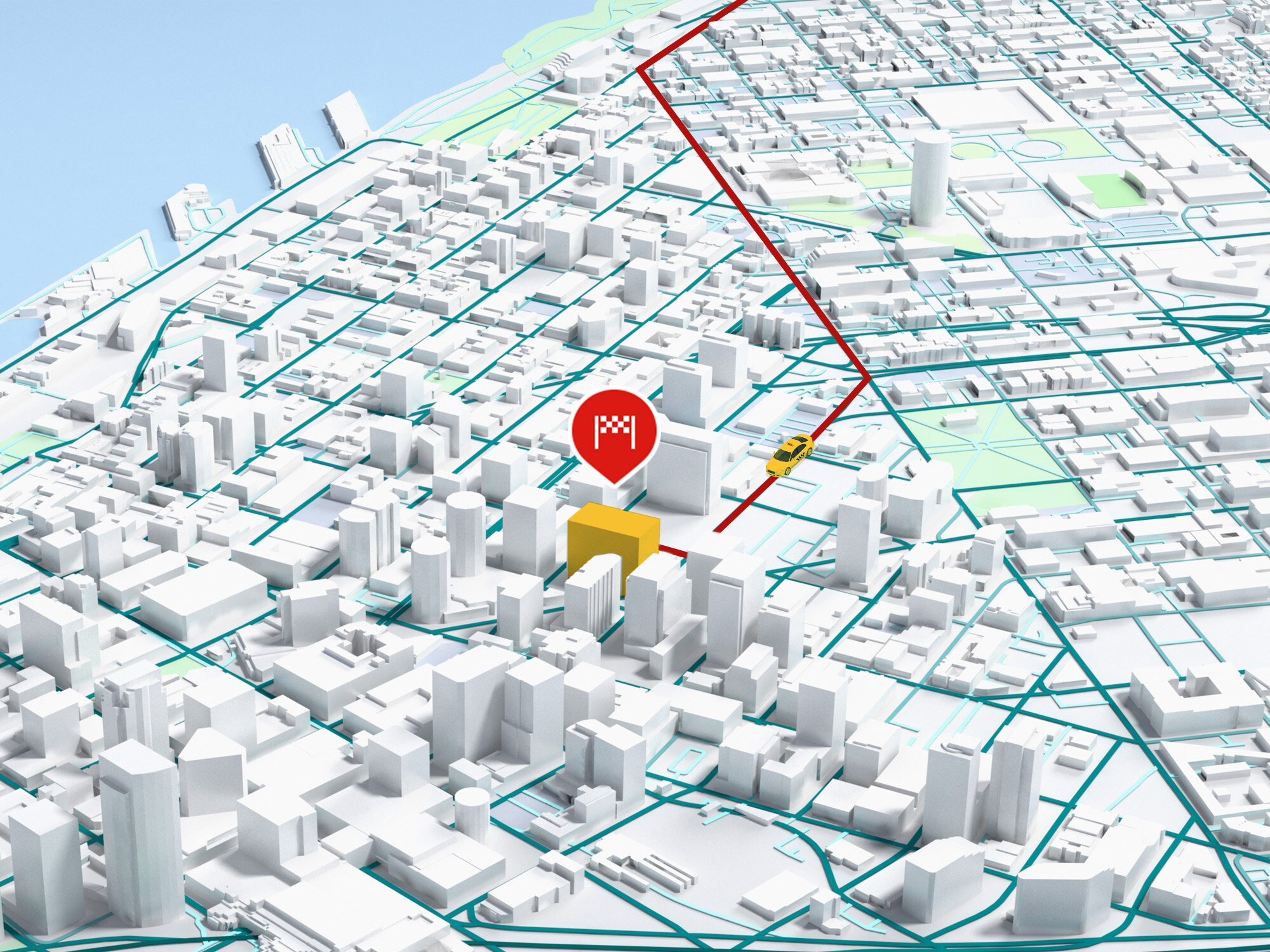
In this tutorial, we review how to program a taxi summoning service using TomTom APIs and SDKs. You just need to know a little JavaScript to follow this tutorial — but TomTom libraries support other languages too.
Taxis were the most popular driver service before ridesharing services existed. But taxis lack the ease of use — for customers and drivers — that ridesharing services offer. If customers are not near a taxi, they have to search for a company’s phone number, call and speak to dispatch, and hope that there is a driver available.
A phone call is not always convenient. A customer at a hotel may be traveling outside their country without cell service, requiring them to speak to the concierge to find transportation. Or, a customer at a bar may need to step outside to escape their noisy environment.
TomTom’s mapping services, coupled with a hardware device and software server, can make summoning a taxi easy for the venue and the customer. This boosts business for the taxi company. Picture a device with a screen and three simple buttons: one to summon a vehicle, one to cancel the call if it is no longer needed, and one for configuration.
What’s inside the device? It could be as simple as a Raspberry Pi 2 or 3. It only needs a small processor, memory to store its settings, a basic video (and optional audio) chip, and an Internet connection. A system on a chip can handle the design.
A venue’s staff or visitors would simply press a button to summon a taxi. This avoids the hassle of a phone call, the customer is happy to get a ride home, and the taxi service and drivers are happy to get the work.
In this tutorial, we review how to program a taxi summoning service using TomTom APIs and SDKs. You just need to know a little JavaScript to follow this tutorial — but TomTom libraries support other languages too.

Hardware in Motion
When using a taxi-summoning device like this, we press the call button. This call goes out to a preferred taxi service, which has a server running the device software. GPS devices equipped on taxis, or custom applications on mobile phones, report driver location to the server. The TomTom Location History API then catalogs this information and potentially also backs it up with an optional database.
This information includes an identifier assigned to that taxi and its current coordinates. If its driver’s information is included via the mobile application, the report can include that as well. These are stored as objects that the API can use to respond to additional queries. If the taxi reporting in is not yet in the location history, the code adds it:
curl -XPOST "Content-type: application/json" -d
'{
"name": "Taxi_000000",
"properties": {
"key": "value",
“taxi ID”: 0000-000-0000,
“driver”: Taxi Driver,
"latitude": 32.774403,
"longitude": -96.808632
}
}'
'https://baseURL/locationHistory/versionNumber/objects/object?key=Your_API_Key&adminKey=Your_Admin_Key
Note that API commands do this in your language of choice.
Otherwise, the code updates and tracks the taxi’s information. This can give the service a log of where their vehicles have been. We might also want to send additional information like vehicle speed to your app’s back-end — which can be easily obtained from a mobile device’s built-in GPS sensors. Or, we might want to let taxi drivers set their status, such as ‘Available’ or ‘On Break’ to ensure we don’t try to assign rides to drivers who are unavailable.
A taxi service often has an operation area, which can be defined by a geofence. In this case, it might be their whole city, for example. The application considers taxis anywhere inside that geofence, as they are likely to respond the quickest. It ignores any taxis outside the geofence.
Each taxi gets a request on their computer systems. Drivers accept or decline the work on a first-come-first-serve basis.
We assume here that at least one taxi will accept the request. After the driver confirms the destination and job, the system sends them a route to the business that requested the driver. If for some reason the business placed a call by mistake or no longer needs the taxi, the staff there can cancel the service using the cancel button.
If there is no cancellation, the taxi drives to the business and picks up the passengers!
Routing Control
Let’s look at how this works using TomTom’s API and the server.
The user (a hotel, bar, or other another venue) initially configures the device by selecting taxi services that have signed up. A touch screen helps the user set it up and enter payment information, in case they wished to comp the ride, as well as their address. A GPS chip inside the device could also take care of the location. The business owner then places the device where it is easy for their staff members to access.
To use the taxi-summoning device, a staff member, such as a bartender, a concierge, or front desk staff, simply presses the summon button. In this design, it is a large green call button. The call goes out to the taxi’s server.
The device generates a request ID including the business coordinates and address. Let us use a random address for this example.
{ “Request”: [{
“Business Name”: “North Bar”,
“Request ID”: “0000-0000-0000”,
“Coordinates”: “32.761196,-96.816742”,
“Address”: “1424 North Zang Boulevard, Dallas, TX 75203”
}]}
The code on the server uses the Location History API data to get the location of available taxis and send a request to TomTom’s Routing API, which determines the nearest taxi. It first requests the list of objects.
curl
‘https://baseURL/locationHistory/versionNumber/objects?key=Your_API_Key’
Then, the server iterates through the list and gets each taxi’s data.
curl 'https://baseURL/locationHistory/versionNumber/objects/objectId?key=Your_API_Key'
Each object includes the taxi’s last reported coordinates:
{
"key": "value",
“taxi ID”: 0000-000-0000,
“driver”: Taxi Driver,
"latitude": 32.774403,
"longitude": -96.808632
}
After it gets the list, the server then feeds the Routing API a new request using the matrix format. The matrix in this case consists of all the taxi locations and the destination (the business that made the request). A Matrix Routing API request looks somewhat like this when in JSON format, but it may be slightly different due to API updates:
curl ‘https://api.tomtom.com/routing/1/matrix/sync/json?key=<YOUR_API_KEY>&routeType=fastest&travelMode=car’
Request Body:
‘{
"origins": [
{
"point": {"latitude":32.778553,"longitude":-96.827011},
"point": {"latitude":32.746052-96.851463,"longitude":-96.851463},
"point": {"latitude":32.752831,"longitude": -96.765701}
}
],
"destinations": [
{"point": {"latitude": 32.761196,"longitude": -96.816742}}
]
}’
We have one certain destination and multiple GPS coordinates representing the currently available taxis. When the server submits this request, the Routing API calculates the fastest routes for each origin point to the destination. If it’s included, a customer destination can also be added to the calculations as another Point — this is Batch Routing.
Taxi En Route
At this point, the server uses TomTom’s request to match the taxi with the fastest time for the route. Then it puts in a request directly to the taxi identified. If the driver accepts the request, their application or device sends a confirmation to the server and taxi-summoning device.
Now that the driver is on their way, the server can alert the venue. If not, the request is still open, and it will go to the taxi with the next-fastest route. We’ll assume here the driver accepts the request.
As the taxi gets closer, the server can update the device with the taxi’s estimated time of arrival (ETA). The server gets this data from the taxi’s device or application and shows it on the business device’s screen. The employee who started the request can keep the customer updated and inform them when the taxi arrives.
Depending on the device design, it can also have some user experience (UX) features, such as a glowing light strip that lets users know when the taxi arrives or is close. Here’s how an administrator view of the app in action might look, showing all available taxis along with their projected arrival times:

You can set up your application so the customer or staff member can enter the destination when requesting a ride. Or, the customer can tell the driver when they arrive, and the driver can enter it into the app. However, the destination can be known long before even selecting the taxi, thanks to the matrix routing calculation which takes the entire route into consideration. The service then makes a new Routing API request and the taxi’s destination is updated on the server. The driver should receive the best route of the next segment, and take the passengers to their destination.
As an additional assurance, the hardware device can update the business that requested the taxi with the current ETA to the passenger’s destination. It helps guarantee that the passenger arrived safely.
In Conclusion
We have explored how a hardware device coupled with TomTom makes it easier for customers to summon a taxi and for taxi services to get a new customer. It is even easy for developers like us to incorporate TomTom APIs into an app with minimal development time.
You can use these same methods to summon taxis using a server and applications. However, using a hardware device reduces reliance on mobile devices and saves time. Either way, this project is a great way to make it easier for event venues and customers to find a taxi. Taxi companies can generate some more business or an enterprising developer like you can create a helpful new service for your city.
Check out our video tutorial below where developer advocate Olivia Vahsen goes over our article "Do Taxi Drivers Take the Fastest Route?". Getting driving data from a taxi driver in Porto, we recalculate the routes using the TomTom Maps APIs to check for deviations on the taken routes.
YouTube video playerLastly, you can explore our JavaScript taxi dispatcher tutorial below.
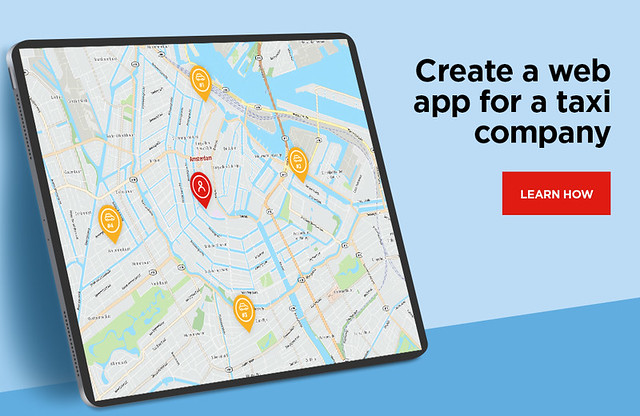
Ready to create this project or another mapping application? Register your TomTom developer account today to explore these and other APIs.
Happy mapping!