How to Migrate from Google Maps to TomTom Maps in 8 Easy Steps
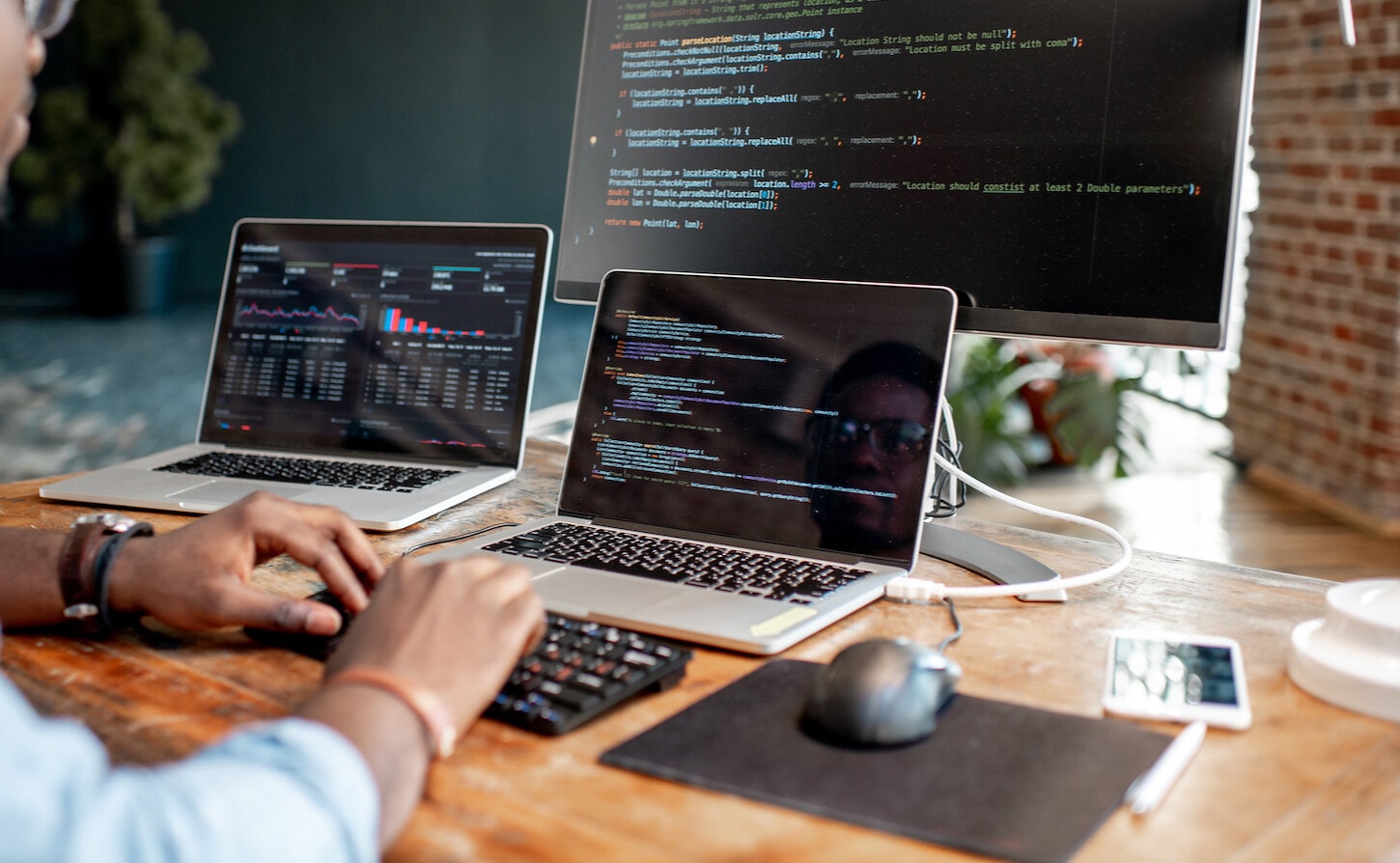
Using the TomTom SDK for Web as your new mapping solution has never been easier. Learn how to transition different elements of your map from Google Maps to TomTom Maps, from displaying a simple map to adding Traffic, Routing, Search and Map Markers in a matter of minutes.
Using the TomTom SDK for Web as your new mapping solution has never been easier. Here, I’ll go through not only how to transition different elements of your map to TomTom, but where the API differences lie. I’ll start with displaying a simple map, and go on to cover adding Traffic, Routing, Search and Map Markers in a matter of minutes.
Whether you’re looking to overhaul your location services for your website as a seasoned developer, or this is your first time interacting with Maps APIs, you should be able to set up your map and other features quickly and seamlessly.
Let’s get started!
But first…. A few things you’ll need.
The Maps library: The Maps SDK for Web can be used in three ways. In this tutorial, we are using a CDN as an example.
API Key - If you don't have an API key visit the How to get a TomTom API Key site and create one.
Npm and Node.Js installed on your machine to run the HTTP server.
1. Displaying a Map
Using the code below will call the API using version 6 of the TomTom SDK for Web. All you need to change is line 19, where you’ll add the API key you generated above.
<!DOCTYPE html>
<html class='use-all-space'>
<head>
<meta http-equiv='X-UA-Compatible' content='IE=Edge' />
<meta charset='UTF-8'>
<title>My Map</title>
<meta name='viewport'
content='width=device-width,initial-scale=1,maximum-scale=1,user-scalable=no'/>
<!-- Replace version in the URL with desired library version -->
<link rel='stylesheet' type='text/css' href='https://api.tomtom.com/maps-sdk-for-web/cdn/6.x/<version>/maps/maps.css'/>
</head>
<body>
<div id='map' class='map' style='width:100%; height:100vh'></div>
<!-- Replace version in the URL with desired library version -->
<script src='https://api.tomtom.com/maps-sdk-for-web/cdn/6.x/<version>/maps/maps-web.min.js'></script>
<script>
tt.setProductInfo('<your-product-name>', '<your-product-version>');
tt.map({
key: '<your-tomtom-API-Key>',
container: 'map'
});
</script>
</body>
</html>
2. Run an HTTP Server
For our map to render on a website, you need to run an HTTP server. This requires npm and Node.Js.
To set up the server, run the following commands in your terminal:
~$ cd sdk-tutorial/~sdk-tutorial$
Install a light-weight HTTP server (you might need to run this command with admin/root privileges):
npm install --global http-server
Then, run this command to run the server.
http-server
Note that npm comes bundled with Node.js, so please make sure you have it installed on your machine.
http://localhost:8080/index.html. You should be able to see a map! If not, use developer mode (press F12 on Windows or Linux or ⌘ + ⌥ + I on Mac) to check for console errors.
When you’re done, heading to the following address in your web browser’s should result in seeing your new map!
3. Initializing a Map
Below are the basic instantiations of the map object for both Google and TomTom for you to examine. You can see the differences in essential setup, notably where the TomTom Maps SDK for Web requires a ‘key’ property for your API key. You receive your API key for free after registering at developer.tomtom.com, and it allows for up to 2500 free API transactions per day.
Googlevar map = new google.maps.Map(document.getElementById('map'), {
center: { lat: 37.769167, lng: -122.478468},
zoom: 15
});
TomTomvar map = tt.map({
key: '<your-tomtom-API-key>',
container: 'map',
center: [-122.478468, 37.769167],
zoom: 15
});
Both create a variable to refer to later.
Both use ‘center’ and ‘zoom’ as properties.
Both require the ID of an element to render the map (in this example, 'map').
In addition, the TomTom Maps SDK for Web expresses coordinates as an array rather than an object with properties.
Within tt.map, paste your key, set up the container, pick your center coordinates, and your default zoom. When the map initially loads on your websites, it will display at the location you specified in lat-long, and will load at the given zoom.
4. Displaying Traffic
Next, you can add a traffic layer to your new TomTom Map.
This is how you might be familiar with adding traffic via the Google Maps API:
var trafficLayer = new google.maps.TrafficLayer();
trafficLayer.setMap(map);
TomTomThere are two services that offer traffic information:
Adding Traffic Flow adds a visual layer showing the difference between current and free flow speed.
Green indicates that the speeds are the same, with no traffic jams.
Red indicates that traffic is in a slowdown period, where there are more traffic jams.
The Traffic Incidents layer indicates traffic disturbances at specific points, such as closed roads, rain, ice, or individual accidents, as opposed to broader slow moving traffic stretches which would be visualized with Traffic Flow.
The code below helps you display both types of traffic data in Javascript. For the Typescript option, head over here.
map.on('load', function() {
map.showTrafficFlow();
map.showTrafficIncidents();
});
The following sets traffic visibility when instantiating the map object.
var map = tt.map({
key: '${api.key.maps}',
container: 'map',
stylesVisibility: {
trafficIncidents: true,
trafficFlow: true
}
});
5. Displaying a Marker
Adding markers to your map only takes two lines of code, which you’ll see is a little shorter than what you may be used to with Google.
var marker = new google.maps.Marker({
position: { lat: 37.768454, lng: -122.492544 },
map: map
});
First, add a var marker at a specific point:
var marker = new tt.Marker().setLngLat([-122.492544, 37.768454]).addTo(map);
Now that we already have the marker itself, let’s add a popup, which will display when the user clicks the marker. You can add extra information in this popup, say, details about a specific place, for your user to interact with.
This just takes one extra line of code:
marker.setPopup(new tt.Popup().setHTML('my marker'));
Google’s version:
var infowindow = new google.maps.InfoWindow({
content: 'my marker'
});
marker.addListener('click', function() {
infowindow.open(map, marker);
});
6. Displaying a Route/Directions
We’ll work with the Routing API a little bit in this section to display a route from point A to point B on your map. Google’s name for this service is “directions”.
This is how you would formerly access Google’s directions, utilizing two services: one to ask for route from A to B, and one to render the route itself on the map.
var directionsService = new google.maps.DirectionsService();
var directionsDisplay = new google.maps.DirectionsRenderer();
directionsDisplay.setMap(map);
var request = {
origin: new google.maps.LatLng(37.768014, -122.510601),
destination: new google.maps.LatLng(37.769167,-122.478468),
travelMode: 'DRIVING'
};
directionsService.route(request, function(response, status) {
if (status == 'OK') {
directionsDisplay.setDirections(response);
}
});
TomTomFirst, add the services library in your HTML.
<!-- Replace version in the URL with desired library version -->
<script src='https://api.tomtom.com/maps-sdk-for-web/cdn/6.x/<version>/services/services-web.min.js'></script>
Then, copy the code below:
tt.services.calculateRoute({
key: 'YOUR_API_KEY',
locations: '-122.510601,37.768014:-122.478468,37.769167'
})
.then(function(response) {
var geojson = response.toGeoJson();
map.addLayer({
'id': 'route',
'type': 'line',
'source': {
'type': 'geojson',
'data': geojson
},
'paint': {
'line-color': '#00d7ff',
'line-width': 8
}
});
var bounds = new tt.LngLatBounds();
geojson.features[0].geometry.coordinates.forEach(function(point) {
bounds.extend(tt.LngLat.convert(point));
});
map.fitBounds(bounds, {padding: 20});
});
The only required parameters for the routing call are the start and end points, separated by a colon. If more colon-separated coordinates are provided, the ones in the middle will be used as intermediate locations (waypoints). By default, the route will be calculated for a car, but this and many other parameters can be changed. See the Maps SDK For Web Documentation - Calculate Route for complete details of routing parameters and filters.
Call the routing service by executing the tt.services.calculateRoute() function. The result will be returned as GeoJSON containing a 'LineString' feature. This is a (poly)line that can be added to a map, with defined properties like color, opacity, and weight.
Then call 'fitBounds' to center the map on the route.
On displaying the route:The code above generates a polyline, but no markers. Developers have a lot of freedom to display the route, but if a start and end marker are required, they can be added in the same way as the "pop-up marker" above, shown again below:
new tt.Marker().setLngLat([-122.478468,37.769167]).addTo(map);
new tt.Marker().setLngLat([-122.510601,37.768014]).addTo(map);
You can find more information about markers, including how to customize them, in the Maps SDK for Web documentation.
7. Using Search
You can query the Fuzzy Search API and place a marker at the point indicated by the search result automatically. This isn’t the same as including a search bar in your map, which you can add from this guide, but it’s a brief primer on accessing fuzzy search to get you started.
Thankfully, searching for places is very similar in both SDKs. We need to provide a query, and then work on the Response provided by the service.
Googlevar request = {
query: 'Golden Gate Golf Course San Francisco',
fields: ['name', 'geometry'],
};
service = new google.maps.places.PlacesService(map);
service.findPlaceFromQuery(request, function(results, status) {
if (status === google.maps.places.PlacesServiceStatus.OK) {
for (var i = 0; i < results.length; i++) {
new google.maps.Marker({
map: map,
position: results[i].geometry.location
});
}
}
});
TomTomtt.services.fuzzySearch({
key: 'YOUR_API_KEY',
query: 'Golden Gate Golf Course San Francisco'
})
.then(function(response) {
for (var i = 0; i < response.results.length; i++) {
new tt.Marker().setLngLat(response.results[i].position).addTo(map);
}
});
8. Happy Mapping!
If you followed all the steps above, you’ve worked with a good number of the TomTom Maps APIs already and might be ready to move on to more mapping components for your project.
For your website, check out: Setting up WordPress Plugins, and Adding a Map to your Website for CMS Users.
If you've like to check out a video version of this tutorial, check this out:
YouTube video playerIf you have more questions, find us on our forum!
Happy mapping!